Immutability vs. Thread Safety: Clarifying the Confusion
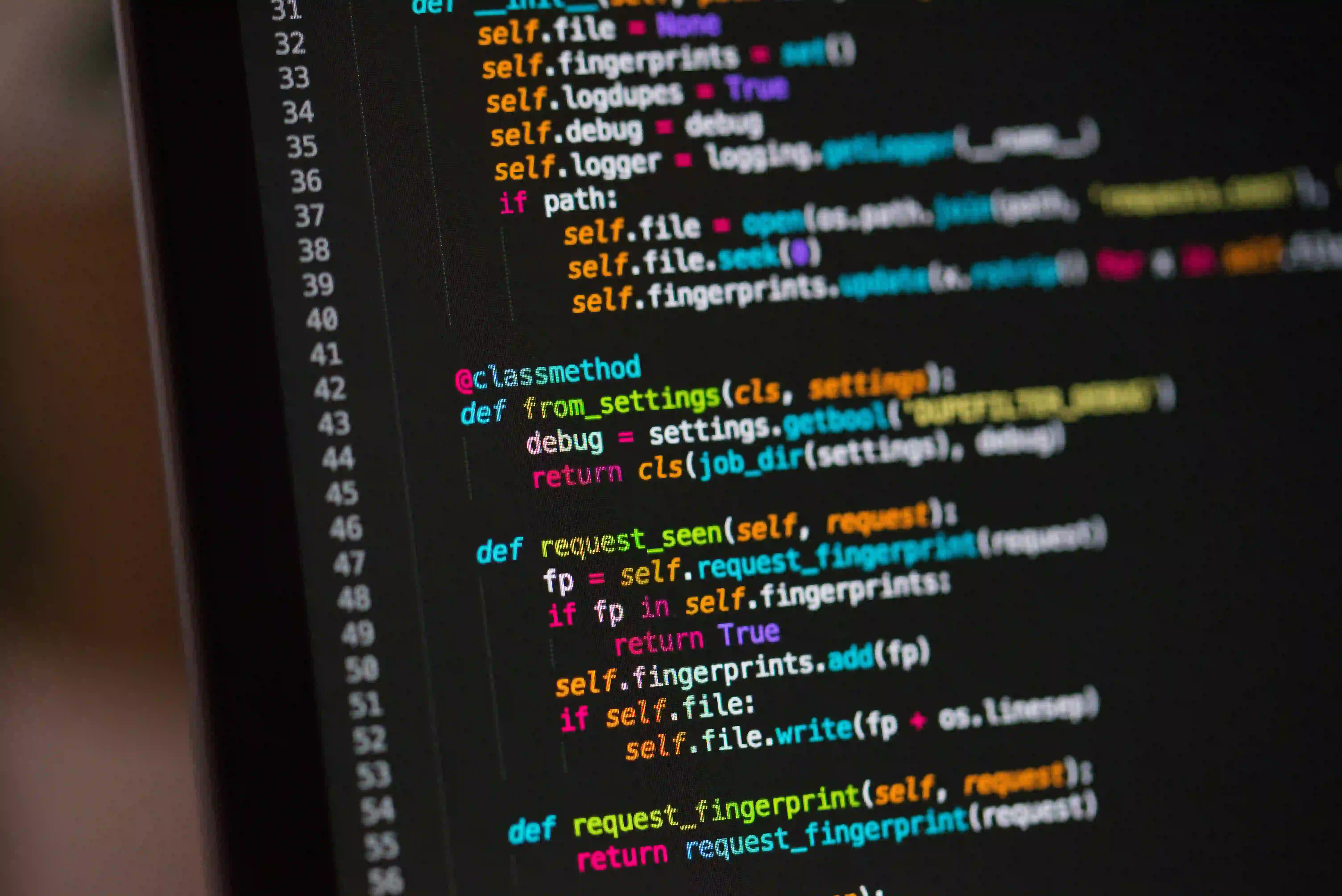
Immutability vs. Thread Safety: Clarifying the Confusion
In the realm of Java programming, two concepts often emerge that can lead to confusion for both beginner and experienced developers: immutability and thread safety. While related, they are distinct concepts, and understanding their differences is crucial for writing clean, efficient, and robust Java applications.
What is Immutability?
Immutability is a property of an object that, once created, cannot be modified. In Java, this is typically achieved by making the class final and ensuring that fields are final as well. The most notable example of an immutable class in Java is String
.
Benefits of Immutability
- Simplicity: Since immutable objects cannot change, you can avoid unexpected side effects and behaviors.
- Ease of concurrency: Immutable objects can be shared across threads without synchronization, which is usually a source of bugs in concurrent processing.
- Caching and Performance: Immutable objects can be cached and reused without concerns of altering their state.
Example of an Immutable Class in Java
Here’s a simple implementation of an immutable class:
public final class ImmutablePoint {
private final int x;
private final int y;
public ImmutablePoint(int x, int y) {
this.x = x;
this.y = y;
}
public int getX() {
return x;
}
public int getY() {
return y;
}
// No setters allowed, which makes this class immutable.
}
In the above code:
- The class
ImmutablePoint
is declared asfinal
to prevent subclassing. - The fields
x
andy
are also declared asfinal
, ensuring they can only be set once. - There are no setter methods, which further enforces the immutability.
Remember, immutability simplifies debugging, but it also can lead to increased memory usage in some cases, as a new object is created for every modification.
What is Thread Safety?
Thread safety, on the other hand, refers to the concept where shared data is accessed by multiple threads without causing any race conditions. In a thread-safe class, methods are designed to work correctly when accessed from multiple threads simultaneously.
Key Characteristics of Thread Safe Classes
- Synchronization: Using synchronized methods or blocks to control access to shared resources.
- Atomicity: Ensuring that operations on shared data are completed without interruption.
- Locking: Employing locks to manage resource access among threads, which may involve considerable performance overhead.
Example of a Thread-Safe Class
Here’s a simple example of a thread-safe counter using synchronization:
public class ThreadSafeCounter {
private int count = 0;
public synchronized void increment() {
count++;
}
public synchronized int getCount() {
return count;
}
}
In this example:
- The
increment
andgetCount
methods are marked assynchronized
, ensuring that only one thread can execute these methods at a time on the same object.
However, synchronous methods can come with performance costs, especially in high-concurrency applications.
Drawing the Line: Immutability vs. Thread Safety
These two concepts intertwine, but confusion arises when trying to apply them in various scenarios.
-
Immutability provides inherent thread safety: Since immutable objects cannot change state, they do not need synchronization when accessed by multiple threads. Thus, all instances of immutable objects can be shared freely among threads.
-
Thread safety does not imply immutability: A thread-safe class can still have mutable state, and its behavior can change during execution if not handled correctly. Thread-safe classes can still end up in inconsistent states if atomicity is not guaranteed.
Example of a Mutable Thread-Safe Class
Consider the following mutable thread-safe queue implementation:
import java.util.LinkedList;
public class ThreadSafeQueue {
private final LinkedList<String> queue = new LinkedList<>();
public synchronized void enqueue(String item) {
queue.addLast(item);
}
public synchronized String dequeue() {
return queue.isEmpty() ? null : queue.removeFirst();
}
}
In this example:
- The
ThreadSafeQueue
class is thread-safe due to synchronized access, but it is not immutable. It accepts modifications via its enqueue and dequeue methods.
Common Use Cases
When to Use Immutability
- When you need a simple object that is safe to share between threads, like configuration objects or data structure keys.
- When designing classes to represent values rather than states, such as coordinates, dates, or even data transfer objects.
When to Use Thread Safety
- When your application needs to maintain shared mutable state, such as in cases dealing with user sessions, banking transactions, or resource management mechanisms.
For more detailed insights on thread-safety and best practices, consider reading Oracle's Java Concurrency Tutorial.
In Conclusion
Understanding both immutability and thread safety is essential for a Java developer. While immutability offers a straightforward way to ensure thread safety, it’s crucial to note that thread safety can also apply to mutable objects under certain conditions and practices.
Immutability simplifies concurrency but may lead to complexity in memory management. Conversely, thread safety requires careful design considerations and may introduce performance trade-offs.
Embracing both principles will elevate your Java skills, helping you write cleaner, more effective, and maintainable code. As you tackle various challenges within your Java projects, keep these concepts in mind to navigate the intricacies of concurrent programming confidently.
For additional reading on the topic, check out Effective Java by Joshua Bloch, which provides in-depth explanations and best practices.
Happy coding!