Troubleshooting JNDI Connection Pool Issues in Spring Tests
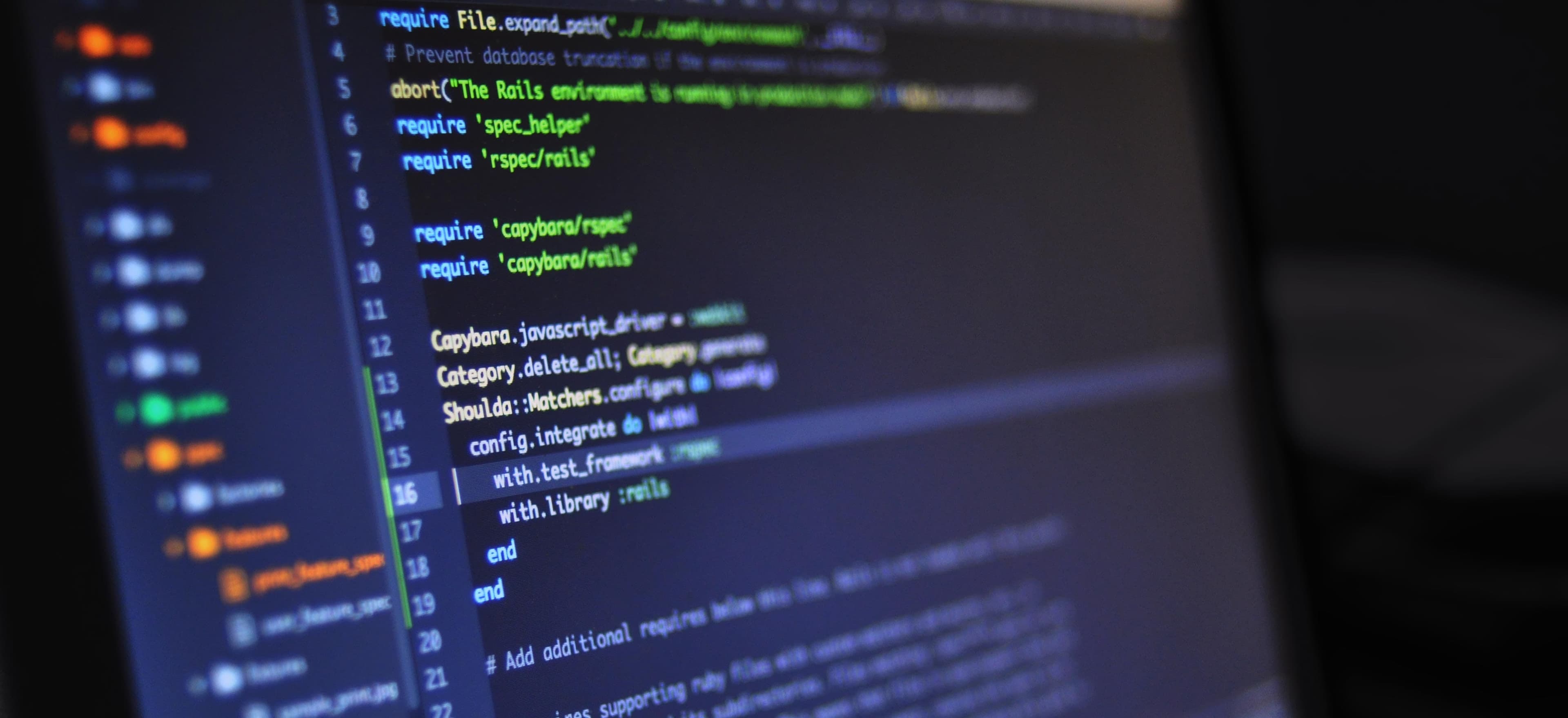
- Published on
Troubleshooting JNDI Connection Pool Issues in Spring Tests
Spring is a powerful framework Java developers trust for building robust applications. One of the most critical components of a Java application is the database connectivity, often managed via Java Naming and Directory Interface (JNDI). JNDI allows Java applications to discover and look up data and resources such as connection pools. In this blog post, we'll explore how to troubleshoot common JNDI connection pool issues, especially in the context of Spring testing.
Understanding JNDI in Spring
JNDI provides an application-aware mechanism to obtain database connections from a pool rather than opening a new connection each time one is needed. This improves performance and resource management. In a Spring application, JNDI is used alongside a connection pool, such as Apache DBCP or HikariCP, to configure data sources.
Here’s an example of how a JNDI data source can be configured in a Spring application.
<bean id="dataSource" class="org.apache.commons.dbcp.BasicDataSource" destroy-method="close">
<property name="lookupOnCreate" value="true" />
<property name="jndiName" value="java:comp/env/jdbc/MyDataSource" />
</bean>
In the example above, we’re defining a BasicDataSource
bean using a JNDI lookup to fetch the actual database connections.
Common Connection Pool Issues
Before diving into troubleshooting techniques, let’s identify problems you might encounter with JNDI connection pools in Spring tests:
- No Initial Context: You're not able to find the JNDI resources.
- Resource Not Bound: The expected resource is not available.
- Connection Pool Exhaustion: The limits of connections are reached.
- Configuration Issues: Problems with resource properties, such as the database URL, username, or password.
- Timing Issues: Test configurations might not be set up before the methods which need them are executed.
Troubleshooting Steps
Let’s take a closer look at how to troubleshoot these issues effectively.
1. Validate JNDI Resource Availability
The first step in troubleshooting is to ensure that the JNDI resource is indeed available. You can perform this by writing a minimal standalone Java application that attempts to perform a JNDI lookup.
import javax.naming.Context;
import javax.naming.InitialContext;
import javax.naming.NamingException;
public class JndiLookupTest {
public static void main(String[] args) {
try {
Context ctx = new InitialContext();
Object obj = ctx.lookup("java:comp/env/jdbc/MyDataSource");
System.out.println("Successfully looked up JNDI resource: " + obj);
} catch (NamingException e) {
System.err.println("JNDI lookup failed: " + e.getMessage());
}
}
}
Why: By using a standalone application, you can isolate the problem and confirm that your JNDI resource is indeed configured correctly.
2. Check Your Application Server Configuration
If the lookup fails, check the configuration of your application server (such as Tomcat, WildFly, or Jetty). Ensure that:
- The JDBC driver is correctly included.
- The JNDI resource is defined in the server's configuration file.
- The context path is set correctly.
Here’s an example of how you can define a JNDI resource in a Tomcat context.xml
:
<Resource name="jdbc/MyDataSource" auth="Container"
type="javax.sql.DataSource" maxTotal="20" maxIdle="10"
maxWaitMillis="-1" username="dbUser" password="dbPass"
driverClassName="com.mysql.cj.jdbc.Driver"
url="jdbc:mysql://localhost:3306/mydatabase"/>
Why: This ensures that your Spring application can correctly refer back to the JNDI resource when it’s deployed in the server.
3. Increasing Connection Pool Limits
If you are experiencing connection pool exhaustion, you need to review and possibly increase the limit of your connection pool. For instance, using HikariCP, you can set properties like:
spring:
datasource:
hikari:
maximum-pool-size: 30
Why: By adjusting these parameters, you ensure that your application can handle spikes in traffic without running into connection availability issues.
4. Ensure Proper Cleanup
Make sure that connections from the pool are correctly closed after use. Failing to close connections may lead to pool exhaustion, as demonstrated in the following example:
try (Connection connection = dataSource.getConnection()) {
// Use the connection
} catch (SQLException e) {
e.printStackTrace();
}
// Connection will automatically be closed here
Why: By employing the try-with-resources statement, you're guaranteeing that each connection is closed, thus avoiding leaks which can exhaust the pool.
5. Set Up Testing Context Properly
In Spring tests, the context setup is crucial. Ensure you annotate your tests with the appropriate Spring annotations. One common issue is that the context is not loaded properly, which can lead to JNDI resources not being available.
Here’s an example of setting up your test class correctly:
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(locations = "classpath:application-test-context.xml")
public class MyServiceTest {
@Autowired
private MyService myService;
@Test
public void testServiceMethod() {
// Test logic
}
}
Why: Correct setup ensures that JNDI resources are available to your tests through Dependency Injection.
6. Monitor Connection Usage
If you continue to see connection pool issues, consider using monitoring tools that can profile the pool’s usage. Libraries like HikariCP include a built-in connection pool metrics facility.
HikariDataSource dataSource = (HikariDataSource) context.getBean("dataSource");
System.out.println("Active Connections: " + dataSource.getHikariPoolMXBean().getActiveConnections());
Why: Monitoring active connections gives valuable insights into whether your pool configuration is adequate for your application’s usage patterns.
Closing Remarks
Troubleshooting JNDI connection pool issues in Spring applications requires a methodical approach. By verifying resource availability, checking server configurations, ensuring proper cleanup, and leveraging Spring's testing capabilities, you can effectively resolve issues that might slow down or hinder your application.
Additional Resources
- Spring Documentation on DataSource
- JNDI Overview
- HikariCP Documentation
By following these guidelines and leveraging the provided code examples, you can enhance your troubleshooting skills and ensure your Spring applications maintain robust database connectivity. Happy coding!