Common Pitfalls When Using Embedded Tomcat with Maven
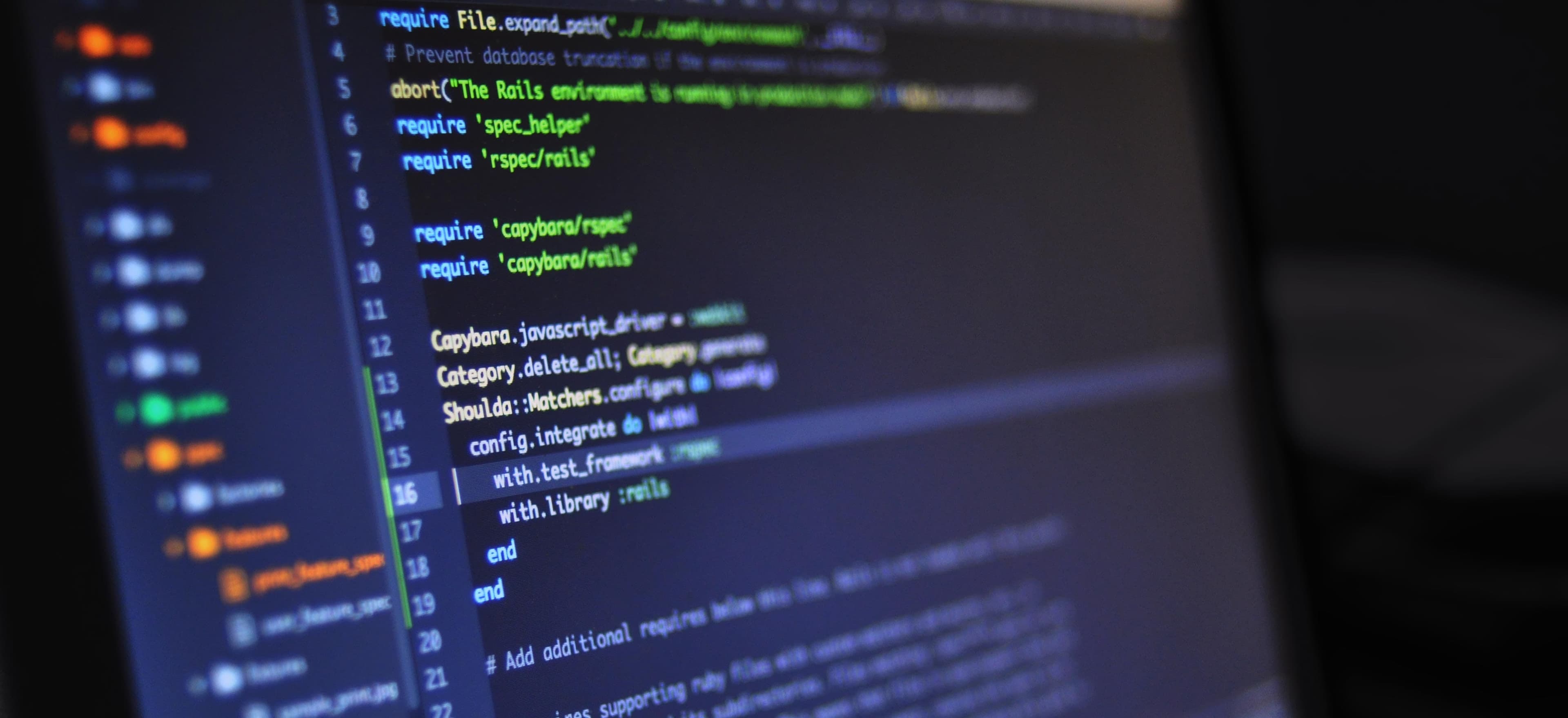
- Published on
Common Pitfalls When Using Embedded Tomcat with Maven
In today’s rapidly evolving software landscape, the incorporation of lightweight, embeddable tools has become essential. One such tool is Embedded Tomcat—an adept solution for developers who need a streamlined way to run web applications in a Java environment. Leveraging Maven for dependency management and project setup further simplifies the build process for Java applications. However, it’s easy to trip over certain common pitfalls that can hinder productivity and lead to perplexing issues.
In this post, we will explore these pitfalls, illustrating how to avoid them, all while leveraging Maven effectively with Embedded Tomcat.
Overview of Embedded Tomcat
Embedded Tomcat allows developers to embed a Tomcat server directly within their Java applications. You get the benefits of standard Tomcat configurations, such as servlets, JSPs, filters, and session management, while avoiding the hassle of finding a suitable Tomcat installation.
When paired with Maven, which excels at dependency management and project automation, the combination becomes powerful for rapid development and testing.
Common Pitfalls
1. Ignoring Dependency Management
One of the most significant advantages of using Maven is its robust dependency management. However, failing to include the necessary dependencies for Embedded Tomcat can lead to a world of hurt.
Example Dependency Configuration
<dependencies>
<dependency>
<groupId>org.apache.tomcat.embed</groupId>
<artifactId>tomcat-embed-core</artifactId>
<version>9.0.50</version>
</dependency>
<dependency>
<groupId>org.apache.tomcat.embed</groupId>
<artifactId>tomcat-embed-websocket</artifactId>
<version>9.0.50</version>
</dependency>
<dependency>
<groupId>org.apache.tomcat.embed</groupId>
<artifactId>tomcat-embed-jasper</artifactId>
<version>9.0.50</version>
</dependency>
</dependencies>
Why This Matters
Adding the right dependencies helps the Tomcat server function with all the necessary features, such as web socket support or JSP rendering. Missing out on essential dependencies could lead to runtime exceptions that can be tricky to debug.
Additional Resource
For a comprehensive look at the different Maven dependencies you can utilize, visit Maven Central Repository.
2. Misconfiguring the Server Port
By default, Embedded Tomcat runs on port 8080. However, if there's a conflict with another service, (say your local database), you will need to adjust the server port.
Changing the Port Example
import org.apache.catalina.startup.Tomcat;
public class EmbeddedTomcat {
public static void main(String[] args) throws Exception {
Tomcat tomcat = new Tomcat();
tomcat.setPort(8081); // Set to a different port
tomcat.addWebapp("", "src/main/webapp");
tomcat.start();
tomcat.getServer().await();
}
}
Why This Matters
Failing to configure the correct port can cause the server to fail to start, leading to wasted time hunting for errors. Always check what services are running on your machine to prevent such issues.
3. Not Cleaning Up Resources
When running a web application using Embedded Tomcat, developers may forget to properly shut down the server and free up resources.
Proper Cleanup Example
public class EmbeddedTomcat {
public static void main(String[] args) throws Exception {
Tomcat tomcat = new Tomcat();
tomcat.setPort(8080);
tomcat.addWebapp("", "src/main/webapp");
Runtime.getRuntime().addShutdownHook(new Thread(tomcat::stop));
tomcat.start();
tomcat.getServer().await();
}
}
Why This Matters
The use of a shutdown hook ensures that resources are freed upon termination of the JVM process, saving issues related to unclosed database connections or lingering threads.
4. Overlooking Logging
Embedded Tomcat supports various logging strategies. Relying solely on console logs can obscure valuable server diagnostics.
Configuring Logging Example
You may opt to use java.util.logging
to enhance your visibility.
import org.apache.juli.logging.Log;
import org.apache.juli.logging.LogFactory;
public class EmbeddedTomcat {
private static final Log log = LogFactory.getLog(EmbeddedTomcat.class);
public static void main(String[] args) throws Exception {
Tomcat tomcat = new Tomcat();
try {
tomcat.setPort(8080);
tomcat.addWebapp("", "src/main/webapp");
tomcat.start();
log.info("Embedded Tomcat started.");
tomcat.getServer().await();
} catch (Exception e) {
log.error("Tomcat failed to start.", e);
}
}
}
Why This Matters
Incorporating structured logging enables you to capture critical runtime information that can aid with debugging and enhancing overall application performance.
5. Failing to Enable Hot Swap
During development, it’s common to change code frequently. Hot swapping allows changes to be reflected without restarting the server.
Maven Plugin Configuration
To enable hot swapping, you should utilize the Maven Cargo Plugin or incorporate tools such as JRebel. Below is a configuration snippet using Cargo:
<build>
<plugins>
<plugin>
<groupId>org.codehaus.mojo</groupId>
<artifactId>cargo-maven2-plugin</artifactId>
<version>1.9.6</version>
<configuration>
<container>
<containerType>embedded</containerType>
</container>
</configuration>
</plugin>
</plugins>
</build>
Why This Matters
Hot swapping saves time during development, allowing you to focus on writing code instead of constantly restarting the server. Not leveraging this feature is an understandable pitfall that can slow down your development process.
Bringing It All Together
While Embedded Tomcat offers immense flexibility, it does come with its own set of pitfalls when combined with Maven. By understanding these challenges—like dependency management, server configuration, proper resource cleanup, logging, and enabling hot swapping—you can improve your development efficiency and build applications that are robust and performant.
Always ensure you are maintaining the best practices to maximize the effectiveness of your tools. For further reading on optimizing your Tomcat and Maven experience, check Apache Tomcat Documentation.
Mastering Embedded Tomcat with Maven will significantly enhance your development workflow, leading to better-built applications and fewer headaches along the way. Happy coding!
Checkout our other articles