Mastering POST Requests in Apache Camel: Common Pitfalls
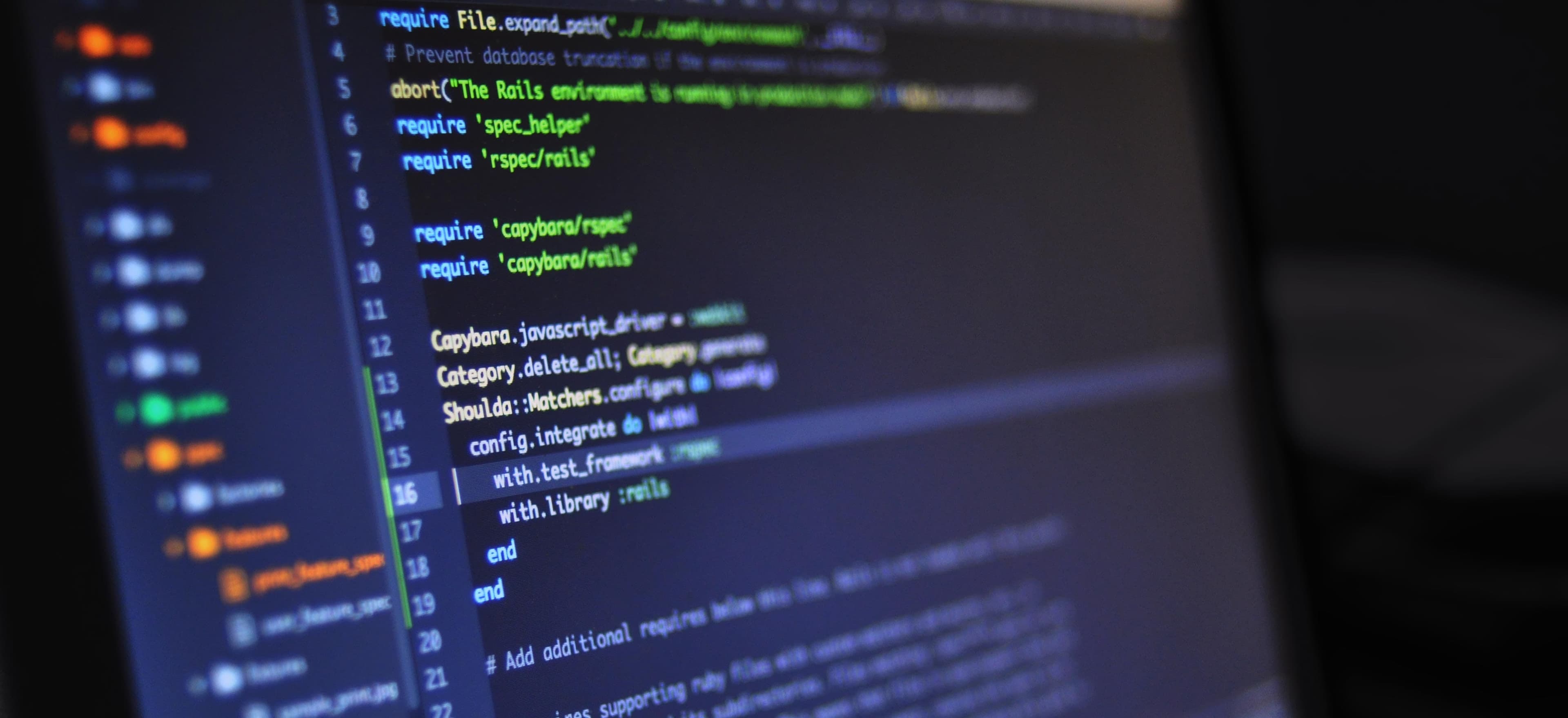
- Published on
Mastering POST Requests in Apache Camel: Common Pitfalls
Apache Camel is an integration framework that allows developers to create powerful routing and mediation rules in a range of applications. One of the most common operations performed in Camel is sending HTTP POST requests. While it might seem straightforward, developers often face pitfalls that can lead to unexpected behaviors or failures. In this blog post, we will explore some of these common pitfalls and how to avoid them while mastering POST requests in Apache Camel.
Why Use Apache Camel for POST Requests?
Apache Camel provides a unified API for handling various transport protocols including HTTP, JMS, and FTP. The lightweight architecture allows for easy integration with different systems and can streamline the process of sending POST requests. But just as useful, it abstracts much of the complexity involved in making these calls, making it essential for robust API communications.
Let’s delve into some of the common pitfalls developers encounter when using POST requests in Apache Camel.
1. Understanding the Basics of a POST Request
Before diving into the pitfalls, it's crucial to comprehend what a POST request is and why it’s important. A POST request is used when you need to send data to a server for processing. This could be in the form of creating a new resource in a RESTful API.
Example Code Snippet
Using the camel-http
component to perform POST request:
from("direct:start")
.to("http://example.com/api/resource?bridgeEndpoint=true&httpMethod=POST")
.process(exchange -> {
String response = exchange.getIn().getBody(String.class);
System.out.println("Response: " + response);
});
Why this works: In this example, direct:start
serves as the starting point for your Camel route. The to
method specifies the target endpoint, where we pass httpMethod=POST
as a parameter. The bridgeEndpoint=true
allows for a straightforward HTTP communication without Camel introducing its own headers or additional parameters.
2. Handling Content-Type Properly
One of the prevalent mistakes is not correctly setting the Content-Type
header. This header is crucial because it tells the server how to interpret the data being sent. A common scenario is not correctly defining it when sending JSON
.
Solution
Make sure to set the Content-Type
header when sending a POST request:
from("direct:start")
.setHeader(Exchange.HTTP_METHOD, constant("POST"))
.setHeader(Exchange.CONTENT_TYPE, constant("application/json"))
.setBody(constant("{\"key\":\"value\"}"))
.to("http://example.com/api/resource?bridgeEndpoint=true")
.process(exchange -> {
String response = exchange.getIn().getBody(String.class);
System.out.println("Response: " + response);
});
Why this matters: If the wrong Content-Type
is set, the server may interpret the data incorrectly, leading to errors. Using application/json
when sending JSON data is essential for successful communication.
3. Forgetting to Include Error Handling
Another common pitfall is neglecting error handling during POST requests. In a production environment, not having a means to gracefully manage failures can lead to potential data loss or corrupt states.
Best Practices
Utilize Camel’s built-in error handling features to manage exceptions effectively:
from("direct:start")
.errorHandler(deadLetterChannel("log:error")
.maximumRedeliveries(3).redeliveryDelay(2000))
.setHeader(Exchange.HTTP_METHOD, constant("POST"))
.setBody(constant("{\"key\":\"value\"}"))
.to("http://example.com/api/resource")
.process(exchange -> {
String response = exchange.getIn().getBody(String.class);
System.out.println("Response: " + response);
});
Why it's crucial: The deadLetterChannel
allows you to log errors and retry the connection a specified number of times. This is invaluable for detecting transient errors, ensuring that they don't halt your application.
4. Sending Large Payloads
When sending large payloads in a POST request, developers can encounter performance issues or even timeouts. It's important to manage larger data in chunks or streaming mode.
Solution: Streaming
Use streaming to handle large payloads:
from("direct:start")
.setHeader(Exchange.HTTP_METHOD, constant("POST"))
.setBody(simple("{{your.large.data}}"))
.to("http://example.com/api/resource?bridgeEndpoint=true&transferEncodingChunked=true")
.process(exchange -> {
String response = exchange.getIn().getBody(String.class);
System.out.println("Response: " + response);
});
Why streaming matters: Setting transferEncodingChunked=true
enables the request to send large payloads in smaller chunks, preventing memory overflow issues.
5. Ignoring Response Codes
Many developers overlook checking HTTP response codes. Ignoring this can result in treating unsuccessful responses as successes.
Solution: Implement Response Validation
Check for the response code effectively:
from("direct:start")
.setHeader(Exchange.HTTP_METHOD, constant("POST"))
.setBody(constant("{\"key\":\"value\"}"))
.to("http://example.com/api/resource")
.process(exchange -> {
int responseCode = exchange.getIn().getHeader(Exchange.HTTP_RESPONSE_CODE, Integer.class);
if (responseCode >= 200 && responseCode < 300) {
String response = exchange.getIn().getBody(String.class);
System.out.println("Success: " + response);
} else {
throw new RuntimeException("Failed: HTTP error code: " + responseCode);
}
});
Why checking response codes is essential: Validating the response code allows you to ensure that your application behaves appropriately based on the server's response. This is critical for error handling and debugging.
6. Not Utilizing Camel’s Logging Capabilities
Underestimating the value of logging insights can lead to frustrating debugging sessions. Camel’s logging features allow for robust monitoring.
Enable Logging
In your route, use Camel’s logging:
from("direct:start")
.log("Requesting ${header.CamelHttpUri}")
.to("http://example.com/api/resource")
.log("Response: ${body}");
Why logging is beneficial: This simple yet powerful addition aids in monitoring the routes and data flow, crucial for troubleshooting any issues that arise during the POST request process.
Key Takeaways
POST requests in Apache Camel can streamline your application's interactions with external systems. However, to truly master this functionality, one must be aware of the common pitfalls. From setting headers correctly to managing error handling and payload sizes, each aspect plays a pivotal role in ensuring successful communication.
By understanding and addressing these issues, you can create effective and reliable integration solutions using Apache Camel. For further reading on best practices with Apache Camel, consider checking out Apache Camel Documentation and RESTful API Design. With practice, your proficiency in using POST requests within Apache Camel will flourish, enhancing your development endeavors. Happy coding!
Checkout our other articles