Common Pitfalls in Securing REST APIs with Spring
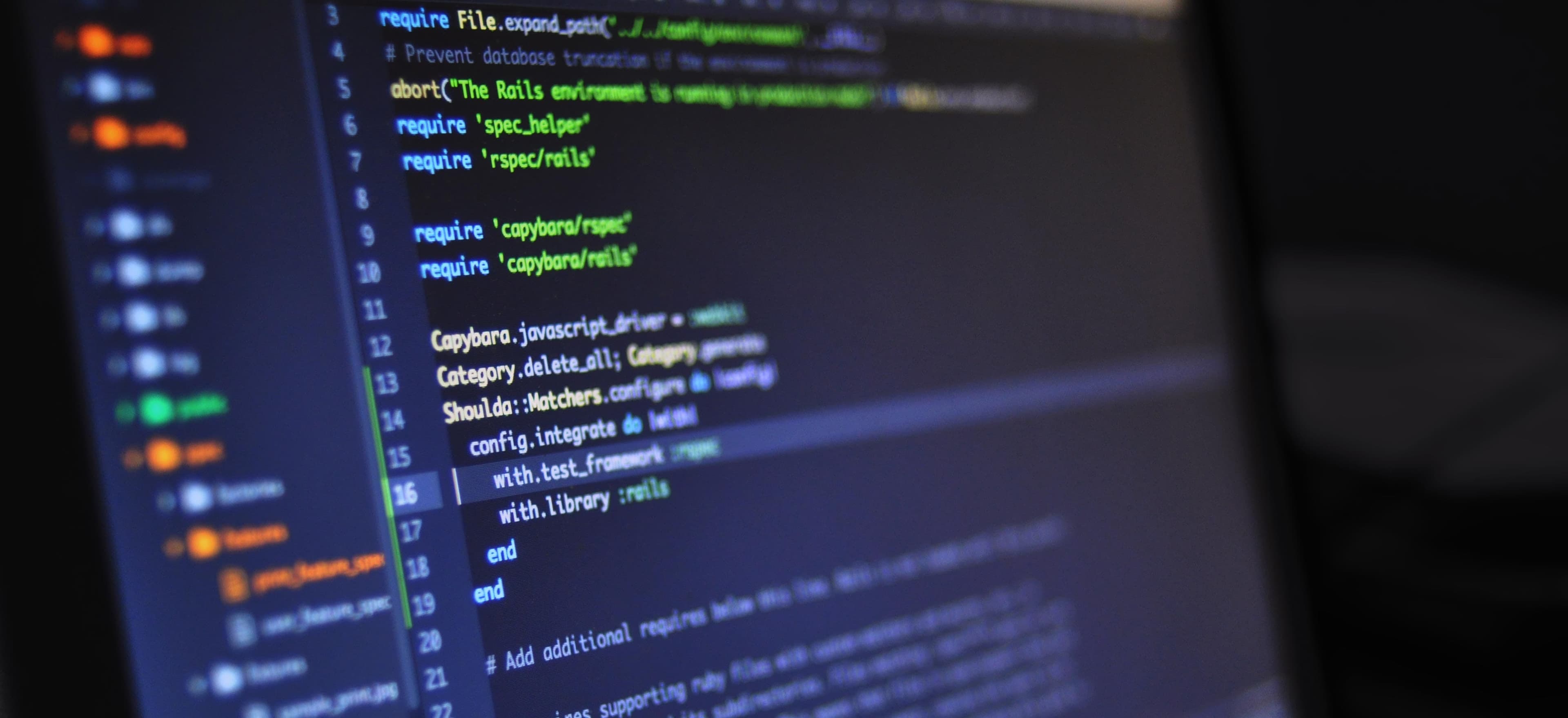
- Published on
Common Pitfalls in Securing REST APIs with Spring
In today's digital landscape, building a secure REST API is paramount. With the proliferation of cyber threats, developers must ensure that their APIs are resilient against various attacks. Spring, a leading framework in Java development, provides robust tools for creating RESTful services. However, when it comes to securing these services, developers often encounter several pitfalls. In this blog post, we will explore these common mistakes and how to effectively avoid them.
Understanding the Basics
Before diving into the pitfalls, let's briefly discuss what makes APIs susceptible to security risks. According to the OWASP Top Ten, APIs can be vulnerable to risks such as injection attacks, broken authentication, sensitive data exposure, and excessive data exposure. Understanding these vulnerabilities is the first step in implementing robust security measures.
1. Inadequate Authentication Mechanisms
The first pitfall is using weak or insufficient authentication methods for your REST API. It's crucial to ensure that only authorized users have access to sensitive endpoints.
Example of Weak Authentication
@RestController
@RequestMapping("/api")
public class MyController {
@GetMapping("/data")
public ResponseEntity<String> getData() {
return ResponseEntity.ok("Sensitive Data");
}
}
In this example, anyone can access the /data
endpoint without authentication. To improve security, implement an authentication mechanism such as OAuth2 or JWT (JSON Web Token):
Improved Authentication Using JWT
@RestController
@RequestMapping("/api")
public class MyController {
@Autowired
private JwtService jwtService;
@GetMapping("/data")
@PreAuthorize("hasRole('USER')")
public ResponseEntity<String> getData() {
return ResponseEntity.ok("Sensitive Data");
}
}
Why Use JWT?
JWT enables stateless authentication. It allows your server to authenticate users without the need to maintain session state, thus improving performance and scalability.
For a more detailed understanding, check out Spring Security's guide.
2. Lack of API Rate Limiting
Another common mistake is failing to implement rate limiting. Without it, malicious actors can overwhelm your API with excessive requests, leading to denial-of-service (DoS) attacks.
How to Implement Rate Limiting
@Bean
public FilterRegistrationBean<RateLimiterFilter> rateLimiterFilter() {
FilterRegistrationBean<RateLimiterFilter> registrationBean = new FilterRegistrationBean<>();
registrationBean.setFilter(new RateLimiterFilter());
registrationBean.addUrlPatterns("/api/*");
return registrationBean;
}
Why is Rate Limiting Important?
Rate limiting protects your API by controlling the number of requests a user can make within a given timeframe. This prevents misuse and ensures that all users receive equitable service.
Learn more about implementing rate limiting in your Spring application in this comprehensive article.
3. Exposing Sensitive Information in Error Messages
Developers often overlook the sensitivity of error messages. Detailed errors can expose vulnerabilities and help attackers understand your API's inner workings.
Example of Poor Error Handling
@RestController
public class ErrorController {
@ExceptionHandler(Exception.class)
public ResponseEntity<String> handleAllExceptions(Exception ex) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR)
.body("Error Occurred: " + ex.getMessage());
}
}
Improving Error Handling
@ExceptionHandler(Exception.class)
public ResponseEntity<String> handleAllExceptions(Exception ex) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR)
.body("An error occurred - please try again later.");
}
Why is Error Management Crucial?
By providing generic error messages, you reduce the amount of helpful information that could be exploited by an attacker. Always log detailed error information internally, but return user-friendly messages to the client.
For further reading on error handling in Spring, refer to this Spring documentation.
4. Overlooking CORS Configuration
Cross-Origin Resource Sharing (CORS) is an important aspect of API security. Failing to configure CORS restrictions can allow unwanted domains to access your API.
Example of Unrestricted CORS
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**").allowedOrigins("*");
}
}
Proper CORS Configuration
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**").allowedOrigins("https://yourdomain.com");
}
}
Why Configure CORS?
Restricting allowed origins to specific domains protects your API from unauthorized access while still allowing your legitimate frontend application to make calls.
For additional CORS configurations and best practices, visit Spring's CORS documentation.
5. Ignoring Secure Transport
Another major pitfall is neglecting secure transport protocols. Always enforce HTTPS for your API.
Enforcing HTTPS
To enforce HTTPS in a Spring Boot application, you can use the following properties in your application.properties
file:
server.port=8443
server.ssl.key-store=classpath:keystore.jks
server.ssl.key-store-password=yourpassword
server.ssl.keyStoreType=JKS
server.ssl.keyAlias=youralias
Why Use HTTPS?
HTTPS encrypts data in transit, ensuring that sensitive information exchanged between the client and server cannot be intercepted easily. Protecting user credentials and other sensitive data is non-negotiable.
You can explore a more comprehensive guide on enforcing HTTPS in Spring applications in this Medium article.
6. Insufficient Input Validation
Input validation is crucial in protecting your API from various attacks, including SQL injection and cross-site scripting (XSS).
Example of Lack of Input Validation
@PostMapping("/user")
public ResponseEntity<String> createUser(@RequestBody User user) {
// Logic to create user
return ResponseEntity.ok("User created successfully");
}
Implementing Input Validation
Use annotations from the Java Validation API:
@PostMapping("/user")
public ResponseEntity<String> createUser(@Valid @RequestBody User user) {
// Logic to create user
return ResponseEntity.ok("User created successfully");
}
Why Validate Input?
Validating user input ensures that you only process data conforming to expected formats, thus lowering the risk of injection and other exploits. For a deeper understanding of validation in Spring, refer to the Spring Boot validation documentation.
A Final Look
Securing your REST APIs using Spring is an essential practice to protect sensitive data and maintain user trust. By avoiding these common pitfalls, you can enhance the security of your APIs significantly. Understanding the importance of robust authentication, CORS configurations, HTTPS enforcement, and input validation will go a long way in building a secure application.
For further exploration of security practices, consider looking into Spring Security and other reputable resources such as OWASP.
Remember, security is a continual process. Stay updated with the latest security trends and best practices to keep your API safe from evolving threats. Happy coding!
Checkout our other articles