Top Techniques to Defend Against SQL Injection Attacks
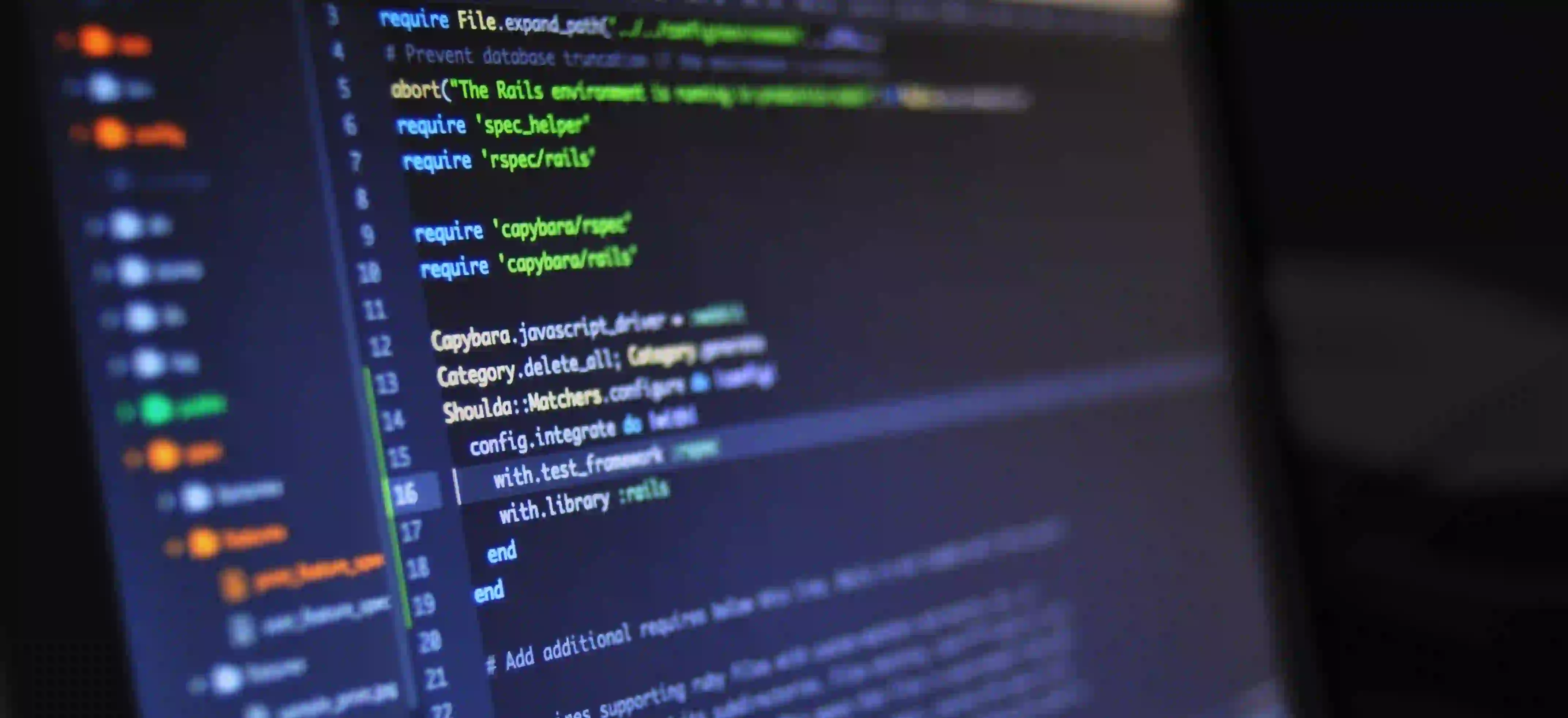
Top Techniques to Defend Against SQL Injection Attacks
SQL Injection attacks are among the most common threats to web applications today. Attackers exploit vulnerabilities in an application's software to execute arbitrary SQL code on the backend database. This can lead to unauthorized access to sensitive data, data corruption, or even complete system compromise. In this blog post, we will explore some of the most effective techniques to defend against SQL Injection attacks, ensuring your applications remain secure.
Understanding SQL Injection
Before diving into the techniques, it is crucial to understand what SQL Injection is.
SQL Injection occurs when an attacker manipulates a web application's input data to alter the intended SQL query. For example, consider a simple login form:
String userInput = request.getParameter("username");
String passwordInput = request.getParameter("password");
String sql = "SELECT * FROM users WHERE username = '" + userInput + "' AND password = '" + passwordInput + "'";
In the code above, an attacker might input admin' --
as the username, leading to the execution of the following SQL command:
SELECT * FROM users WHERE username = 'admin' --' AND password = ''
Here, the comment notation (--
) effectively ignores the password check, potentially granting access to the attacker.
1. Use Prepared Statements with Parameterized Queries
One of the most effective ways to prevent SQL Injection is through the use of prepared statements. Prepared statements ensure that SQL code and user input are processed separately, thus mitigating the risk of SQL injection.
Example:
String sql = "SELECT * FROM users WHERE username = ? AND password = ?";
PreparedStatement pstmt = connection.prepareStatement(sql);
pstmt.setString(1, userInput);
pstmt.setString(2, passwordInput);
ResultSet rs = pstmt.executeQuery();
Why Use Prepared Statements?
- Separation of Code and Data: By using parameterized queries, you ensure user inputs never interfere with the SQL code execution.
- Automatic Escaping: JDBC handles type conversions and escaping, reducing the risk of manipulation.
For more details on this topic, visit OWASP’s guide on SQL Injection prevention.
2. Input Validation and Escaping
Input validation is another crucial defense mechanism. Always validate user inputs before processing them. Use whitelisting techniques—allow only expected data formats and reject everything else.
Example:
if(userInput.matches("[a-zA-Z0-9]*")) {
// proceed with database operations
} else {
// reject or sanitize input
}
Why Validate Input?
- Catch Invalid Data Early: By rejecting erroneous inputs upfront, you reduce the risk of malicious data reaching the database.
- Improve Application Stability: Validated inputs lead to fewer errors and unexpected behaviors in your application.
3. Implement Least Privilege Principle
The least privilege principle means that users should only have the minimal level of access necessary to perform their tasks. This practice limits the potential damage caused by an SQL injection attack.
Example:
Instead of using a high-privilege account (such as db_admin
), create a dedicated database user for your application with limited permissions:
GRANT SELECT, INSERT, UPDATE ON user_db TO 'app_user'@'localhost';
Why Implement Least Privilege?
- Contain Damage: If an attacker gains access, limited privileges restrict their ability to execute harmful actions.
- Reduced Risk Exposure: Limiting access minimizes the attack surface for SQL Injection and other vulnerabilities.
4. Regularly Update and Patch Systems
Regular software updates and patches are vital for maintaining application security. Out-of-date systems may have vulnerabilities that attackers can exploit.
Why Keep Systems Updated?
- Fix Known Vulnerabilities: Software providers regularly release updates to fix security holes that could be exploited.
- Enhance Security Features: Newer versions may include additional security measures.
Always stay informed about available updates for your database and web application frameworks to ensure you are not leaving gaps that could be exploited.
5. Use Web Application Firewalls (WAF)
Web Application Firewalls must be integral to your security strategy. A WAF can filter and monitor HTTP requests, blocking potential SQL injections before they even reach your application.
Why Use a WAF?
- Real-time Protection: A WAF can provide immediate scanning of incoming traffic and responses, identifying potentially harmful requests.
- Multi-layer Defense: It adds an additional layer of security alongside prepared statements and input validation.
Several reputable WAF providers include Cloudflare and Imperva.
6. Perform Regular Security Audits and Penetration Testing
Conducting regular security audits and penetration tests is essential for identifying and addressing potential vulnerabilities, including SQL Injection risks.
Why Conduct Audits?
- Proactive Risk Management: Regular testing helps you identify security weaknesses before they can be exploited.
- Compliance Requirements: Many regulations require regular security audits to protect sensitive data.
Implementing a continuous security testing routine will help keep your application resilient.
A Final Look
SQL Injection attacks pose a serious threat to web applications. However, by understanding the nature of these attacks and employing robust defensive techniques, you can significantly enhance your application's security.
Using prepared statements, validating inputs, implementing the least privilege principle, keeping systems updated, employing WAFs, and executing regular security audits will provide comprehensive protection against SQL injection attacks. Remember, security is not a product but a process—one that evolves continually. Stay vigilant, educate your development team, and adapt your strategies as needed.
By following these practices, you can effectively mitigate the risks posed by SQL Injection and protect your sensitive data. For ongoing learning, consider reviewing resources from leading security organizations for the latest trends and defense practices.
Additional Resources
Become a proactive part of the solution, safeguarding your applications for a more secure technological future.