Streamlining Local Java Dev: Skaffold Setup Struggles
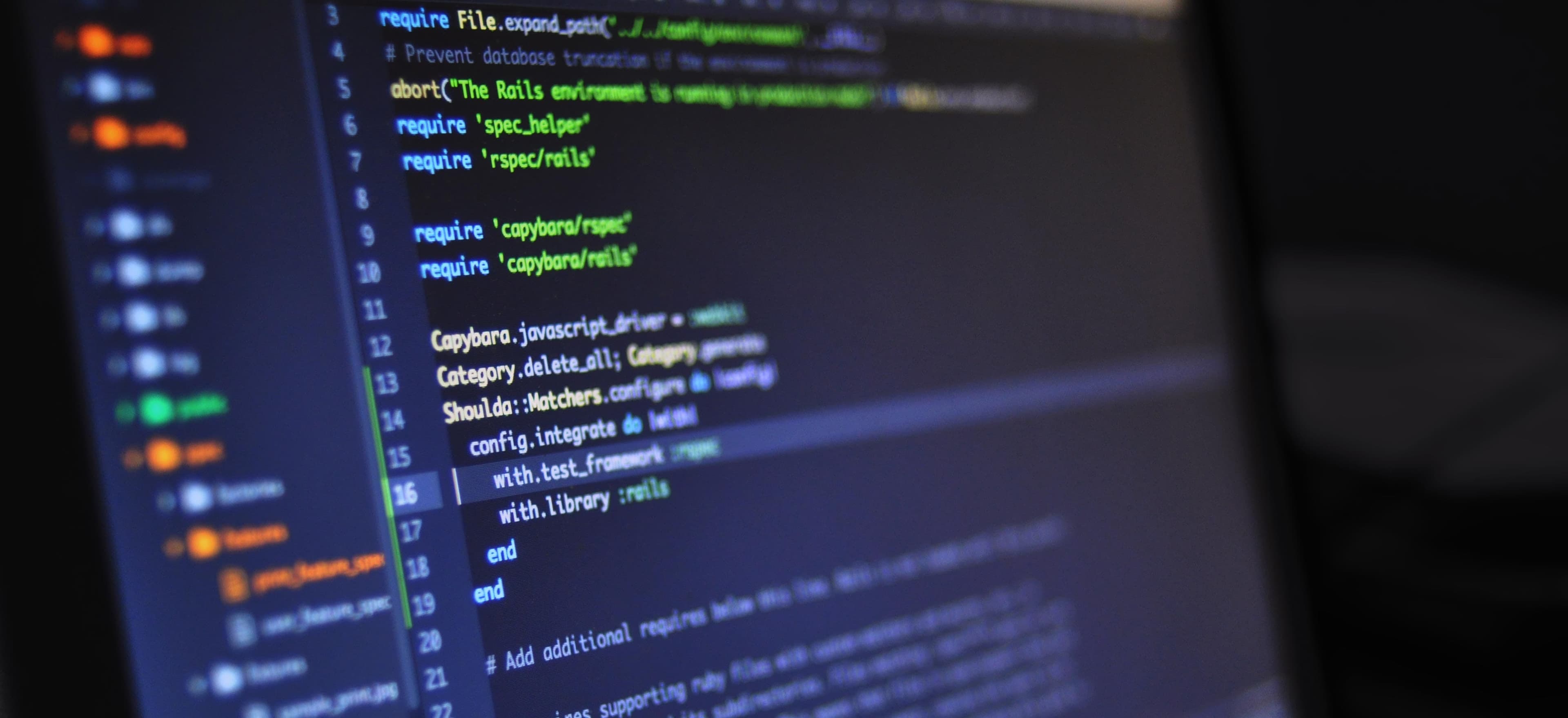
- Published on
Streamlining Local Java Development: Skaffold Setup Struggles
In recent years, containerization has transformed the way we develop, test, and deploy applications. Among the tools that ease this transition is Skaffold, a command-line tool that facilitates continuous development for Kubernetes applications. If you're a Java developer looking to leverage the advantages of containerized environments, you’ve probably encountered some challenges in setting up Skaffold for your local development. This blog post aims to guide you through the process, troubleshooting common setup struggles along the way.
Understanding the Basics of Skaffold
Before diving into the setup, let's ensure we understand what Skaffold does. Skaffold automates the workflow for building, pushing, and deploying your applications to Kubernetes. The primary benefit? It significantly shortens the feedback loop during development, as you can see your changes in real time without having to manually rebuild and redeploy every time.
Why Use Skaffold?
- Speed: Shorten development cycles by automating tasks like building images and deploying them to your Kubernetes cluster.
- Simplicity: Manage multi-environment setups effortlessly.
- Integration: Works seamlessly with various CI/CD tools, making it easier to craft your complete development pipeline.
Setting Up Your Local Environment
To effectively use Skaffold with your Java applications, follow these steps:
Prerequisites
-
Java Development Kit (JDK): Ensure you have JDK 8 or later installed. You can check your Java version using the command:
java -version
-
Docker: Install Docker on your machine, as Skaffold utilizes Docker containers for building images. Docker's official site provides comprehensive installation instructions.
-
Skaffold: You can install Skaffold via Homebrew (on macOS) or by downloading the latest release from the Skaffold GitHub Page.
brew install skaffold
Creating Your Java Application
Let’s start by creating a simple Java Web Application. You can use Spring Boot, which is a powerful framework for building web applications in Java.
mkdir my-spring-app
cd my-spring-app
Initialize a new Spring Boot application with the following command:
curl https://start.spring.io/starter.zip -d dependencies=web -d name=my-spring-app -o my-spring-app.zip
unzip my-spring-app.zip
cd my-spring-app
This will create a Spring Boot application with the Web dependency included.
Writing a Simple Controller
Now, let’s create a simple REST controller. Open the src/main/java/com/example/demo/DemoApplication.java
file and edit it as follows:
package com.example.demo;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
public class DemoApplication {
public static void main(String[] args) {
SpringApplication.run(DemoApplication.class, args);
}
}
@RestController
class HelloController {
@GetMapping("/hello")
public String hello() {
return "Hello, World!";
}
}
Commentary on the Code
- @SpringBootApplication: It's a convenience annotation that adds all of
@Configuration
,@EnableAutoConfiguration
, and@ComponentScan
. - @RestController: This annotation makes it easier to create RESTful web services. By including the
@GetMapping
, we've defined a simple endpoint that returns a string when accessed.
Setting Up Skaffold
Now, let’s integrate Skaffold into your Java application.
Creating a Skaffold Configuration File
To configure Skaffold, create a skaffold.yaml
file at the root of your project:
apiVersion: skaffold/v2beta17
kind: Config
metadata:
name: my-spring-app
build:
artifacts:
- image: my-spring-app
docker:
dockerfile: Dockerfile
deploy:
kubectl:
manifests:
- k8s-*
Adding Dockerfile
Next, you’ll need a Dockerfile to specify how Skaffold should build your application. Create a Dockerfile
in the root directory of your project:
FROM openjdk:11-jre-slim
VOLUME /tmp
COPY target/my-spring-app-0.0.1-SNAPSHOT.jar app.jar
ENTRYPOINT ["java","-jar","/app.jar"]
Explanation of Dockerfile
- FROM openjdk:11-jre-slim: We’re using an official OpenJDK image, specifically a slim variant, which reduces the image size.
- COPY target/my-spring-app-0.0.1-SNAPSHOT.jar app.jar: This step copies the built JAR file into the container. Ensure that you build your application before running Skaffold.
Kubernetes Manifests
Create a directory named k8s
for your Kubernetes manifests, and add a deployment.yaml
and service.yaml
file.
deployment.yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-spring-app
spec:
replicas: 1
selector:
matchLabels:
app: my-spring-app
template:
metadata:
labels:
app: my-spring-app
spec:
containers:
- name: my-spring-app
image: my-spring-app
ports:
- containerPort: 8080
service.yaml
apiVersion: v1
kind: Service
metadata:
name: my-spring-app
spec:
type: NodePort
ports:
- port: 8080
targetPort: 8080
selector:
app: my-spring-app
Explanation of Kubernetes Manifests
- Deployment: Defines the desired state for your application, including the image that should be used.
- Service: Exposes your application on a network, allowing users to access it using a consistent IP address.
Running Your Application with Skaffold
Now that everything is set up, you can start your application using Skaffold:
./mvnw clean package
skaffold dev
This command not only builds your application but also deploys it to your Kubernetes cluster in development mode. It will watch for code changes and automatically sync them, allowing you to see updates almost instantly.
Common Setup Struggles and Troubleshooting
-
Kubernetes Context: Ensure your Kubernetes context is set correctly. You can check it with the following command:
kubectl config current-context
-
Port Forwarding: If you can't access your application, you may need to set up port forwarding. Use this command to forward local port 8080 to your service:
kubectl port-forward service/my-spring-app 8080:8080
-
Image Not Found: If you encounter errors related to the Docker image not being found, ensure that the image is correctly built and tagged as specified in your Skaffold configuration.
The Closing Argument
Skaffold is a powerful tool that can drastically improve your local development workflow for Java applications. Despite some initial setup struggles, using Skaffold effectively allows for rapid feedback and iterative development. By following the steps outlined in this post, you can streamline your Java development experience in a Kubernetes environment.
Advanced tools like Skaffold, along with Java frameworks like Spring Boot, are paving the way for modern development practices, enabling developers to build, deploy, and manage robust applications with ease.
For more resources on Java development and Kubernetes, you can check the Java Spring Documentation and Kubernetes Official Documentation.
Happy coding!