Mastering Dynamic Queries in Spring Data Solr
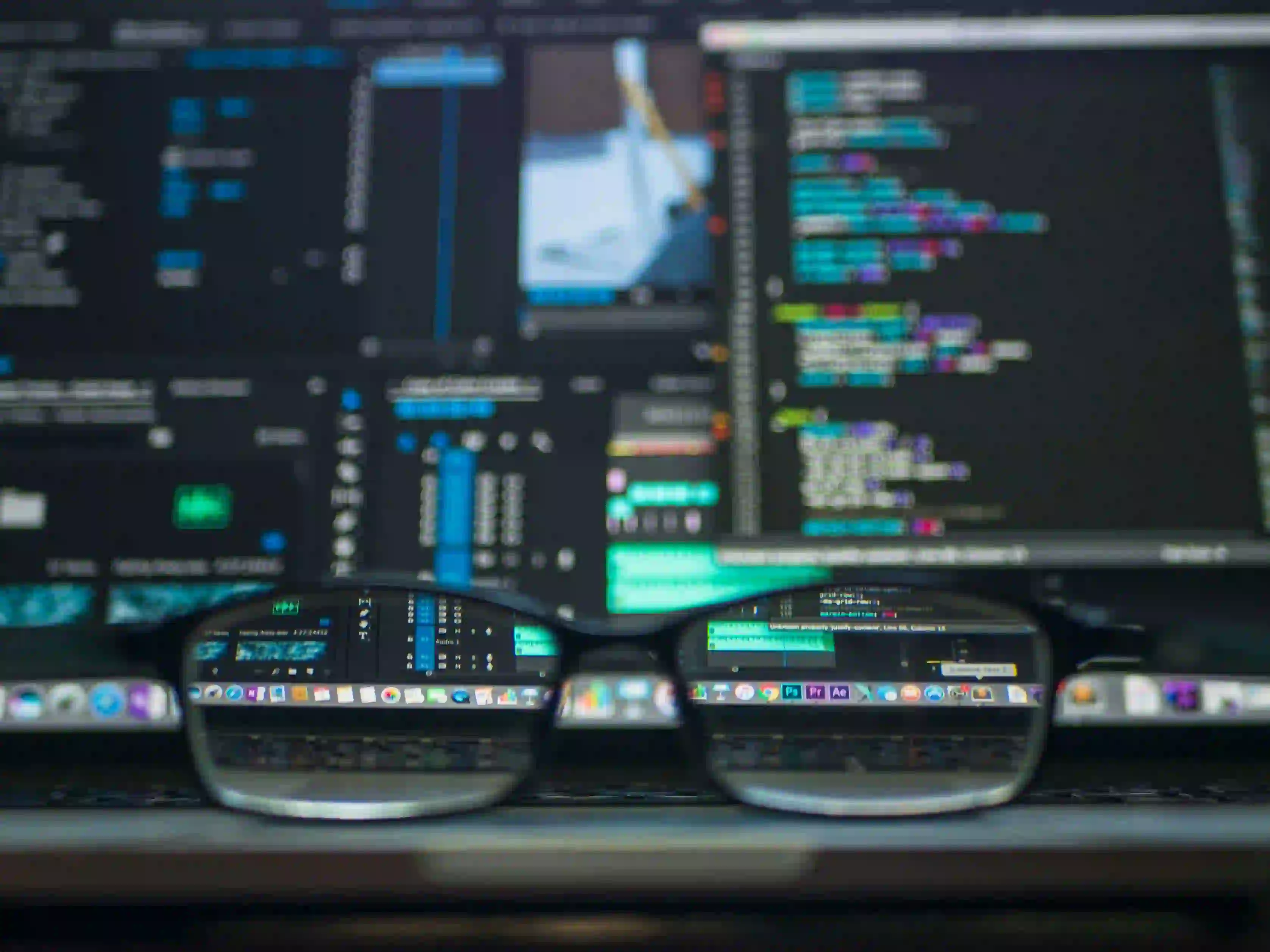
Mastering Dynamic Queries in Spring Data Solr
Spring Data Solr is a powerful framework that simplifies the integration of Solr search capabilities into Spring applications. It allows developers to create, manage, and execute queries against Solr seamlessly. One of the standout features of Spring Data Solr is its ability to create dynamic queries. This post will walk you through the essentials of mastering dynamic queries in Spring Data Solr, with engaging discussions, practical examples, and insightful commentary.
Table of Contents
- Understanding Dynamic Queries
- Setting Up Spring Data Solr
- Creating Dynamic Queries
- Using Query Builders for Dynamic Searches
- Error Handling in Dynamic Queries
- Best Practices for Dynamic Queries
- Conclusion
Understanding Dynamic Queries
Dynamic queries allow for more flexible database interactions. Unlike static queries, which are hardcoded and fixed, dynamic queries adapt to user input or other parameters at runtime. For instance, use cases include search filters in e-commerce websites or custom reporting tools. Dynamic queries enhance user experience by providing tailored results based on specific criteria.
Why Choose Solr?
Apache Solr is a robust search platform built on Apache Lucene, known for its powerful full-text search capabilities, faceted search, and extensive support for various content types. Integrating Solr with Spring Data provides a cohesive development experience, allowing you to leverage the strengths of both platforms.
Setting Up Spring Data Solr
Before diving into dynamic queries, you need to set up your Spring Data Solr project. Here are the key steps:
1. Add Dependencies
Add the following dependencies in your pom.xml
if you are using Maven:
<dependency>
<groupId>org.springframework.data</groupId>
<artifactId>spring-data-solr</artifactId>
<version>4.0.0</version> <!-- Use the latest stable version -->
</dependency>
<dependency>
<groupId>org.apache.solr</groupId>
<artifactId>solr-solrj</artifactId>
<version>8.10.0</version> <!-- Use the latest stable version -->
</dependency>
These dependencies provide you the libraries required to work with Solr in a Spring environment.
2. Configure Solr
You will need to configure your application properties:
spring:
data:
solr:
solr-url: http://localhost:8983/solr/mycore
username: your-username
password: your-password
Make sure to replace mycore
with the name of your core in Solr.
3. Create Domain Objects
Define your entities, also known as documents in Solr.
import org.springframework.data.annotation.Id;
import org.springframework.data.solr.core.mapping.SolrDocument;
@SolrDocument(collection = "mycore")
public class Product {
@Id
private String id;
private String name;
private String category;
// Getters and setters
}
Creating Dynamic Queries
Now that your setup is complete, we can dive into dynamic querying. Spring Data Solr provides an expressive API for constructing dynamic queries.
Using Query
and Criteria
You can create a dynamic query using the Query
and Criteria
classes. Here’s how:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.solr.core.SolrTemplate;
import org.springframework.data.solr.core.query.Criteria;
import org.springframework.data.solr.core.query.Query;
import org.springframework.data.solr.core.query.SimpleQuery;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class ProductService {
@Autowired
private SolrTemplate solrTemplate;
public List<Product> searchProducts(String name, String category) {
Criteria criteria = new Criteria("name").contains(name)
.or(new Criteria("category").is(category));
Query query = new SimpleQuery(criteria);
return solrTemplate.queryForList(query, Product.class);
}
}
Why This Matters
- Flexibility: The code allows the search method to be flexible, accommodating different search terms by using
contains
andis
. - Reusability: A single method can handle various search criteria without the need for multiple distinct methods.
Using Query Builders for Dynamic Searches
Dynamic queries often require complex logic depending on various parameters. You can leverage Solr's Query Builders to create reusable search logic.
import org.springframework.data.solr.core.query.Criteria;
import org.springframework.data.solr.core.query.Query;
import org.springframework.data.solr.core.query.SimpleQuery;
public class DynamicQueryBuilder {
public static Query buildQuery(String name, String category) {
Criteria criteria = new Criteria("*:*"); // Start with all documents
if (name != null && !name.isEmpty()) {
criteria = criteria.and(new Criteria("name").contains(name));
}
if (category != null && !category.isEmpty()) {
criteria = criteria.and(new Criteria("category").is(category));
}
return new SimpleQuery(criteria);
}
}
Why Use a Query Builder?
- Scalability: As your requirements grow, modifying or extending your querying capabilities requires only a few adjustments.
- Separation of Concerns: It keeps your service code clean and focused on business logic.
Error Handling in Dynamic Queries
Handling errors gracefully is crucial for a good user experience. Here’s how to add robust error handling to your querying logic.
public List<Product> searchProducts(String name, String category) {
try {
Query query = DynamicQueryBuilder.buildQuery(name, category);
return solrTemplate.queryForList(query, Product.class);
} catch (Exception e) {
throw new RuntimeException("Error executing Solr query", e);
}
}
Best Practices for Dynamic Queries
-
Limit Queries: Always limit the fields you query against. For example, instead of querying all fields with
*:*
, specify fields for better performance. -
Parameter Validation: Validate input parameters to avoid unnecessary queries or potential SQL injection by ensuring values are sanitized and conform to expected formats.
-
Caching: Leverage query caching mechanisms to enhance performance when dealing with frequently executed queries.
-
Profiling Queries: Regularly profile your Solr queries using the Solr Admin interface, allowing you to identify slow queries and adjust your indices accordingly.
-
Documentation: Always document dynamic queries for future reference and to facilitate knowledge sharing among team members.
The Closing Argument
Mastering dynamic queries in Spring Data Solr unlocks a plethora of possibilities for creating responsive, user-driven applications. With the ability to craft flexible queries, adapt to user input, and scale with your application, you can ensure that your search capabilities meet the expectations of modern applications.
For more in-depth tutorials and advanced techniques, feel free to check the official Spring Data Solr Documentation and the Apache Solr Reference Guide.
Happy querying!