Common Pitfalls When Using Groovy's Node XMLParser
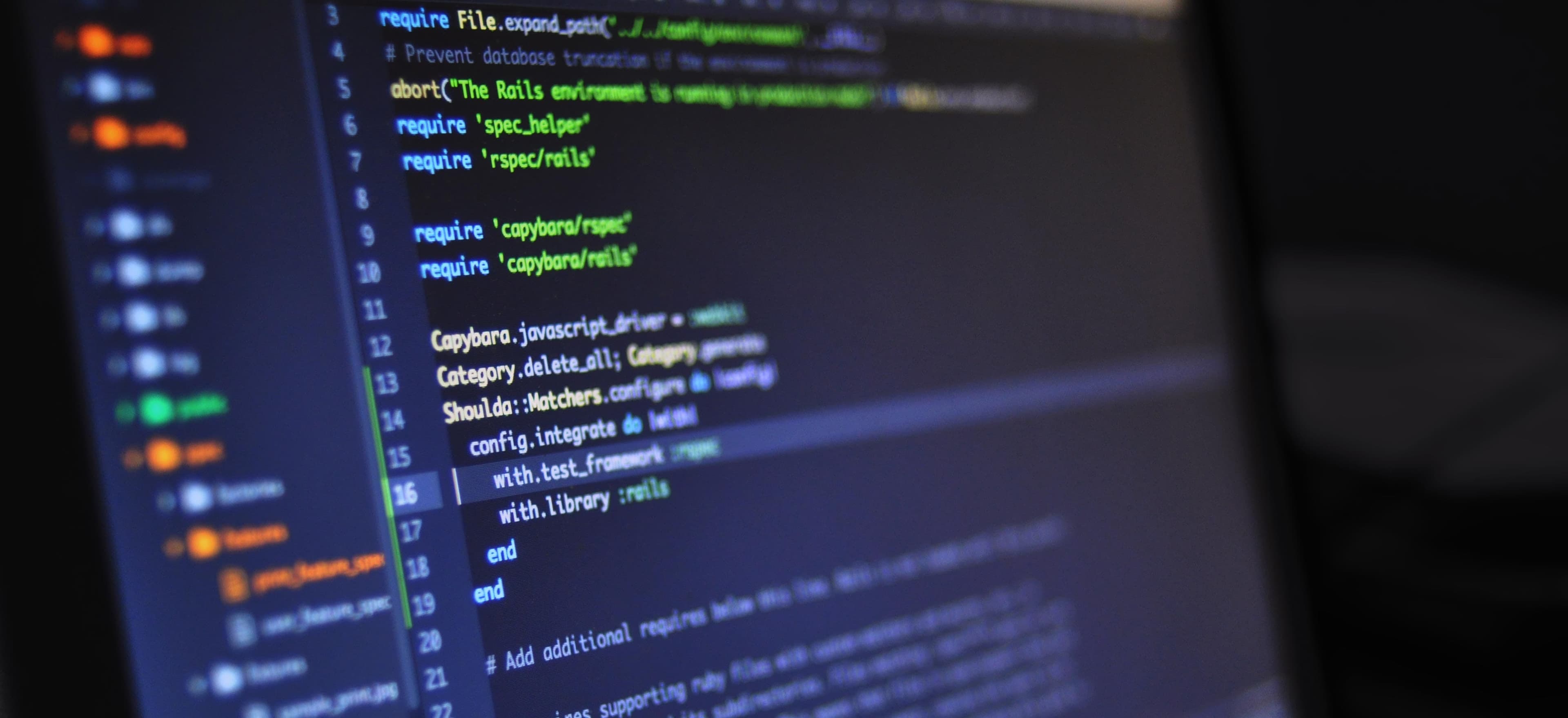
- Published on
Common Pitfalls When Using Groovy's Node XMLParser
Groovy is a powerful language that integrates seamlessly with Java, providing a more concise and expressive syntax while retaining the robustness of Java’s capabilities. Among its many features, Groovy's Node XMLParser stands out for simplifying XML parsing and manipulation. However, while working with Node XMLParser, developers can easily encounter pitfalls that can lead to inefficient code or runtime errors. In this blog post, we will explore some of these common pitfalls and how you can avoid them.
What is Node XMLParser?
Before diving into the pitfalls, it's essential to understand what Node XMLParser is. The Node XMLParser is a part of Groovy’s groovy.util.XmlSlurper
and groovy.util.XmlParser
classes. It allows for easy parsing and handling of XML documents using a node-based approach, which abstracts a lot of the complexities associated with traditional XML parsers.
Here's a simple example for clarity:
import groovy.xml.XmlSlurper
def xml = '''<books>
<book>
<title>Groovy in Action</title>
<author>James Strachan</author>
</book>
<book>
<title>Learning Groovy</title>
<author>Andrew Glover</author>
</book>
</books>'''
def parser = new XmlSlurper()
def books = parser.parseText(xml)
println books.book[0].title // Output: Groovy in Action
In this code snippet, we use XmlSlurper
to parse a string containing XML data, allowing us to access the data nodes easily.
Pitfall 1: Ignoring Namespace Handling
One of the most frequent pitfalls developers encounter when using Node XMLParser is neglecting XML namespaces. When XML documents include namespaces, it can lead to runtime errors when querying elements.
Example of Namespace Issue
Consider the following XML:
<book xmlns:ns="http://example.com/ns">
<ns:title>Learning Groovy</ns:title>
<ns:author>Andrew Glover</ns:author>
</book>
If you attempt to access the title without specifying the namespace, like so:
def title = books.title.text()
You will end up with an error because the parser will not recognize the title under its proper namespace.
Solution
Always ensure that you handle namespaces correctly, either by using the full namespace in your queries or by registering the namespace mappings beforehand. Here's how you can do that:
def ns = new groovy.xml.Namespace("http://example.com/ns", "ns")
def title = books.'ns:title'.text()
println title // Output: Learning Groovy
For more details on namespaces in XML, refer to the W3C XML Namespace Recommendation.
Pitfall 2: Overlooking Error Handling
When dealing with XML, parsing errors are quite common. These can arise due to malformed XML or logical errors in your structure. Failing to implement adequate error handling can lead to the entire application crashing.
Example of Error Handling
Consider the following incorrect XML structure:
<books>
<book>
<title>Groovy in Action<title> <!-- Missing closing tag -->
</book>
</books>
If you try to parse this, the result will be an exception that you might not have anticipated.
Solution
Whenever you're parsing XML, wrap your parsing logic in a try-catch block. This way, you can catch exceptions gracefully and log appropriate error messages.
try {
def books = parser.parseText(xml)
} catch (Exception e) {
println "Failed to parse XML: ${e.message}"
}
Pitfall 3: Inefficient Access Patterns
Node XMLParser allows you to access XML nodes easily, but if you're not careful, you might end up with inefficient access patterns that could slow down performance, especially with large XML files.
Example of Inefficient Access
Consider the following code where you loop through nodes multiple times:
books.book.each { book ->
println book.title.text()
}
If you perform additional operations on each title later on, your performance could diminish rapidly.
Solution
To improve efficiency, collect necessary data in one go:
def titles = books.book*.title.text()
titles.each { title ->
println title
}
This approach utilizes Groovy's spread operator (*.
) to collect titles in a single pass, improving both readability and performance.
Pitfall 4: Not Utilizing Groovy’s Built-in Features
Many developers coming from a Java background often overlook Groovy's powerful features, leading to verbose and unnecessarily complex code.
Example of Verbose Code
Here’s a less efficient way of extracting information:
def titles = []
books.book.each { book ->
titles << book.title.text()
}
Solution
Instead, you can use Groovy's list comprehension:
def titles = books.book.collect { it.title.text() }
This method is far more concise and leverages Groovy's capabilities to create a new list while iterating through the nodes.
Pitfall 5: Misunderstanding the Difference Between XmlParser and XmlSlurper
Many developers confuse XmlParser
and XmlSlurper
, assuming they function identically. In reality, they serve different purposes.
XmlParser vs. XmlSlurper
- XmlParser is geared for parsing well-formed XML documents. It loads the entire document into memory and allows for DOM-like traversal.
- XmlSlurper, on the other hand, provides a streaming approach, allowing you to parse large XML documents without needing to hold the entire document in memory.
When to Use Which
If you are dealing with large XML files, XmlSlurper
is a better choice due to its inherent efficiency. Conversely, if the XML document is small and well-structured, XmlParser
might be optimal for simpler parsing logic.
Example Using XmlSlurper for Large XML Files
def xmlFile = new File("large-file.xml")
def slurper = new XmlSlurper().parse(xmlFile)
This snippet demonstrates how to efficiently parse a potentially large XML file with minimal memory usage.
The Closing Argument
Avoiding pitfalls when using Groovy's Node XMLParser is essential for writing clean, efficient, and error-free code. By being mindful of namespace handling, implementing sound error resolution, optimizing access patterns, leveraging Groovy's features, and understanding the distinctions between XmlParser
and XmlSlurper
, you can avoid the common traps that many developers fall into.
For further reading on Groovy XML processing techniques, check out Groovy's XML documentation.
Happy coding, and may your XML parsing be efficient and error-free!