Overcoming Common Spring Social Setup Issues
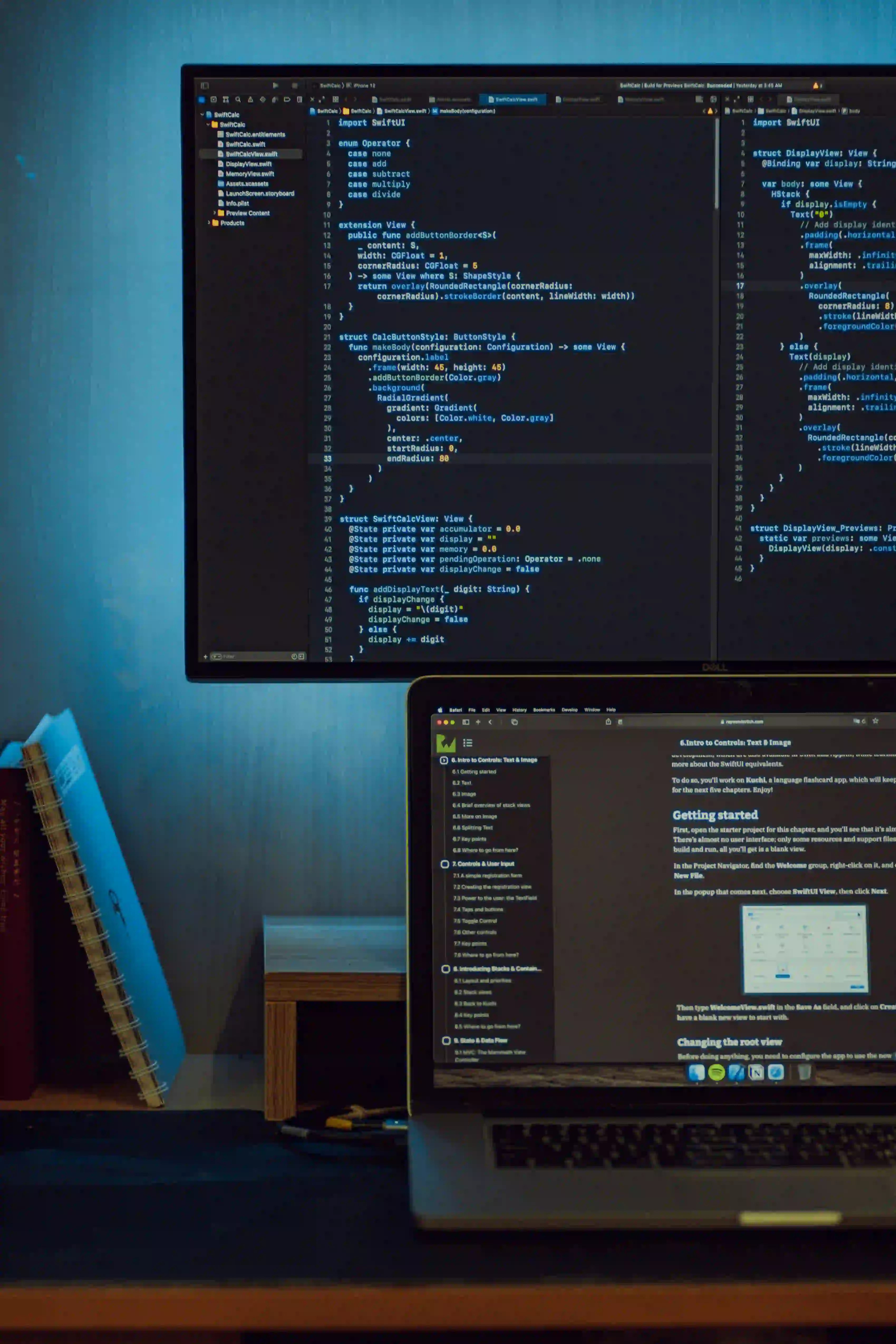
Overcoming Common Spring Social Setup Issues
Setting up Spring Social can often seem daunting, especially for developers who are new to integrating social networking features into their applications. Spring Social is a powerful framework that simplifies the process of connecting to social web APIs. However, as with any framework, challenges can arise during the setup phase.
In this blog post, we'll explore common issues developers face when setting up Spring Social and provide actionable solutions to overcome them. Let's get started!
Understanding Spring Social
Before diving into troubleshooting, it’s essential to understand what Spring Social is and why you might want to use it. Spring Social provides a way to build applications that interact with various social media services—like Facebook, Twitter, and LinkedIn—by allowing developers to easily integrate social features into their applications.
This framework manages authentication, API interactions, and even responses from the social platforms, which saves developers a significant amount of time by not needing to reinvent the wheel.
Common Setup Issues
1. Dependency Management Problems
When setting up Spring Social, you might encounter issues related to Maven or Gradle dependencies. Missing or incompatible dependencies can prevent your application from compiling or running correctly.
Solution:
Ensure your pom.xml
(for Maven) or build.gradle
(for Gradle) is set up correctly. Here’s an example of how your pom.xml
should look:
<dependency>
<groupId>org.springframework.social</groupId>
<artifactId>spring-social-config</artifactId>
<version>2.0.2.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework.social</groupId>
<artifactId>spring-social-core</artifactId>
<version>2.0.2.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework.social</groupId>
<artifactId>spring-social-security</artifactId>
<version>2.0.2.RELEASE</version>
</dependency>
Make sure you’re using compatible versions. It's critical to check the official Spring Social documentation for the latest releases.
2. Authentication Configuration Issues
Authentication is a cornerstone of social integration. Misconfigurations can lead to authentication failures, leaving users unable to connect their social accounts.
Solution:
Follow these steps meticulously while configuring authentication:
- Consumer Keys and Secrets: Obtain the consumer key and secret from the social platform’s developer console (e.g., Facebook Developers, Twitter Developer).
- Spring Security Setup: Ensure your security configuration allows for OAuth authentication.
Here’s an example of configuring Facebook authentication in your application:
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private ConnectionFactoryLocator connectionFactoryLocator;
@Autowired
private UsersConnectionRepository usersConnectionRepository;
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/", "/signup").permitAll()
.anyRequest().authenticated()
.and()
.social()
.connectionFactoryLocator(connectionFactoryLocator)
.usersConnectionRepository(usersConnectionRepository)
.and()
.addFilterBefore(socialAuthenticationFilter(), BasicAuthenticationFilter.class);
}
private Filter socialAuthenticationFilter() {
// Configuration for the social authentication filter
}
}
By ensuring you have the correct consumer keys and properly configured filters, you can mitigate common authentication failures.
3. URL Redirect Issues
Redirect URIs can become a point of confusion. If URLs aren't set up properly, you may find yourself facing 404 errors or unauthorized access messages.
Solution:
- Callback URLs: Ensure the callback URL registered with the social platform matches the URL in your application.
- Spring Redirect Handling: Configure your application to handle these redirects seamlessly. Use the following code to define a redirect URL:
@Bean
public OAuth2Operations oauth2Operations() {
return new OAuth2Template(
clientId,
clientSecret,
authorizeUrl,
accessTokenUrl
);
}
@GetMapping("/login")
public String login() {
String redirectUrl = oauth2Operations.buildAuthorizeUrl(...);
return "redirect:" + redirectUrl;
}
Double-check these values against your application settings. Properly setting these reduces transition errors when users engage with social login features.
4. API Calls Failing
After setting up Spring Social properly, you might encounter issues where API calls fail or return unexpected results. This can be due to permission issues or incorrect scopes.
Solution:
- Checking Permissions: Ensure that your application requests the right permissions from users. Scopes define what data you can access. For example:
FacebookTemplate facebookTemplate = new FacebookTemplate(accessToken);
User userProfile = facebookTemplate.userOperations().getUserProfile();
Verify what permissions you've requested in the Facebook Developer Console, and modify your request if needed.
- Error Handling: Implement error handling to catch and log issues. You might use try-catch blocks to understand where an API call failed.
try {
User userProfile = facebookTemplate.userOperations().getUserProfile();
} catch (Exception e) {
logger.error("Error fetching user profile: ", e);
}
5. Integration Testing
Integration tests can be tricky but are essential when dealing with external APIs. You may find your tests not passing due to misconfigurations or incorrect assumptions about API behavior.
Solution:
- Mocking External Services: Use frameworks like Mockito to mock external API calls during testing.
- Test Profiles: Configure different application profiles to ensure configurations for testing do not mix with production settings.
Here’s a brief example using Mockito:
@RunWith(MockitoJUnitRunner.class)
public class SocialServiceTest {
@InjectMocks
private SocialService socialService;
@Mock
private FacebookTemplate facebookTemplate;
@Test
public void testGetUserProfile() {
User mockUser = new User("name", "email");
when(facebookTemplate.userOperations().getUserProfile()).thenReturn(mockUser);
User userProfile = socialService.getUserProfile();
assertEquals("name", userProfile.getName());
}
}
Mocking allows you to focus on your testing logic without hitting the actual social API repeatedly.
Lessons Learned
Integrating Spring Social into your Java application doesn’t have to be a headache. By understanding these common issues and their resolutions, you can avoid pitfalls and create seamless social features more efficiently. The key takeaways include ensuring proper dependency management, carefully following authentication procedures, accurately configuring URLs, and implementing robust error handling.
Remember to keep the Spring Social documentation close at hand during development, as it provides invaluable insights into the latest features and best practices.
With careful setup, your application can leverage the power of social media integrations effectively, enhancing user experience and expanding engagement.
Should you face any other challenges or if you have insights from your experiences, feel free to share in the comments below! Happy coding!