Troubleshooting Skinning Issues in JavaFX 8 Applications
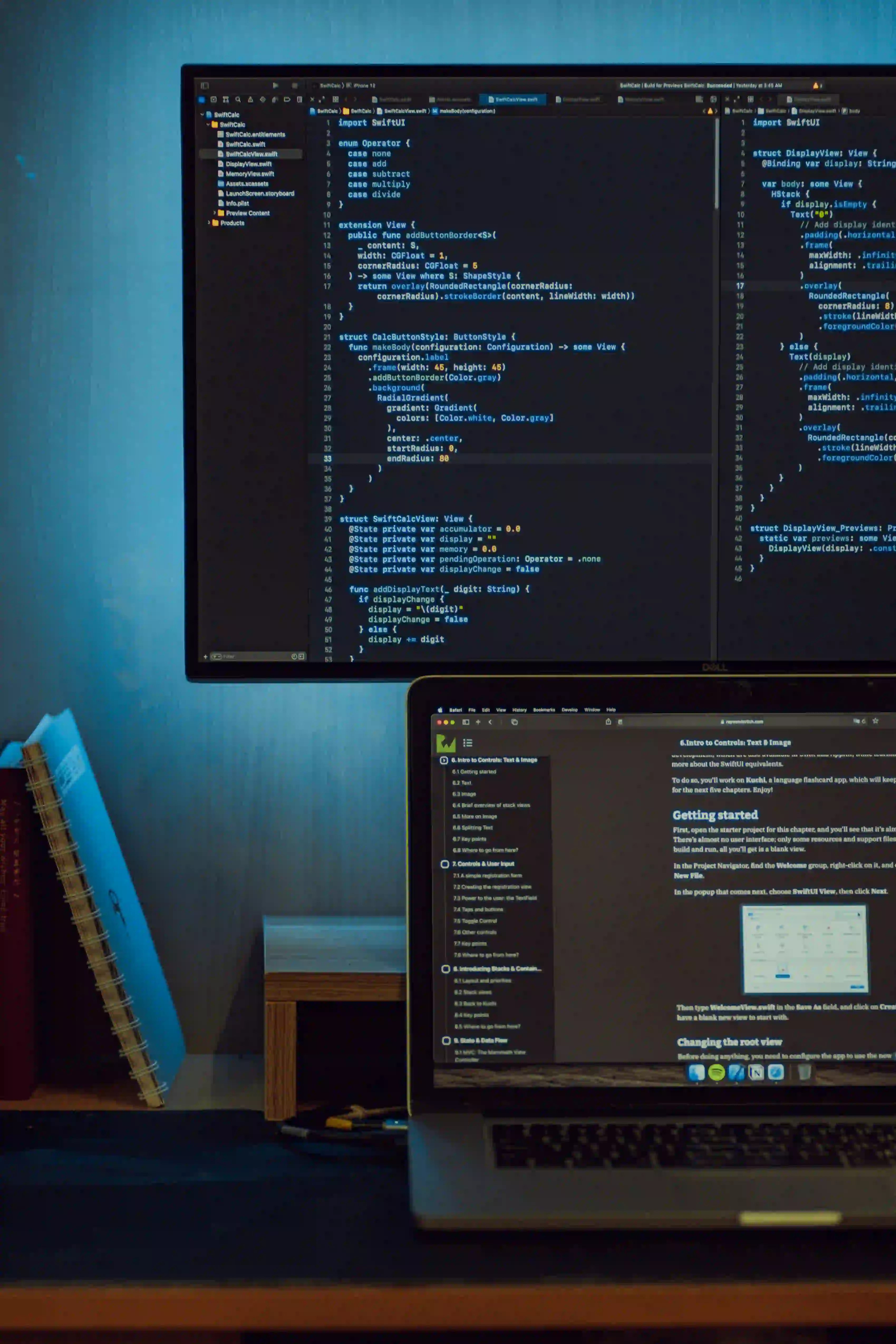
Troubleshooting Skinning Issues in JavaFX 8 Applications
JavaFX is a powerful framework for building rich client applications in Java. One of its core features is the ability to customize the look and feel of your applications using "skinning." However, skinning can sometimes lead to unexpected behavior or visual discrepancies in your application. In this blog post, we will explore common skinning issues encountered in JavaFX 8 applications, how to troubleshoot them, and provide solutions.
Understanding Skinning in JavaFX
Skinning in JavaFX is the process of changing the appearance and behavior of UI controls. Each UI control has an associated skin class that defines how it is rendered. The skin is responsible for the visual representation and user interaction of the controls.
Why Skinning Matters in User Experience
A good skin can significantly enhance user experience by making the application visually appealing and intuitive. For instance, custom skins can:
- Improve accessibility
- Align with brand identity
- Facilitate better usability
Defining the Basics
Before diving into troubleshooting, it’s essential to understand a few key concepts:
- Control: A component that extends
javafx.scene.control.Control
. - Skin: A class that implements the
Skin
interface. - Pseudo-classes: CSS-like selectors that allow changing styles based on state.
Common Skinning Issues
Let’s explore some common skinning problems you might encounter:
- Missing Styles
- Incorrect Layouts
- Performance Problems
- Inconsistent Behavior
Missing Styles
Problem: You might find that styles are not applied to certain UI controls.
Solution:
-
Ensure CSS is Loaded: Double-check if the CSS file is correctly referenced and loaded.
☕snippet.javaScene scene = new Scene(root); scene.getStylesheets().add("styles.css");
Why: If the stylesheet is not loaded, none of the styles within it will be applied.
-
Check File Paths: Ensure the paths to your CSS files are correct and accessible from your project.
-
Inspect Pseudo-classes: Verify if your CSS uses pseudo-classes correctly. If the control’s state does not match any defined pseudo-class, styles won’t apply.
Incorrect Layouts
Problem: Custom layouts may render UI controls incorrectly.
Solution:
-
Use Layout Containers: Always nest your controls in appropriate layout containers (like
VBox
,HBox
, orGridPane
) to ensure proper alignment.☕snippet.javaVBox vbox = new VBox(); vbox.getChildren().addAll(button1, button2);
Why: Layout containers manage spaces and positions of child controls, ensuring they appear as intended.
-
Validate Skins: Make sure that your custom skin class properly overrides the layout methods.
☕snippet.javapublic class MyCustomButtonSkin extends SkinBase<MyCustomButton> { public MyCustomButtonSkin(MyCustomButton control) { super(control); layoutChildren(); } }
Why: If layoutChildren is not called, your controls might not render in the expected location.
Performance Problems
Problem: Skinning can sometimes lead to performance issues, especially when using complex skins.
Solution:
-
Optimize Rendering: Reduce the number of nodes created during rendering. Use fewer shapes and effects, as they can be expensive.
-
Batch Operations: Use
Group
nodes to group less frequently changed components, minimizing redraws.☕snippet.javaGroup group = new Group(); group.getChildren().add(icon);
Why: Grouping nodes helps in reducing the rendering cost, which is crucial in complex UIs.
Inconsistent Behavior
Problem: UI elements might not behave as expected under certain conditions.
Solution:
-
Event Handling: Ensure that event handlers are appropriately set on your custom controls.
☕snippet.javabutton.setOnAction(e -> handleAction());
Why: Without handlers, actions triggered by the user may not perform as expected.
-
Debugging State Changes: Track changes in your control’s states and ensure that skins react accordingly to state changes.
☕snippet.javacontrol.pseudoClassStateChanged(myPseudoClass, true);
Why: Pseudo-class states determine how skins should respond visually to user interactions.
Best Practices for Custom Skins
-
Follow Standard Conventions: Always adhere to JavaFX UI control patterns to maintain consistency and ease of understanding.
-
Documentation: Comment your skinning code to explain the design choices. This is invaluable for both current and future developers.
-
Leverage JavaFX Docs: Utilize JavaFX Documentation to stay updated on the best practices and framework changes.
-
Testing: Always test your custom skins in various scenarios and under multiple resolutions to ensure consistent behavior and appearance.
Final Thoughts
Troubleshooting skinning issues in JavaFX 8 applications doesn’t have to be overwhelming. By understanding common problems and their solutions, you can create visually appealing and reliable user interfaces. Remember the core concepts of JavaFX skinning and utilize the best practices we've discussed to minimize issues as you develop.
For further reading, you may find these resources helpful:
- JavaFX CSS Reference Guide
- JavaFX Tutorials
With a bit of strategic thinking and debugging, you can achieve a flawless skin for your applications, enhancing the overall user experience. Happy coding!