Mastering Java Frameworks for Dynamic Form Input Styling
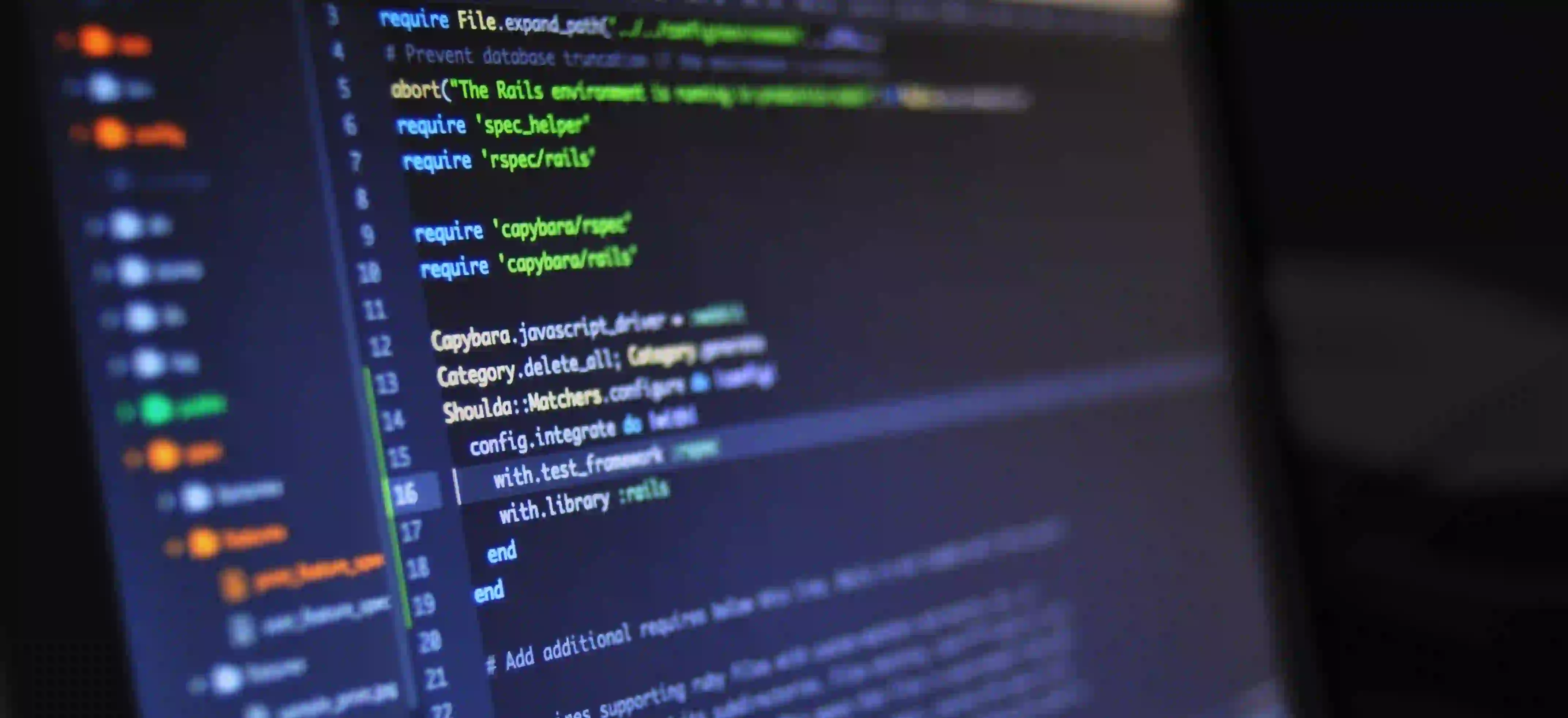
Mastering Java Frameworks for Dynamic Form Input Styling
When it comes to web development, one of the most crucial components is the form. Forms are essential for collecting user input, and as such, their design and functionality significantly impact user experience. In this guide, we’ll explore how Java frameworks can assist in the dynamic styling of form inputs.
Why Dynamic Styling Matters
Dynamic styling refers to changing the appearance of form inputs based on user interactions or other events. This technique enhances user engagement and ensures that the user interface is responsive and appealing. For instance, changing the style of an input field when it is focused or showing error messages can dramatically improve user experience.
Benefits of Using Java Frameworks
Java frameworks like Spring, JavaServer Faces (JSF), and Thymeleaf provide strong foundations for building dynamic web applications. Here are a few reasons why using a Java framework is beneficial:
- Speed and Efficiency: Frameworks usually come with built-in libraries and tools that expedite development processes.
- Maintainability: Code organization is simplified, making it easier to maintain and scale projects.
- Community and Support: Most popular frameworks have extensive documentation and community support.
Java Frameworks in Action
1. Spring MVC Framework
Spring MVC is a foundational web framework under the broader Spring framework umbrella. It promotes a clear separation of concerns, enabling developers to easily manage input forms.
Getting Started with Spring MVC
To begin, you will need to set up a Spring MVC project. Once you have your project structure ready, create a Spring Boot application with the necessary dependencies. Below is a simple controller for handling form submissions.
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
@Controller
@RequestMapping("/form")
public class FormController {
@GetMapping
public String showForm() {
return "form"; // returns the form view
}
@PostMapping
public String submitForm(@RequestParam String input) {
// Process your input here
return "result"; // returns the result view after submission
}
}
Why Use This Code?
- The
@Controller
annotation indicates that this class applies to the MVC pattern, handling requests. @GetMapping
maps HTTP GET requests onto the specified method. Here, it returns the form when accessed.@PostMapping
handles the submission of the form.
Dynamic Styling with Thymeleaf
Thymeleaf, a template engine for Java, seamlessly integrates with Spring MVC for rendering HTML/CSS based views dynamically.
Utilizing Thymeleaf for Styling
You can use Thymeleaf to provide dynamic feedback alongside your input fields. Here’s an example of how to incorporate validation styles directly in your form view:
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Form Input</title>
<link rel="stylesheet" href="/css/styles.css" />
</head>
<body>
<form action="#" th:action="@{/form}" method="post">
<input type="text" name="input"
th:class="${error ? 'error-input' : ''}" />
<div th:if="${error}" class="error-message">Please correct the error</div>
<button type="submit">Submit</button>
</form>
</body>
</html>
Why This Code Matters:
- The
th:class
dynamically adds theerror-input
class based on the$error
variable, styling the input field differently when there is a validation issue. th:if
conditionally displays an error message, enhancing user feedback regarding form submissions.
2. JavaServer Faces (JSF)
JSF is another popular framework designed specifically for Java web applications. It simplifies the development of user interfaces by using reusable UI components.
Building a Dynamic Form in JSF
JSF can auto-update the form input using its AJAX capabilities. Here’s a basic JSF form:
<h:form>
<h:inputText id="inputField" value="#{myBean.inputData}"
styleClass="#{myBean.hasError ? 'error-input' : ''}" />
<h:commandButton value="Submit" action="#{myBean.submit}" onclick="validate();" />
<h:outputText value="#{myBean.errorMessage}" rendered="#{myBean.hasError}"
styleClass="error-message" />
</h:form>
Why Choose JSF?
h:inputText
andh:commandButton
create an intuitive user interface while handling events intrinsically.- The
styleClass
attribute allows dynamic styling based on your managed bean’s properties, providing a smooth UX.
Combining Frameworks for Enhanced Functionality
You can integrate frameworks like Spring and Thymeleaf or JSF to accomplish a more complex application architecture. Employing multiple frameworks provides robust functionalities, ensuring that your dynamic form input styling is both effective and user-friendly.
Case Study: Overcoming Styling Challenges for Form Inputs
In reference to the article Overcoming Styling Challenges for Form Inputs, the discussion there emphasizes the significance of using effective techniques for designing forms. You can find it at infinitejs.com/posts/overcoming-styling-challenges-form-inputs. The challenges addressed align with the techniques mentioned in this article, providing a comprehensive understanding of dynamic form input styling.
A Final Look
Mastering Java frameworks like Spring and JSF allows developers to create dynamic and visually engaging form inputs with ease. By leveraging libraries like Thymeleaf, developers can enhance their forms by dynamically responding to user interactions while maintaining clean and maintainable code.
The emphasis on styling can elevate user experience significantly. As we have explored, dynamic styles not only improve aesthetics but also enhance usability through real-time feedback. Armed with this knowledge, you are well on your way to creating intuitive and user-centered forms in your applications. Happy coding!