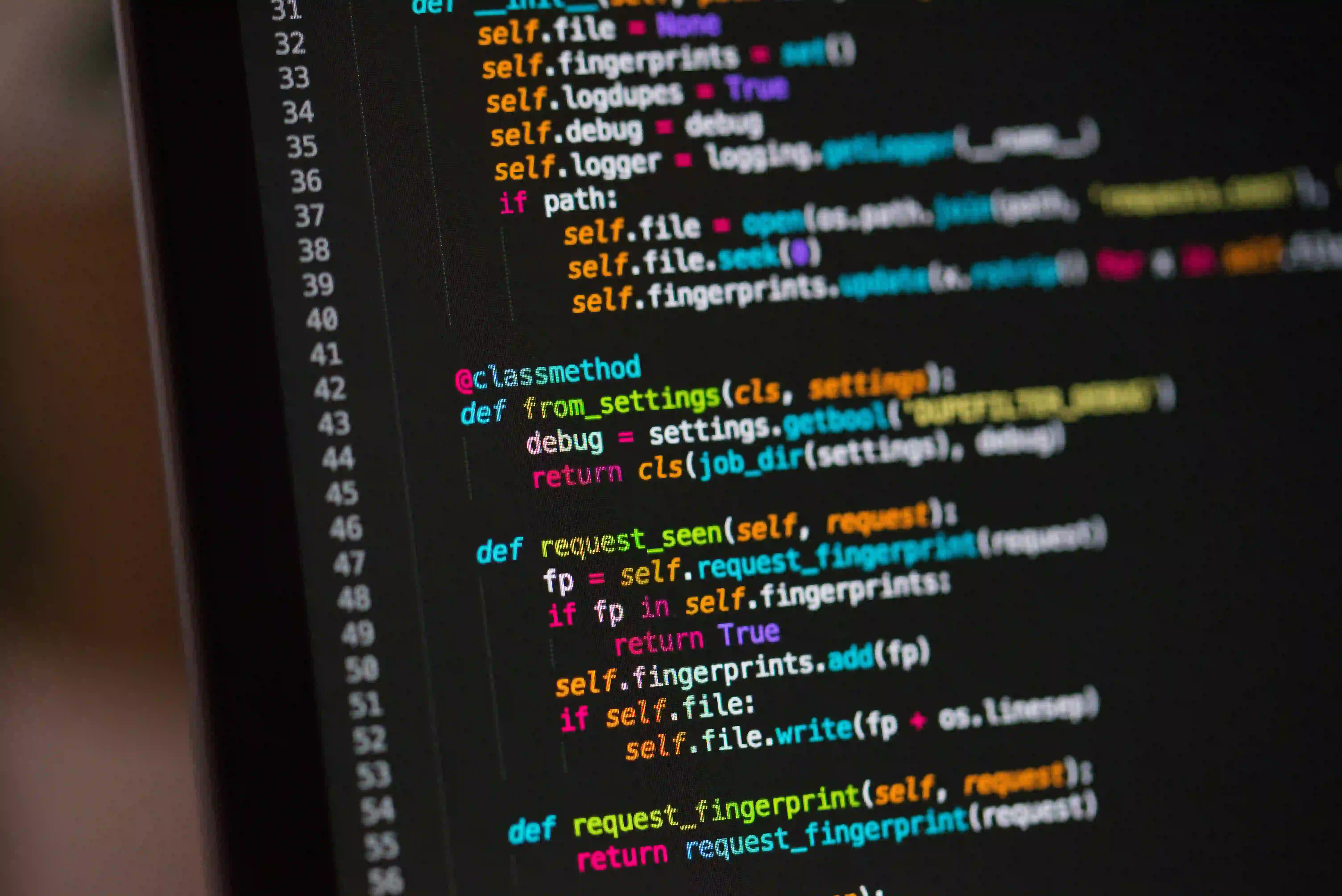
Mastering Java: Tackling Form Input Styling Issues
When developing a web application, form inputs play a crucial role in user interaction. Whether it's a simple contact form or a complex registration page, the appearance and behavior of form inputs can significantly affect user experience. In Java, specifically when using frameworks such as Spring or Java Server Faces (JSF), handling form inputs effectively is essential. This blog post delves into common challenges associated with styling form inputs and provides solutions to master these challenges effectively.
Understanding Form Inputs
Form inputs are HTML elements that allow users to enter data. They can include text fields, checkboxes, radio buttons, dropdown lists, and more. Each input type serves a unique purpose, yet they all share common styling challenges. These challenges frequently arise from browser inconsistencies, different rendering engines, and the need for responsiveness.
The Importance of Styling
The visual presentation of form inputs can influence user behavior. A well-styled form can enhance usability, increase conversion rates, and provide users with a pleasant experience. Conversely, poorly styled inputs may lead to frustration and deter users from completing a form.
To understand how Java can assist in overcoming these challenges, let’s explore some common issues associated with styling form inputs and how we can resolve them.
1. Browser Inconsistencies
Different browsers render form inputs differently. A checkbox in Chrome may appear different in Firefox or Safari. This inconsistency can confuse users and degrade the overall user experience.
Solution: Use CSS Reset
A CSS reset helps standardize the appearance of HTML elements across various browsers. Below is an example of a basic CSS reset:
/* Resetting margins and paddings */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
/* Setting a common font */
body {
font-family: Arial, sans-serif;
}
/* Styling input fields */
input, select, textarea {
border: 1px solid #ccc;
border-radius: 4px;
padding: 10px;
font-size: 16px;
}
Why This Works
Using a CSS reset not only eliminates inconsistencies but also provides a uniform base for building styles upon. By standardizing paddings, margins, and borders, we can create a cohesive design that behaves similarly across all browsers.
2. Responsive Design
Modern web applications need to be responsive, adapting to various screen sizes from desktops to smartphones. This adaptability can create significant challenges with form inputs, mainly if the inputs are not styled correctly to be fluid.
Solution: Fluid Styling Techniques
Utilize percentages or viewport width (vw) units to ensure form elements are fluid. Here's how you can achieve this:
/* Making form inputs responsive */
input[type="text"],
select {
width: 100%; /* Full width on smaller screens */
max-width: 400px; /* Limit width on larger screens */
}
Why This Works
By using a percentage width and a maximum width, form inputs resize fluidly while staying constrained on larger screens. This approach ensures that forms remain user-friendly across all devices, which is essential for a good user experience.
3. Customizing Appearance
Out of the box, form elements may not align with your application’s branding guidelines. Customizing the appearance of inputs can be a challenge, especially with certain input types like checkboxes and radio buttons.
Solution: Custom Styling with Selectors
You can create a custom appearance by leveraging CSS pseudo-elements and custom styling techniques. Here's an example of transforming the appearance of checkboxes:
/* Hiding the checkbox */
input[type="checkbox"] {
display: none;
}
/* Custom checkbox styling */
.checkbox-label {
position: relative;
padding-left: 25px;
cursor: pointer;
}
.checkbox-label:before {
content: "";
position: absolute;
left: 0;
top: 0;
width: 20px;
height: 20px;
border: 1px solid #ccc;
background: white;
}
input[type="checkbox"]:checked + .checkbox-label:before {
background: #4CAF50; /* Change background on check */
}
Why This Works
Hiding the default checkbox and creating a custom appearance allows for a more visually appealing form. The use of pseudo-elements enables us to style components without disrupting the functionality. With this approach, the checkbox integrates seamlessly into any design aesthetic.
4. Accessibility Considerations
When styling form inputs, accessibility must be a priority. Poorly styled inputs or lack of proper labels can create challenges for users relying on assistive technologies.
Solution: ARIA Attributes and Labels
Integrate ARIA (Accessible Rich Internet Applications) attributes and ensure all form elements have associated labels:
<form>
<label for="name">Name:</label>
<input type="text" id="name" aria-required="true" required>
<label for="email">Email:</label>
<input type="email" id="email" aria-required="true" required>
<input type="submit" value="Submit">
</form>
Why This Works
Using labels enhances accessibility. When properly associated with form elements, they allow screen readers to provide context to users. Implementing ARIA attributes promotes a better understanding of the form's requirements, enhancing the user experience.
5. Form Validation Feedback
Providing users feedback while filling out forms is critical to ensuring successful submission. Too often, lack of feedback leads users to submit forms prematurely or abandon them altogether.
Solution: Using Java for Validation
In a Java application, validation can be handled elegantly using JavaBeans or similar structures. Below is an example using Java Spring:
import javax.validation.constraints.Email;
import javax.validation.constraints.NotEmpty;
public class UserForm {
@NotEmpty(message = "Name is required")
private String name;
@Email(message = "Invalid email format")
private String email;
// Getters and Setters
}
Why This Works
By defining validation rules as part of your data model, users receive immediate feedback on errors. The application can provide real-time validation messages, allowing users to correct mistakes as they occur.
In Conclusion, Here is What Matters
Styling form inputs effectively is integral to creating user-friendly web applications in Java. By addressing challenges such as browser inconsistencies, responsiveness, custom appearance, accessibility, and validation feedback, developers can enhance their forms and ultimately improving the user experience.
To dive deeper into designing form inputs and tackling various styling challenges, consider reading the article "Overcoming Styling Challenges for Form Inputs" at infinitejs.com/posts/overcoming-styling-challenges-form-inputs.
Mastering Java and understanding the nuances of form input styling is imperative for every web developer. With the strategies outlined in this article, you can elevate your applications to new heights, ensuring that your users enjoy a seamless and accessible experience from start to finish. Happy coding!