Fixing Faded Nav-Link Colors in Java Web Apps
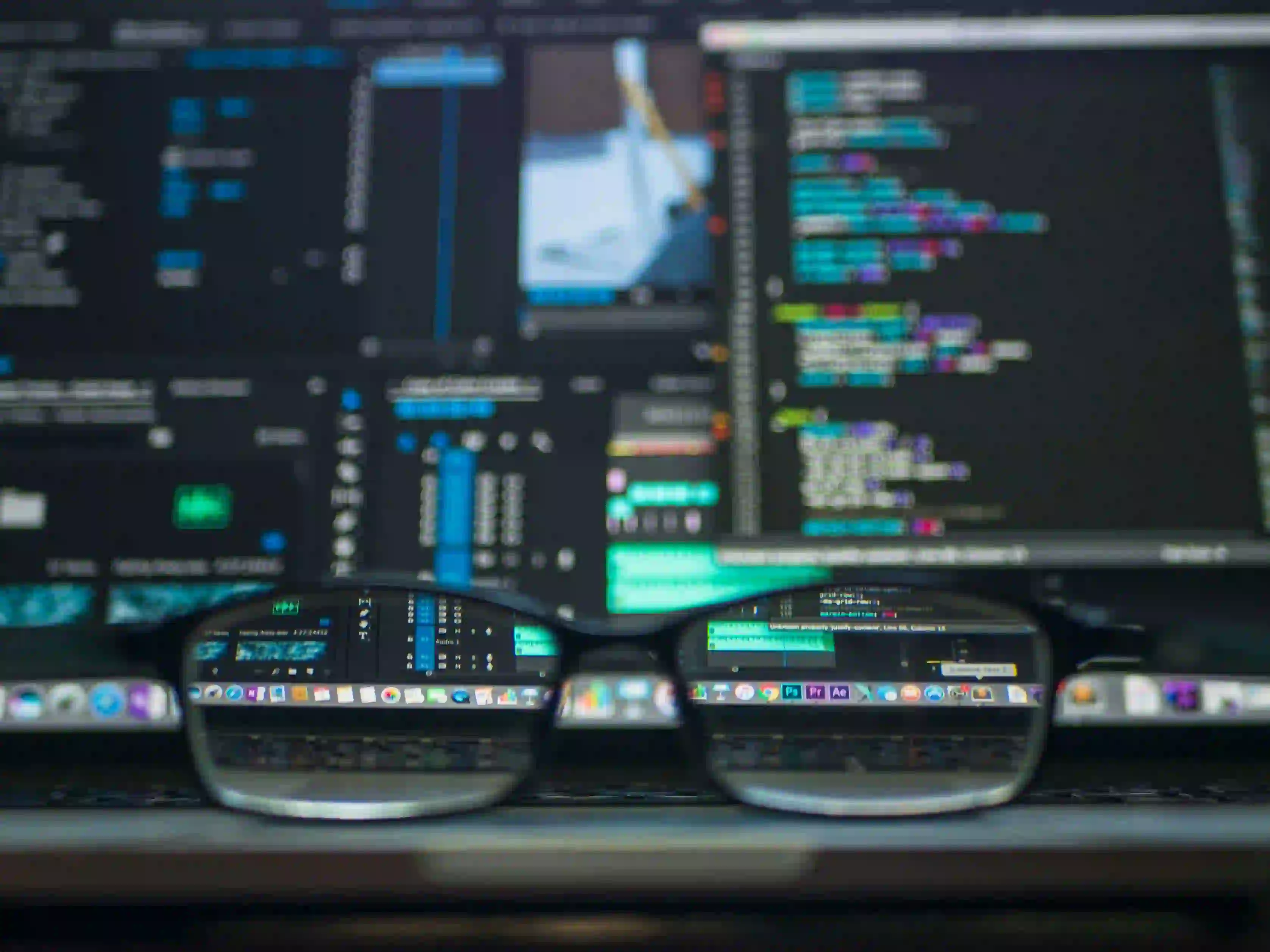
Fixing Faded Nav-Link Colors in Java Web Apps
When developing web applications using Java, you often find yourself not just coding functionality but also crafting a user experience. One common challenge that developers face is ensuring that navigation link colors are vibrant and maintain a consistent appearance across various web browsers and devices. If you've ever encountered faded nav-link colors in your website’s style, you know how distracting it can be.
In this blog post, we'll explore how to address faded nav-link colors in Java web applications and provide you with practical code snippets, tips, and tricks to enhance your application’s UI.
Understanding the Problem:Why Are My Nav-Link Colors Faded?
Faded nav-link colors can stem from various factors. Poor CSS settings, browser compatibility issues, or even HTML structure can contribute to lackluster appearance. Often, developers overlook subtle CSS properties that drastically alter color perception.
It’s also worth mentioning that if you are looking for specific solutions to CSS-related issues, you could check out How to Solve Faded Nav-Link Colors in Your CSS. That article provides an excellent foundation for troubleshooting your CSS styles, and we’ll touch on some principles here as well.
The Basic HTML Structure
Before diving into the Java part of your web application, let’s start with a basic HTML structure that includes a navigation menu:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Java Web App</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<nav>
<ul>
<li><a href="#home" class="nav-link">Home</a></li>
<li><a href="#about" class="nav-link">About</a></li>
<li><a href="#services" class="nav-link">Services</a></li>
<li><a href="#contact" class="nav-link">Contact</a></li>
</ul>
</nav>
<script src="scripts.js"></script>
</body>
</html>
Commentary
Here, we have a basic structure – a navigation bar containing links to different sections of our site. To make it visually appealing and functional, it’s imperative to style these links appropriately in our CSS.
Suggested CSS Styles for Nav-Links
Next, let's move on to how we can address the faded colors using CSS.
nav {
background-color: #333;
}
nav ul {
list-style: none;
}
nav li {
display: inline;
}
nav a {
color: #fff; /* Initial color */
text-decoration: none; /* Remove underline */
padding: 15px 20px;
display: inline-block;
transition: color 0.3s ease; /* Smooth transition for color change */
}
nav a:hover {
color: #ff6347; /* Color on hover */
}
nav a.active {
color: #ff6347; /* Active link color */
}
Commentary
Here's a breakdown of why each line is crucial:
color: #fff;
: This sets the default color of our nav-links to white, which stands out against a dark background, preventing fading.text-decoration: none;
: It simply removes the underline from links, lending a more modern feel.transition: color 0.3s ease;
: Enhances user experience by smoothing out the color changes, ensuring links don’t abruptly change color.- Active Link Color: The distinct color for active links makes it clear to users where they are on the website.
Setting Up Your Java Web Framework
Now, if you are using a Java web framework like Spring, ensure that your view templates correctly link to your CSS:
Sample Code for a Spring Boot Application
Here is an example of how to set up a controller that maps to the home page:
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class HomeController {
@RequestMapping("/")
public String home() {
return "home"; // 'home.html' in `src/main/resources/templates`
}
}
Commentary
In this simple Spring Boot application, we’ve created a controller that maps to the root URL and returns the 'home' view. The view is expected to include the navigation bar defined in the HTML structure above.
Handling Hover States and Accessibility
In web design, usability and accessibility are paramount. It’s essential to ensure that your nav-links do not only look good but are also easily accessible.
-
Keyboard Navigation: Ensure that your nav-links can be accessed via keyboard, enabling use by those who cannot use a mouse.
-
Focus States: It’s beneficial to add focus states to your links:
nav a:focus {
outline: 2px solid #ff6347; /* Focus outline for accessibility */
}
Commentary
Adding this focus state allows users navigating via keyboard to visualize which link is currently selected. This addition not only enhances the user experience but also meets accessibility standards.
JavaScript for Dynamic Interactions (Optional)
For complex interactions—like highlighting the active nav-link based on user actions—JavaScript can elevate your UI.
const navLinks = document.querySelectorAll('.nav-link');
navLinks.forEach(link => {
link.addEventListener('click', function() {
navLinks.forEach(l => l.classList.remove('active'));
this.classList.add('active'); // Mark the clicked link as active
});
});
Commentary
This snippet dynamically adds the 'active' class to the link that the user clicks, enhancing visual feedback and usability as the UI adheres to the user's actions.
In Conclusion, Here is What Matters
Maintaining vibrant nav-link colors while ensuring they are effective and functional in a Java web application can be a nuanced task. By carefully adjusting your HTML, CSS, and, if needed, JavaScript, you can create a user-friendly navigation experience.
Navigational links are a critical aspect of usability. Therefore, it is crucial to frequently revisit your design practices and code base to ensure they adhere to best practices in modern web design.
If you're facing more specific issues related to CSS or UI elements, don't forget to explore How to Solve Faded Nav-Link Colors in Your CSS for deeper insights.
Happy coding! Your users will appreciate the effort you've put into refining their experience.