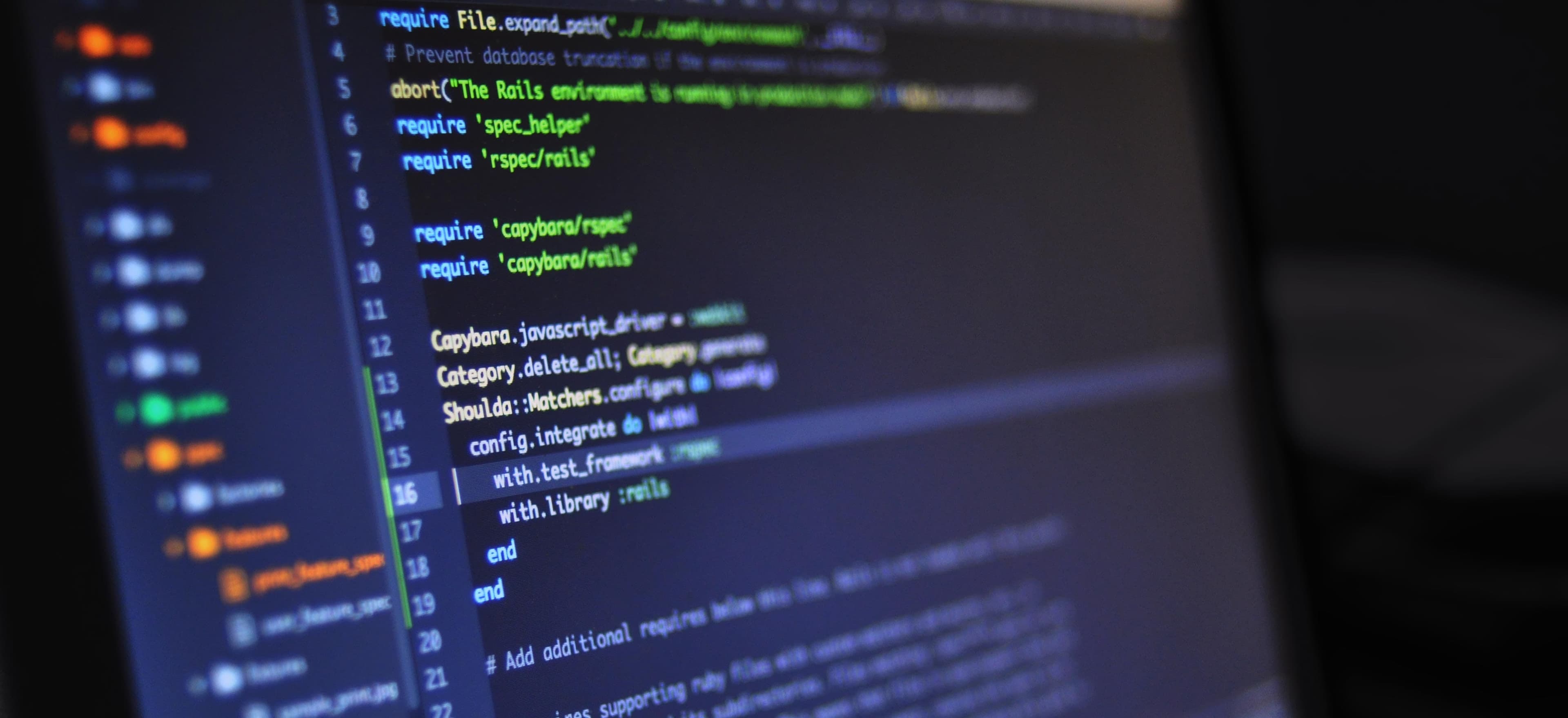
- Published on
Mastering Java: Hiding Elements for Enhanced Accessibility
In the world of web development, accessibility should always take center stage. A vital aspect of this consideration is the ability to manage the visibility of elements on your web pages effectively. Whether you're initiating animations, creating a user-friendly interface, or tailoring content for specific user needs, knowing how to hide elements can play an essential role.
In this article, we will explore strategies for handling the visibility of elements in Java, whether you're using Java for web-based applications or desktop applications. We will also touch upon how these strategies relate to curated accessibility principles. Additionally, if you're interested in reducing the visual clutter in animated elements, check out the article titled "Boost Accessibility: Hide GIF Play Buttons With Ease".
Why Accessibility Matters
Accessibility enables users with disabilities to interact fully with websites and applications. This approach ranges from using descriptive alt text for images to ensuring that all interactive elements are keyboard navigable. Hiding non-essential elements can enhance focus and usability for everyone, particularly for users who depend on assistive technologies.
Hiding Elements in Java: An Overview
In Java, the process of hiding elements typically revolves around manipulating the user interface components. We will look at examples relevant to both Java Swing and JavaFX, the two predominant libraries for building user interfaces.
Hiding Elements in Java Swing
Java Swing has been widely used to create desktop interfaces. Its flexibility allows developers to dynamically change the visibility of components.
Example: Hiding a JButton in Swing
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class HideButtonExample {
public static void main(String[] args) {
JFrame frame = new JFrame("Hide Button Example");
JPanel panel = new JPanel();
JButton button = new JButton("Click to Hide");
// Attach a listener to the button
button.addActionListener(e -> {
// Hide the button when clicked
button.setVisible(false);
});
panel.add(button);
frame.add(panel);
frame.setSize(300, 200);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
}
In this code snippet, we create a simple Swing application with a button. When the button is clicked, it becomes invisible.
Why This Matters
Hiding elements like buttons can declutter interfaces and keep the user's focus where it is needed. For instance, if a task is completed, hiding the 'Complete' button can guide the user to their next steps without overwhelming them.
Hiding Elements in JavaFX
JavaFX extends the capabilities of Swing and provides a more modern approach to UI design.
Example: Hiding a Label in JavaFX
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class HideLabelExample extends Application {
@Override
public void start(Stage primaryStage) {
Label label = new Label("This will be hidden!");
Button button = new Button("Hide the Label");
// Hide the label when button is clicked
button.setOnAction(e -> {
label.setVisible(false);
});
VBox vbox = new VBox(button, label);
Scene scene = new Scene(vbox, 300, 200);
primaryStage.setScene(scene);
primaryStage.setTitle("Hide Label Example");
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Similar to the Swing example, this JavaFX application hides a label when a button is clicked. JavaFX allows for more complex layouts and effects, making it a popular choice for modern applications.
Why This Matters
By controlling visibility, you can create more accessible applications. For instance, if a user achieves a milestone, they may not need the previous instructions cluttering their view.
Advanced Hiding Techniques
While hiding elements is straightforward, consider utilizing CSS for web applications handled through Java. Using libraries like Spring MVC or JSF, you can incorporate CSS to effectively manage visibility.
Example: Using CSS with Java Web Applications
Your project might be using JSP or Thymeleaf with CSS properties. Here’s an example of using CSS to control visibility:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Hide Elements with CSS</title>
<style>
.hidden {
display: none;
}
</style>
</head>
<body>
<button onclick="hideElement()">Hide Text</button>
<p id="textToHide">This text will be hidden.</p>
<script>
function hideElement() {
document.getElementById("textToHide").classList.add("hidden");
}
</script>
</body>
</html>
In this HTML example, clicking the button hides the paragraph element by adding a CSS class. This method is beneficial when working with web development, as it separates style from functionality.
Why This Matters
Utilizing CSS for accessibility helps maintain clean code and separates concerns. This approach improves maintainability and allows developers to adjust styles without modifying the underlying Java logic.
Best Practices for Hiding Elements
- Provide Context: Always ensure that hidden elements serve a purpose and are relevant to the user experience.
- Keyboard Accessibility: Ensure users can navigate all hidden elements using a keyboard, if applicable.
- Use ARIA Attributes: If working on web applications, consider using ARIA (Accessible Rich Internet Applications) attributes like
aria-hidden
to inform assistive technologies about hidden elements. - Test for Accessibility: Use tools to audit your application for accessibility shortcomings regularly. This will help you identify areas that may require more visibility or less clutter.
Lessons Learned
Mastering the art of hiding elements in Java applications not only enhances usability but also supports accessibility goals. Effective management of UI components can lead to a cleaner, more focused user experience. Whether you utilize Swing, JavaFX, or integrate CSS in your web applications, the principles remain the same.
For further context on enhancing accessibility specifically regarding GIFs, feel free to read "Boost Accessibility: Hide GIF Play Buttons With Ease".
By implementing these techniques, you contribute to a more inclusive digital environment. Remember, the goal is to create beautiful and functional applications that everyone can enjoy.