Java Strategies for Managing Financial Data Efficiently
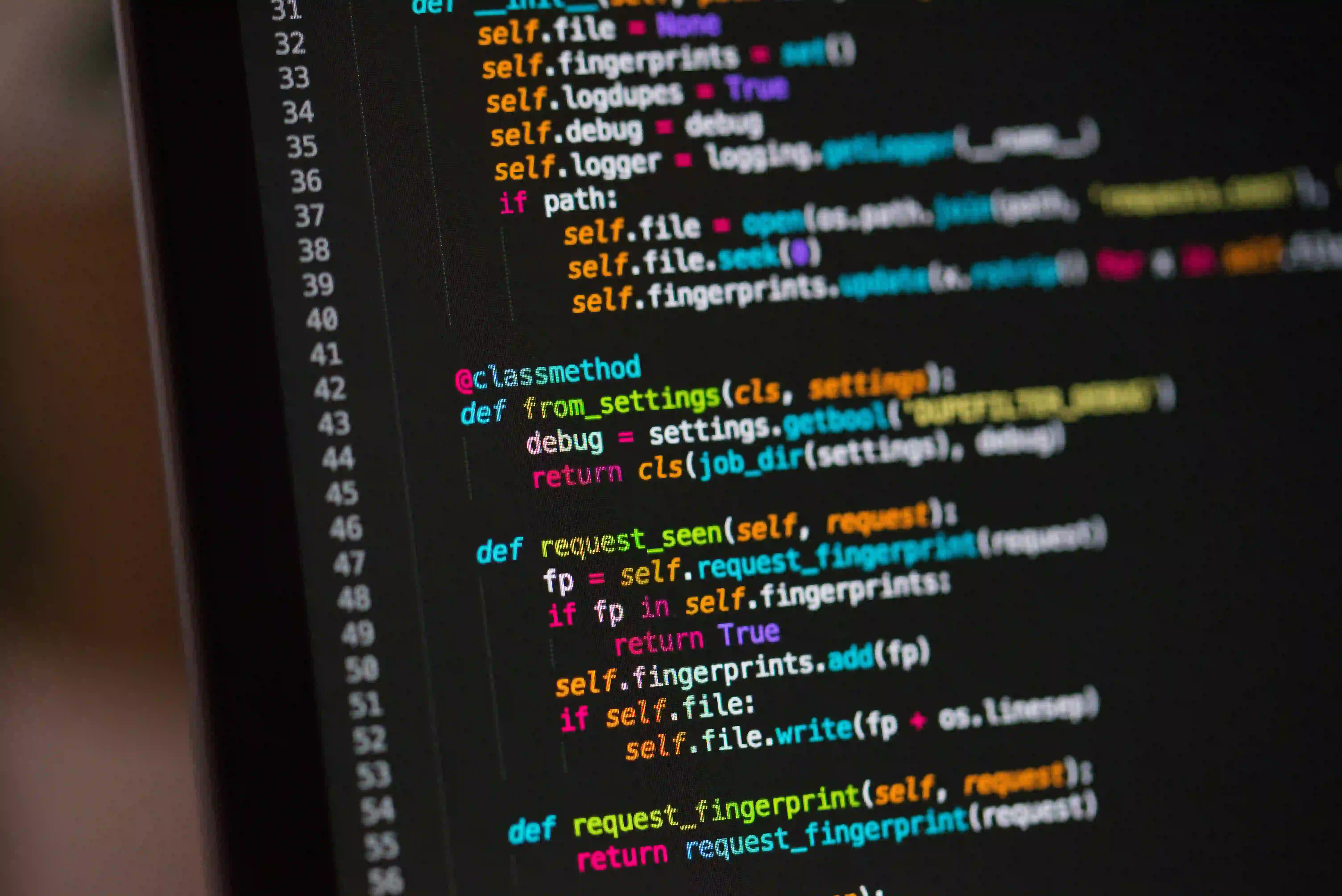
Java Strategies for Managing Financial Data Efficiently
In today's world, efficient handling of financial data is crucial. With exponential growth in data amounts that organizations deal with, leveraging programming for financial analyses has become necessary. Java, a robust and versatile programming language, offers various strategies to manage and process financial data effectively.
Why Use Java for Financial Data Management?
Java is favored in the finance industry due to its portability, security features, and rich ecosystem. Whether you're dealing with transaction records, account balances, or investment portfolios, Java provides the necessary tools to handle complex calculations and data manipulations. Its object-oriented nature allows developers to create reusable code that can be easily extended.
In this article, we will explore various strategies for managing financial data using Java, alongside code snippets to illustrate each concept.
Data Structures: Choosing the Right One
Collections Framework
Java's Collections Framework provides a powerful and flexible way to manage data. For financial applications, you might need to store lists of transactions, account details, or historical data. Choosing the right data structure is crucial for performance and efficiency.
Here's how to use an ArrayList
to store transaction data:
import java.util.ArrayList;
public class Transaction {
private double amount;
private String type;
public Transaction(double amount, String type) {
this.amount = amount;
this.type = type;
}
public double getAmount() {
return amount;
}
public String getType() {
return type;
}
}
public class TransactionManager {
private ArrayList<Transaction> transactions;
public TransactionManager() {
transactions = new ArrayList<>();
}
public void addTransaction(double amount, String type) {
transactions.add(new Transaction(amount, type));
}
public double getTotal() {
double total = 0.0;
for (Transaction transaction : transactions) {
total += transaction.getAmount();
}
return total;
}
}
Why Use ArrayLists?
- Dynamic Size: ArrayLists can grow or shrink dynamically, unlike arrays with fixed size. This is especially useful when the number of transactions can change over time.
- Quick Access: Elements can be accessed quickly using an index, which can speed up retrieval operations.
Data Persistence: Saving Financial Data
Financial applications often need to persist data between sessions. Here, we can use Java Database Connectivity (JDBC) to connect to relational databases.
Basic JDBC Example
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
public class DatabaseManager {
private String url = "jdbc:mysql://localhost:3306/finance_db";
private String user = "username";
private String password = "password";
private Connection conn;
public DatabaseManager() throws Exception {
conn = DriverManager.getConnection(url, user, password);
}
public void saveTransaction(Transaction transaction) throws Exception {
String sql = "INSERT INTO transactions (amount, type) VALUES (?, ?)";
PreparedStatement statement = conn.prepareStatement(sql);
statement.setDouble(1, transaction.getAmount());
statement.setString(2, transaction.getType());
statement.executeUpdate();
}
public void fetchTransactions() throws Exception {
String sql = "SELECT * FROM transactions";
PreparedStatement statement = conn.prepareStatement(sql);
ResultSet resultSet = statement.executeQuery();
while (resultSet.next()) {
double amount = resultSet.getDouble("amount");
String type = resultSet.getString("type");
System.out.println("Transaction: " + type + ", Amount: " + amount);
}
}
}
Why Use JDBC?
- Structured Data: Financial data can often be large and complex. A database allows for structured storage and management.
- Persistence: Data remains available between execution sessions, which is crucial for any financial application.
Handling Concurrency: Multi-Threaded Access
In financial applications, multiple threads might attempt to access or modify data at the same time. Proper management of concurrency is essential to prevent inconsistencies.
Using Synchronization
public class SynchronizedTransactionManager {
private final List<Transaction> transactions = new ArrayList<>();
public synchronized void addTransaction(double amount, String type) {
transactions.add(new Transaction(amount, type));
}
public synchronized double getTotal() {
double total = 0.0;
for (Transaction transaction : transactions) {
total += transaction.getAmount();
}
return total;
}
}
Why Synchronize Access?
- Data Integrity: By synchronizing the methods, we ensure that only one thread can modify the transactions list at a time.
- Avoid Conflicts: This reduces the risk of unexpected behavior during multi-threaded operations, protecting the financial integrity of the application.
Data Analysis: Using Java Streams
For financial analysis, Java Streams offer a powerful way to process data efficiently.
Example of Stream Processing
import java.util.ArrayList;
import java.util.List;
public class FinancialAnalysis {
private List<Transaction> transactions;
public FinancialAnalysis(List<Transaction> transactions) {
this.transactions = transactions;
}
public double calculateAverageTransaction() {
return transactions.stream()
.mapToDouble(Transaction::getAmount)
.average()
.orElse(0.0);
}
}
Why Use Streams?
- Fluent API: The Streams API provides a concise and readable way to perform complex operations on collections.
- Parallel Processing: Streams can leverage multi-core architecture very easily, speeding up large data processing tasks.
Closing Remarks
Managing financial data efficiently using Java involves understanding the right data structures, ensuring data persistence, handling thread safety, and leveraging advanced data processing features. By applying the strategies outlined in this article, you can create robust financial applications that meet today's demands.
For those interested in maximizing their financial resources effectively, consider also reading the article "Maximizing Your Cash Reserves: A Prepper's Guide" at youvswild.com/blog/maximize-cash-reserves-prepper-guide. It provides valuable insights that could complement your technical knowledge with practical financial strategies.
By implementing these approaches, you can take your financial data management in Java to the next level, ensuring both accuracy and efficiency in your applications.