Enhancing Java Applications: Improve Accessibility with Ease
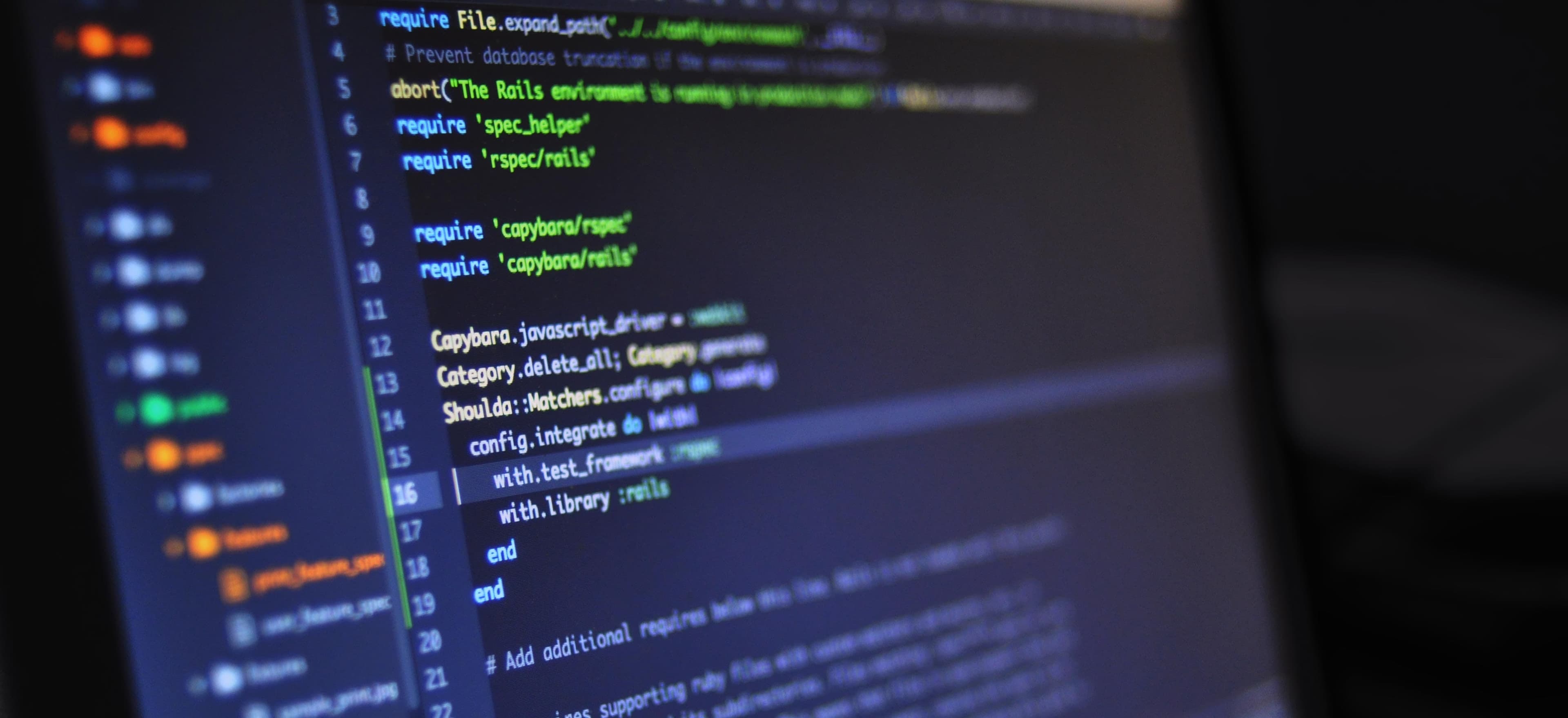
- Published on
Enhancing Java Applications: Improve Accessibility with Ease
In today's world, creating accessible applications is no longer just an option; it is a necessity. With a growing emphasis on inclusivity, developers must ensure that their applications cater to users of all abilities. This blog post focuses on enhancing accessibility within Java applications and provides practical recommendations backed by examples.
What is Accessibility in Software Development?
Accessibility in software development refers to the design of products, devices, services, or environments that are usable by people with disabilities. It emanates from the concept of universal design, which seeks to improve usability for everyone, regardless of their abilities or disabilities. Accessible applications empower users by considering their diverse needs, helping them engage more effectively with the technology.
Why is Accessibility Important?
-
User Inclusivity: By developing accessible applications, you open your product to a broader audience, potentially increasing your user base.
-
Legal Compliance: Many countries have laws mandating digital accessibility, such as the ADA in the United States. Non-compliance can result in legal repercussions.
-
Enhanced User Experience: Accessible applications tend to be more user-friendly, providing a better experience for all users, not just those with disabilities.
-
Brand Loyalty: Companies that actively promote accessibility demonstrate social responsibility, which can enhance brand loyalty.
Key Areas to Focus On
- Semantic Markup
- Keyboard Navigation
- Color Contrast
- Use of ARIA Roles
- Dynamic Content Handling
Let’s delve deeper into these areas, illustrating each with Java-based examples.
1. Semantic Markup
In web development, semantic HTML informs assistive technologies about the structure and meaning of content. For Java applications, ensure that your user interface (UI) components are structured properly.
Example: Using Layout Managers
Instead of hardcoding positions, using layout managers ensures that components resize and reposition based on the user's environment.
import javax.swing.*;
import java.awt.*;
public class AccessibleUI {
public static void main(String[] args) {
JFrame frame = new JFrame("Accessible UI Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// Using GridLayout for dynamic arrangements
frame.setLayout(new GridLayout(2, 2));
JButton button1 = new JButton("Button 1");
JButton button2 = new JButton("Button 2");
frame.add(button1);
frame.add(button2);
frame.setSize(400, 200);
frame.setVisible(true);
}
}
Why?: The GridLayout
manager ensures that buttons adjust their size based on the frame, accommodating various display settings.
2. Keyboard Navigation
Keyboard navigation is vital for users who cannot use a mouse. Ensuring that all functionality is accessible via keyboard shortcuts is a best practice.
Example: Implementing Key Bindings
import javax.swing.*;
import java.awt.event.*;
public class KeyBindingExample {
public static void main(String[] args) {
JFrame frame = new JFrame("Key Binding Example");
JTextArea textArea = new JTextArea();
// Key binding for focusing the text area
textArea.getInputMap().put(KeyStroke.getKeyStroke("F"), "focusTextArea");
textArea.getActionMap().put("focusTextArea", new AbstractAction() {
@Override
public void actionPerformed(ActionEvent e) {
textArea.requestFocus();
}
});
frame.add(textArea);
frame.setSize(400, 200);
frame.setVisible(true);
}
}
Why?: This example allows users to navigate to the text area using the "F" key, enhancing accessibility.
3. Color Contrast
Color contrast is fundamental for visually impaired users. Java Swing provides methods to set foreground and background colors, but they must meet accessibility standards (AA or AAA as defined by WCAG).
Example: Setting Colors with High Contrast
import javax.swing.*;
import java.awt.*;
public class ColorContrastExample {
public static void main(String[] args) {
JFrame frame = new JFrame("Color Contrast Example");
JButton button = new JButton("Click Me");
button.setBackground(Color.BLACK);
button.setForeground(Color.WHITE); // High contrast colors
frame.add(button);
frame.setSize(200, 100);
frame.setVisible(true);
}
}
Why?: Here, black and white provide a high-contrast interaction, which is easier to read for those with visual impairments.
4. Use of ARIA Roles
Accessible Rich Internet Applications (ARIA) roles help screen readers understand how to interpret elements in your application.
Example: Setting ARIA Roles in JavaFX
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class ARIAExample extends Application {
@Override
public void start(Stage primaryStage) {
Button button = new Button("Accessible Button");
button.setAccessibleRole(javafx.scene.accessibility.AccessibleRole.BUTTON); // Setting ARIA role
VBox vbox = new VBox(button);
Scene scene = new Scene(vbox, 300, 250);
primaryStage.setScene(scene);
primaryStage.setTitle("ARIA Example");
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Why?: Adding ARIA roles communicates the role of the button to assistive technologies, making the application more accessible.
5. Dynamic Content Handling
When content updates dynamically, it's essential to inform users of these changes, especially for screen reader users.
Example: Updating Content in JavaFX
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class DynamicContentExample extends Application {
@Override
public void start(Stage primaryStage) {
Label label = new Label("Initial Message");
Button button = new Button("Update Message");
button.setOnAction(e -> {
label.setText("Message Updated!");
label.setAccessibleText("Message Updated!"); // Informing assistive technology
});
VBox vbox = new VBox(label, button);
Scene scene = new Scene(vbox, 300, 200);
primaryStage.setScene(scene);
primaryStage.setTitle("Dynamic Content Example");
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Why?: Updating the accessible text provides clear information to users who rely on assistive technologies.
A Final Look
Integrating accessibility into your Java applications undoubtedly enhances the user experience, broadens your audience, and fulfills legal requirements. By focusing on semantic markup, keyboard navigation, color contrast, ARIA roles, and dynamic content handling, you can create more inclusive software.
For further reading, consider checking out "Boost Accessibility: Hide GIF Play Buttons With Ease"! This article offers practical insights into improving accessibility in digital content, enhancing the experience further for your users. You can find it here.
Remember, accessibility is not a one-time effort but an ongoing process that improves continuously as you gather feedback and learn more about your users. Take the plunge towards making your Java applications accessible today!
Checkout our other articles