Building Resilient Java Apps: Lessons from Prepping
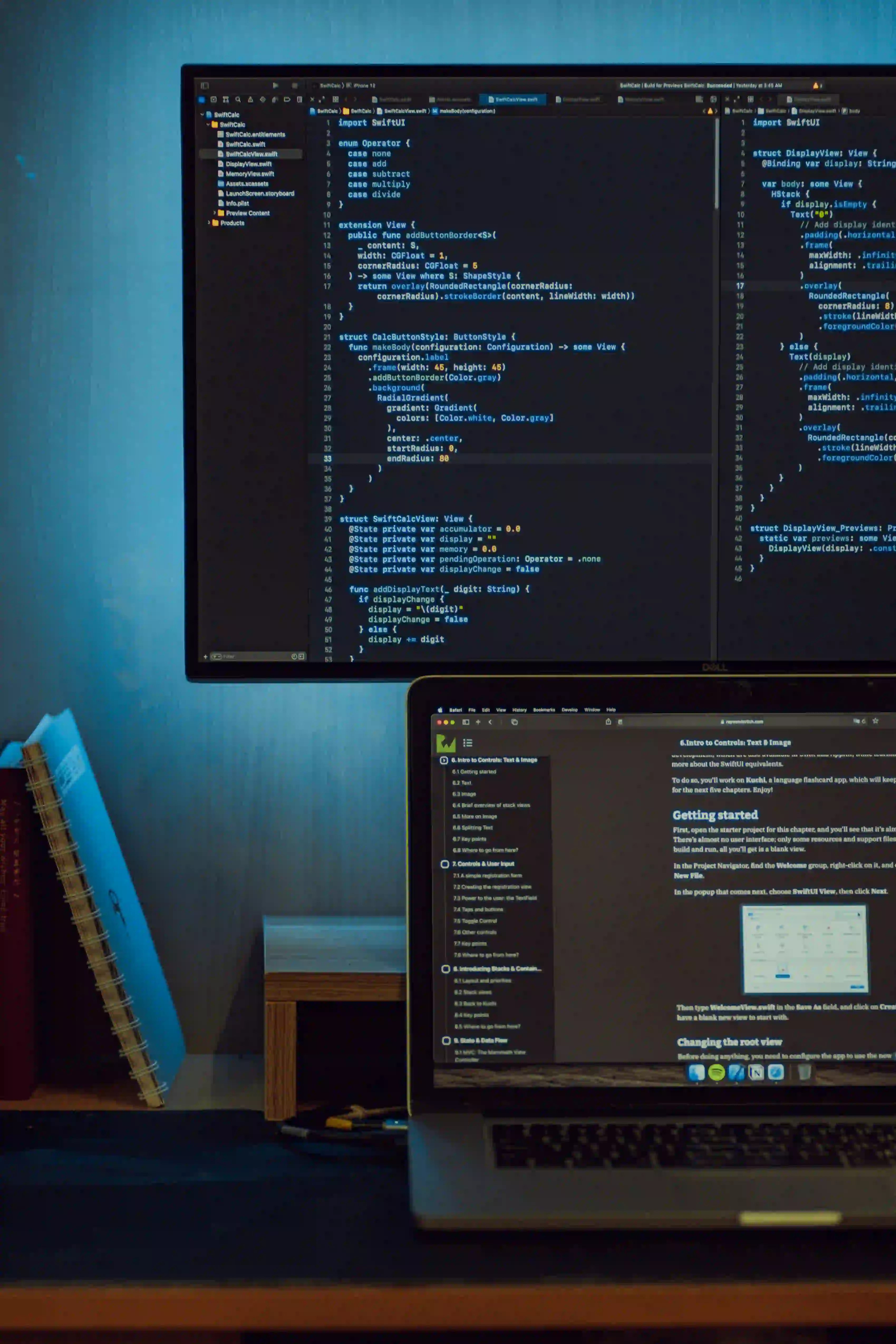
Building Resilient Java Apps: Lessons from Prepping
In an unpredictable world filled with challenges, the philosophy of prepping teaches us to be prepared for the unexpected. Similarly, when developing applications—especially with a robust language like Java—it's crucial to build resiliency into your code. In this blog post, we will explore how lessons from prepping can be applied to create resilient Java applications. Along the way, we will discuss best practices, explore some code snippets, and examine how to make your applications more reliable, much like a well-prepared prepper.
The Importance of Resilience in Java Applications
Resilience in software systems refers to the ability of an application to gracefully handle unforeseen issues, such as high traffic, data corruption, or system failures. A resilient application can assure that essential services remain available and performance is optimized, even under duress.
Consider a simple analogy: just as a prepper stockpiles food, water, and other essentials to weather a crisis, a software developer must implement various strategies to prepare their Java application for unforeseen circumstances.
Key Principles of Resilience
-
Redundancy: Just like having backup supplies, a resilient application should have failover mechanisms. Redundant systems can help maintain availability.
-
Graceful Degradation: If something goes wrong, the application should continue to function in a limited capacity rather than fail completely.
-
Monitoring and Alerts: Preppers keep tabs on their supplies; similarly, developers should monitor their applications to quickly identify issues.
Let's dive deeper into these principles, backed by code examples to illustrate how you can implement resilience in your Java applications.
Implementing Redundancy
One key lesson from prepping is that redundancy is vital. In software, redundancy can manifest in several ways, including load balancers, failover systems, or microservices that maintain separate backups.
Using Load Balancing in Spring Boot
Consider a Spring Boot application serving user requests. You can distribute requests across multiple instances of your application like so:
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class LoadBalancedController {
@GetMapping("/service")
public String serve() {
// This would ideally call various services
return "Service response from app instance!";
}
}
In your application configuration, you can set up a load balancer using Eureka or Ribbon, which immediately creates redundancy and directs requests away from a faulty instance automatically.
Why This Matters
Implementing load balancing keeps your application responsive, ensuring users receive service even during traffic spikes or when one instance is down.
Building Graceful Degradation
Another significant aspect of building resilient systems is to ensure graceful degradation. You want your application to fail gracefully, ensuring that it continues to provide a partial service rather than a total failure.
Example of Graceful Degradation
In cases where the database goes down, you can use caching to serve previous data, like so:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.cache.annotation.Cacheable;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class DataServiceController {
@Autowired
private UserDataService userDataService;
@Cacheable("users")
@GetMapping("/user")
public User getUserData() {
// Simulate a database call
return userDataService.fetchUserFromDatabase();
}
}
Why Use Caching?
Using caching in Java applications helps reduce database load by storing frequently accessed data. In cases of database service unavailability, your application can still provide outdated but usable data to users, thereby maintaining functionality.
Monitoring and Alerts
Similar to how a prepper keeps a watchful eye on supplies, it's crucial for developers to monitor applications in real time for any anomalies. This can prevent small issues from turning into major outages.
Implementing Monitoring
To begin implementing monitoring, you can utilize Micrometer with Spring Boot to track performance metrics. Here's a quick example:
import io.micrometer.core.instrument.MeterRegistry;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class MonitoringController {
private final MeterRegistry meterRegistry;
public MonitoringController(MeterRegistry meterRegistry) {
this.meterRegistry = meterRegistry;
}
@GetMapping("/track")
public String trackPerformance() {
meterRegistry.counter("requests.total").increment(); // Increment total request count
return "Tracking performance!";
}
}
Why Monitoring is Essential
Monitoring gives you insight into how your application is performing in real time. By recording data such as request counts, latency, and error rates, you can quickly react if your application starts to exhibit problematic behavior.
The Closing Argument
Building resilient Java applications is all about preparation, just like in prepping. By employing redundancy, graceful degradation, and monitoring, you can ensure your application remains robust under adverse conditions.
In summary, consider your Java application like a prepper's stash; a well-prepared application can withstand adversity and keep serving its users, just as a well-stocked larder can sustain a family during tough times.
For further reading on preparation strategies, you might find the article "Maximizing Your Cash Reserves: A Prepper's Guide" insightful, highlighting effective ways to prepare both mentally and materially for uncertain times. You can read it at youvswild.com/blog/maximize-cash-reserves-prepper-guide.
Implement these lessons in your next Java project to ensure you are ready for whatever challenges come your way. By doing so, you are not only creating a software solution but also fostering a culture of resilience—something we can all benefit from.