Resolving Java JDBC Issues with MySQL Date and Float Types
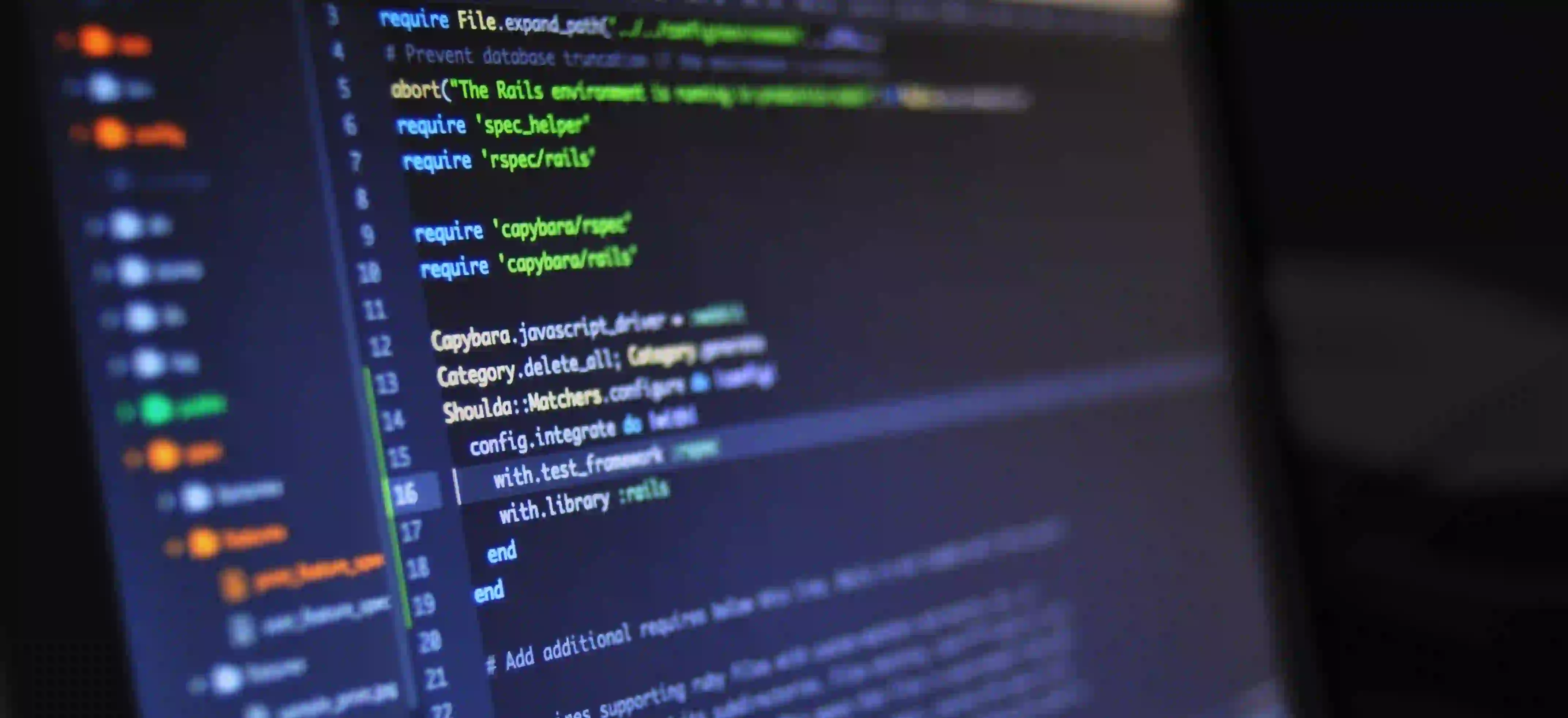
Resolving Java JDBC Issues with MySQL Date and Float Types
When working with Java applications that interact with MySQL databases via JDBC (Java Database Connectivity), developers often encounter data type compatibility issues. These challenges can lead to incorrect data retrieval, insertion errors, or the dreaded runtime exceptions. In this blog post, we'll explore how to effectively handle JDBC issues in Java, particularly focusing on MySQL's Date and Float types.
Understanding Java JDBC
JDBC is a Java-based API that facilitates connecting to databases, executing SQL queries, and retrieving results. It abstracts the complexities of database connections, enabling developers to interact with SQL databases seamlessly. When your application requires data storage and retrieval, understanding JDBC is crucial.
Basic JDBC Connection Example
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class JdbcExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/yourDatabase";
String user = "username";
String password = "password";
try {
Connection connection = DriverManager.getConnection(url, user, password);
System.out.println("Connection established successfully!");
// Remember to close the connection later
} catch (SQLException e) {
e.printStackTrace();
}
}
}
In the above example, we establish a connection to a MySQL database. Handling potential exceptions, as illustrated, is essential for robust application development.
Common Pitfalls with Date and Float Types
While working with MySQL, specific issues surface frequently, especially when dealing with Date and Float types. The root causes often stem from different representations of these data types in Java and MySQL.
Handling MySQL Date in Java
MySQL’s DATE
type corresponds to java.sql.Date
in Java, but converting between these representations can lead to confusion.
Example: Inserting MySQL Date
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.sql.Date;
public class InsertDateExample {
public static void main(String[] args) {
String insertSQL = "INSERT INTO events (event_date) VALUES (?)";
try (Connection connection = DriverManager.getConnection(url, user, password);
PreparedStatement preparedStatement = connection.prepareStatement(insertSQL)) {
// Choosing a specific date
java.util.Date utilDate = new java.util.Date();
Date sqlDate = new Date(utilDate.getTime());
preparedStatement.setDate(1, sqlDate);
preparedStatement.executeUpdate();
System.out.println("Date inserted successfully!");
} catch (SQLException e) {
e.printStackTrace();
}
}
}
In this code, we convert a java.util.Date
to java.sql.Date
before inserting it into the database. This conversion is important because directly passing non-compatible types causes errors.
Timestamp and MySQL
For situations requiring time-specific data, consider using java.sql.Timestamp
.
import java.sql.Timestamp;
// Creating a Timestamp
Timestamp timestamp = new Timestamp(System.currentTimeMillis());
preparedStatement.setTimestamp(1, timestamp);
Float Handling in JDBC
MySQL's FLOAT
type maps to Java's Float
type, but storing and retrieving can yield surprises.
Example: Inserting Float Values
public class InsertFloatExample {
public static void main(String[] args) {
String insertSQL = "INSERT INTO measurements (value) VALUES (?)";
try (Connection connection = DriverManager.getConnection(url, user, password);
PreparedStatement preparedStatement = connection.prepareStatement(insertSQL)) {
float measurementValue = 12.34f; // Make sure to append 'f' to denote float
preparedStatement.setFloat(1, measurementValue);
preparedStatement.executeUpdate();
System.out.println("Float value inserted successfully!");
} catch (SQLException e) {
e.printStackTrace();
}
}
}
The key here is to ensure that you're storing the correct data type. JDBC doesn't automatically convert types and expects precise definitions.
Why the Conversion Matters
Misunderstanding type conversions can lead to two significant issues:
- Data Loss: If the float precision isn't adequately handled, data can become truncated or rounded.
- Insertion and Retrieval Errors: When data types mismatch, you may face SQLExceptions or receive default values instead of expected data.
To further enhance your understanding and management of these data types in PHP, you might want to check out this insightful article titled "Fix PDO BindParam Issues with MySQL Date & Float Types", which draws parallels to JDBC issues.
Best Practices
- Use Corresponding Classes: Always use the specific Java type that correlates to your MySQL type.
- Handle Exceptions: Employ robust try-catch blocks to gracefully handle SQL exceptions.
- Close Connections: Remember to close your database connections to prevent memory leaks.
- Testing: Always test your queries with various inputs to ensure correct functionalities.
Wrapping Up
Navigating through Java JDBC with MySQL can be mildly complex due to type mismatches, especially with Date and Float. Mastering these conversions will greatly enhance data integrity and application stability.
By adhering to best practices in JDBC handling and staying aware of potential pitfalls, you can significantly reduce frustration and enhance the reliability of your Java applications. For further reading on database interactions, I encourage exploring other resources and articles in the technology space.
In conclusion, dealing with nuances in types not only strengthens your application's robustness but also improves the overall development experience. Happy coding!