Spice Up Your Java: Integrating Clove-Infused Logic
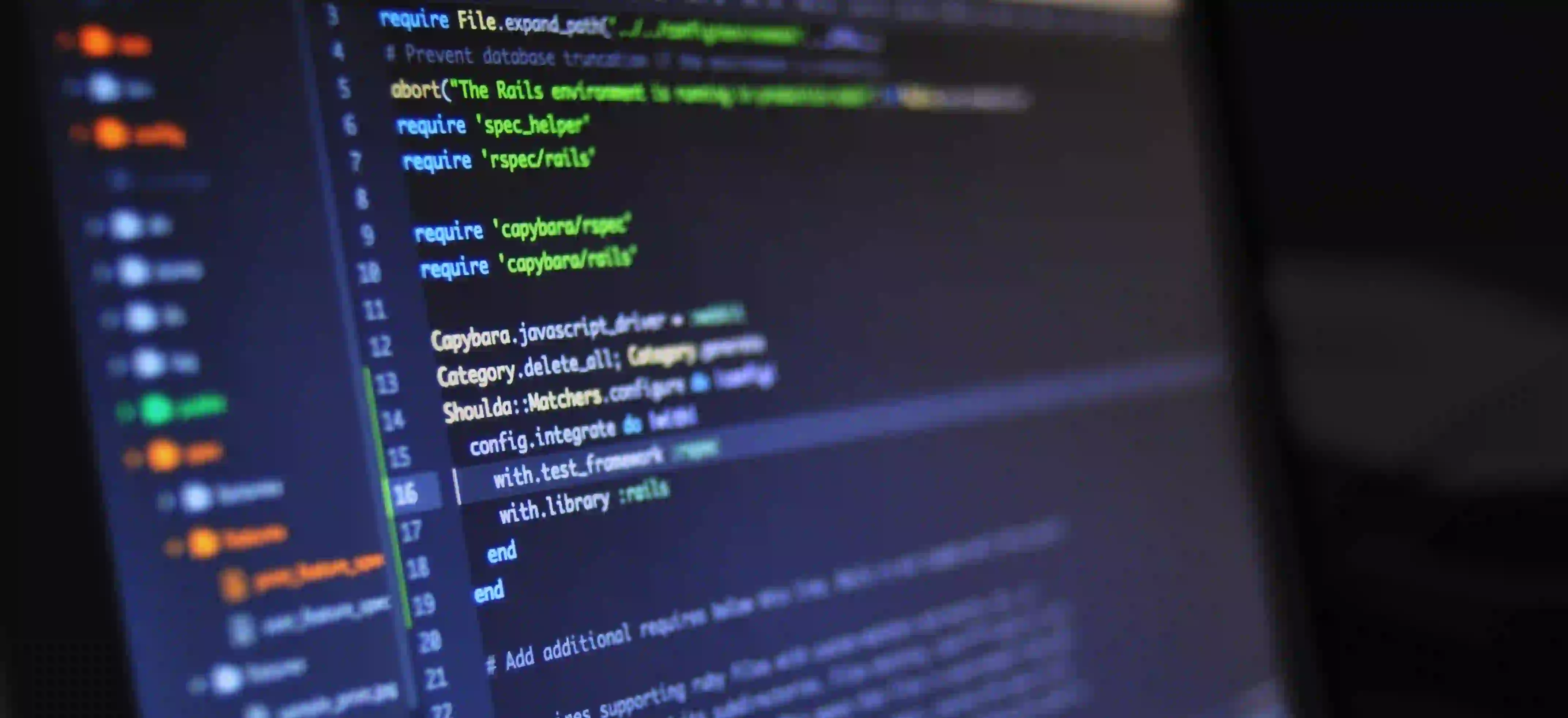
Spice Up Your Java: Integrating Clove-Infused Logic
In the world of programming, just like in cooking, the right ingredients can make a world of difference. One intriguing ingredient that certainly adds a unique flavor to our Java code is clove, or as it is called in German, Gewürznelken. You might be wondering what these aromatic little buds have to do with programming. Well, with their various applications in cooking and medicine, clove serves as an excellent metaphor for how the right coding techniques can transform your application from mundane to extraordinary. For those interested in both culinary arts and programming, you can check out the article "Gewürznelken: Kleine Kostbarkeiten mit großer Wirkung" here.
In this blog post, we will explore how to spice up your Java applications with some essential features and best practices, drawing parallels to the rich utility of clove in various dishes. We will cover object-oriented principles, the importance of design patterns, and practical tips to make your Java code cleaner and more efficient. So, let's dive in!
The Power of Object-Oriented Programming
Much like how clove can enhance the flavor of a dish, Object-Oriented Programming (OOP) enhances the structure and reusability of your code. OOP principles—encapsulation, inheritance, and polymorphism—are fundamental when it comes to building scalable applications in Java.
Encapsulation
Encapsulation is about bundling the data (attributes) and methods (functions) that operate on the data into a single unit or class. It protects the integrity of the object and reduces complexity.
Here's a concise example illustrating encapsulation:
public class Clove {
private String origin;
private double weight;
public Clove(String origin, double weight) {
this.origin = origin;
this.weight = weight;
}
public String getOrigin() {
return origin;
}
public double getWeight() {
return weight;
}
}
In this class, the attributes origin
and weight
are encapsulated. By using getter methods, we restrict direct access to these fields, enabling better control over how they are accessed and modified.
Inheritance
Inheritance allows one class to inherit the properties and behaviors of another, promoting code reusability. For example, we can create a subclass of Clove
to define a specific type, say WholeClove
:
public class WholeClove extends Clove {
private boolean isOrganic;
public WholeClove(String origin, double weight, boolean isOrganic) {
super(origin, weight);
this.isOrganic = isOrganic;
}
public boolean isOrganic() {
return isOrganic;
}
}
Here, WholeClove
extends the Clove
class, adding the isOrganic
attribute. This method allows us to build a more specific class without rewriting existing code, just as different recipes can build off a base flavor.
Polymorphism
Polymorphism allows for methods to do different things based on the object it is acting upon, enhancing flexibility. Consider the method brew()
that functions differently in different scenarios.
public class CloveTea {
public void brew(Clove clove) {
if (clove instanceof WholeClove) {
System.out.println("Brewing tea with organic clove.");
} else {
System.out.println("Brewing tea with regular clove.");
}
}
}
In this code, the brew
method takes a Clove
object but behaves differently if it's a WholeClove
. Just like how different types of clove can bring varied health benefits, this technique diversifies functionality.
Design Patterns: The Recipes of Software Development
Design patterns are standardized solutions to common programming problems. Much like a chef utilizes thousands of recipes, programmers employ design patterns to tackle typical design issues efficiently.
Singleton Pattern
The Singleton pattern ensures a class has only one instance while providing a global point of access to it. Let’s say we want to control the access to your application’s spice inventory:
public class SpiceInventory {
private static SpiceInventory instance;
private SpiceInventory() {}
public static SpiceInventory getInstance() {
if (instance == null) {
instance = new SpiceInventory();
}
return instance;
}
}
This example ensures that no matter how many times you try to create a SpiceInventory
object, you will always get the same instance, much like how the essence of clove should always remain intact in a dish.
Factory Pattern
The Factory pattern provides a way to create objects without exposing the creation logic to the client. This is particularly useful when your code requires the instantiation of various spice types dynamically.
public class CloveFactory {
public static Clove createClove(String type) {
if (type.equals("whole")) {
return new WholeClove("Madagascar", 0.5, true);
} else {
return new Clove("Vietnam", 0.3);
}
}
}
With this factory method, you can create either a whole clove or regular clove by simply providing a parameter. This reduces direct dependencies and allows easier testing and scalability.
Practical Tips for Cleaner Code
To make your code not only functional but also readable and maintainable, consider these tips:
-
Consistent Naming Conventions: Just as culinary ingredients are consistently labeled, ensure that your class and method names are descriptive and follow a standard naming style.
-
Modular Design: Organize your code into smaller, reusable components. This allows any changes or improvements—like a chef adjusting spices in a recipe—without affecting the whole dish.
-
Write Tests: As clove can have unexpected flavors if not measured correctly, ensure that your code is well-tested, catching errors before they disrupt the application's functionality.
-
Documentation: Clear documentation acts as a recipe book for your code. It guides others (and your future self) through the intricacies of your logic.
Closing the Chapter
The beauty of Java lies in its ability to embody principles that mirror the art of cooking. Just as clove can enhance a dish’s profile, applying the principles of Object-Oriented Programming, leveraging design patterns, and adhering to coding best practices can dramatically improve your Java applications.
Remember that, much like tempting dishes that signify the culinary skill, elegant code represents a programmer’s expertise. With every line you write, think of the flavors you want to build into your application. Integrate the clove-infused logic of structured and efficient programming for a mixture so divine, it will beg to be tasted.
Spice up your Java, and enjoy coding!
For more inspiring content on the wonders of spice, feel free to check out the article "Gewürznelken: Kleine Kostbarkeiten mit großer Wirkung" here.