Avoiding Namespace Conflicts in Java Authentication Frameworks
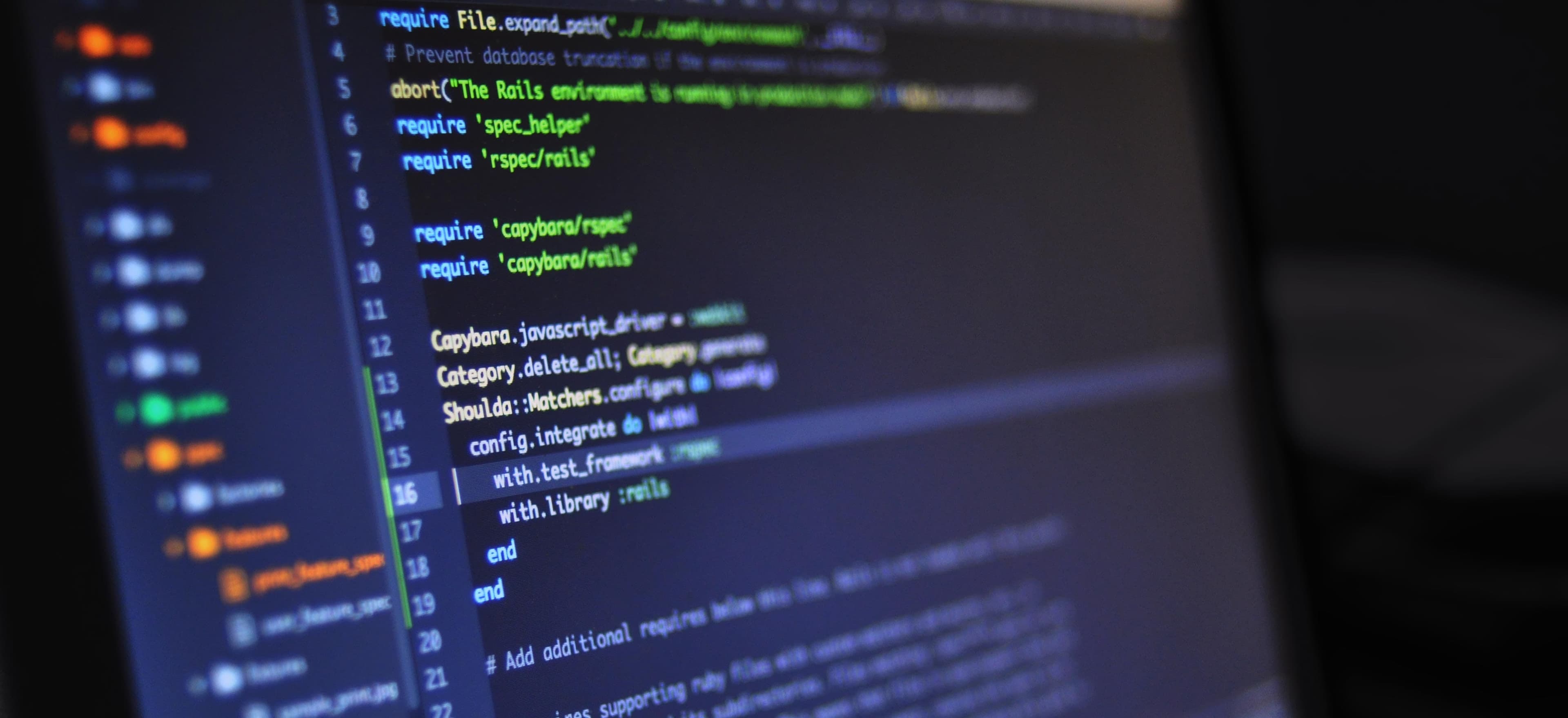
- Published on
Avoiding Namespace Conflicts in Java Authentication Frameworks
In the world of application development, namespace management is critical to maintaining a clean and modular codebase. This holds particularly true when working with authentication frameworks where conflicts can pose risks to security and functionality. This blog post discusses common pitfalls and strategies for avoiding namespace conflicts, drawing parallels with a similar issue discussed in PHP with the article titled Resolving Namespace Conflicts in PHPAuth: A Guide.
Understanding Namespace Conflicts in Java
A namespace is a container that holds a set of identifiers, allowing developers to distinguish between identifiers that may have the same name but belong to different contexts. In Java, namespaces are chiefly associated with packages. Each Java file can belong to a package, which influences how classes and interfaces interact.
Namespace conflicts occur when two classes (or methods) share the same name within the same package or expectation scope, leading to ambiguity. Imagine the confusion that could arise if two classes with the name Authenticator
exist in the same package. As the saying goes, "names matter."
Why Namespace Management is Crucial
- Readability: Avoiding conflicts improves code readability and maintainability.
- Reusability: Namespaces enhance the ability to reuse code without conflicts.
- Security: Authentication processes handling user credentials need to avoid logical ambiguities.
Case Study: Common Namespace Conflicts
Let's take a closer look at how namespace conflicts can occur using an example of two different authentication frameworks, OAuth
and JWT
. If we accidentally import both classes with similar naming conventions into the same file, it can lead to compilation errors or unexpected behavior.
Code Example
import com.auth.oauth.Authenticator;
import com.auth.jwt.Authenticator; // Potential conflict!
public class AuthenticationService {
private Authenticator authenticator;
// Constructor
public AuthenticationService() {
this.authenticator = new Authenticator(); // Which Authenticator is this?
}
}
Commentary: The second Authenticator
class import leads to conflict. Java wouldn’t know which one to instantiate, resulting in compilation failure.
Solution: Use Fully Qualified Names
One of the most straightforward solutions is to use fully qualified names.
public class AuthenticationService {
private com.auth.oauth.Authenticator oauthAuthenticator;
private com.auth.jwt.Authenticator jwtAuthenticator;
// Constructor
public AuthenticationService() {
this.oauthAuthenticator = new com.auth.oauth.Authenticator();
this.jwtAuthenticator = new com.auth.jwt.Authenticator();
}
}
Why This Works
By specifying the entire package name, you eliminate any ambiguity around which Authenticator
class you are referring to. This approach is straightforward and leverages Java's package structure effectively.
Best Practices to Prevent Namespace Conflicts
Here are some best practices to keep your Java applications free from namespace conflicts:
1. Package Naming Conventions
Use logical and hierarchical naming conventions that reflect the functionality. For example, if you are developing an e-commerce application, use com.ecommerce.auth
instead of vague names like com.auth
.
2. Avoid Wildcard Imports
Using import *
can make tracking which classes are imported difficult. Stick to importing specific classes:
import com.auth.oauth.Authenticator;
import com.auth.jwt.Authenticator; // Better: avoid this!
3. Separate Modules
If your application is divided into multiple modules, ensure that each module has a distinct namespace. This is especially important for libraries and dependencies.
4. Use Interfaces and Abstract Classes
Define interfaces that your classes can implement or extend. This helps allow different implementations without causing name clashes.
Code Snippet
public interface Authenticator {
void authenticate();
}
public class OAuthAuthenticator implements Authenticator {
public void authenticate() {
// OAuth logic
}
}
public class JwtAuthenticator implements Authenticator {
public void authenticate() {
// JWT logic
}
}
Commentary: By creating an Authenticator
interface, you allow diverse implementations without conflicting class names. Each specific authenticator can then be easily managed.
When Conflicts Arise: Resolving Existing Issues
Despite our best practices, sometimes conflicts arise. If you are integrating multiple libraries, you can use the following techniques for resolution:
-
Refactor your Code: This is especially relevant if you’re stuck with legacy code that introduces conflicts. Use IDE tools that assist in refactoring.
-
Check for Deprecated Libraries: Libraries that are outdated may cause conflicts with current versions. Always ensure you're using the latest, compatible library versions.
-
Scope Classes Appropriately: Use
inner classes
ornested classes
for encapsulation if certain classes are tightly bound to one another.
Example of Inner Classes
public class AuthService {
class LocalAuthenticator {
void authenticate() {
// local auth logic
}
}
class RemoteAuthenticator {
void authenticate() {
// remote auth logic
}
}
}
Commentary: The inner classes LocalAuthenticator
and RemoteAuthenticator
are scoped within the AuthService
context, effectively avoiding the potential namespace conflict when integrated into a larger codebase.
The Last Word
Namespace conflict management is a vital aspect of Java programming, especially within authentication frameworks, where clarity and security are paramount. By following best practices such as proper package naming, avoiding wildcard imports, and encapsulating classes appropriately, developers can significantly reduce the risks associated with namespace conflicts.
If you want to learn more about namespace conflicts and their resolutions in programming frameworks, the article titled Resolving Namespace Conflicts in PHPAuth: A Guide offers insightful strategies that are applicable across languages, including Java.
Ultimately, by approaching authentication layer constructions with thoughtful namespace management, you position your applications for greater maintainability, readability, and security — principles that every developer should strive for.
Stay safe, stay secure, and happy coding!
Checkout our other articles