Navigating Java's Namespace Issues in Frameworks
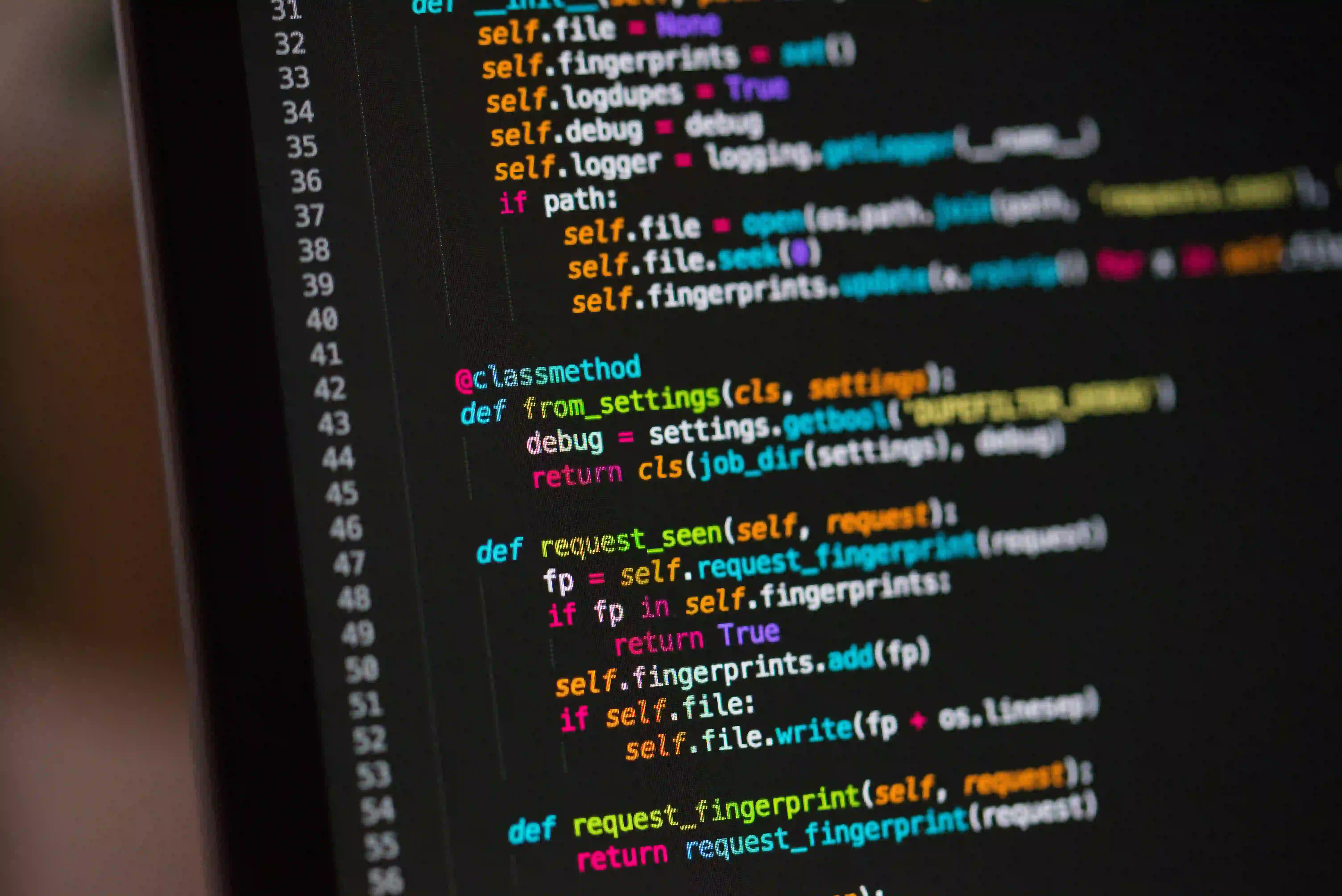
Navigating Java's Namespace Issues in Frameworks
Java is a versatile and widely-used programming language, known for its stability and performance, which makes it a favorite in enterprise applications. However, developers often encounter namespace issues, especially when working with frameworks that might introduce namespace conflicts.
In this post, we will explore the ramifications of namespace issues in Java frameworks, discuss practical approaches to mitigate these conflicts, and provide insights into effective namespace management.
Understanding Namespaces in Java
In Java, a namespace is a container that holds a set of identifiers, such as class names, method names, and variable names. A namespace allows us to organize code and prevent name collisions.
Java uses packages to create namespaces. A package is essentially a directory that contains a set of related classes and interfaces. By using packages, we can have classes with the same name in different contexts.
For example, you can have:
package com.example.service;
public class User {
// User class implementation
}
and
package com.example.dao;
public class User {
// Another User class implementation
}
These two User classes can exist in the same project without conflicts due to their different package structures.
Why Namespace Issues Arise
Namespace issues typically arise from:
- Multiple Libraries: When integrating multiple libraries or frameworks, it is possible to encounter classes with identical names.
- Imported Classes: When classes are imported from different packages, they can overshadow one another, causing conflicts.
- Poor Package Structure: A poorly organized codebase can lead to confusion and errors when locating classes.
Example of a Namespace Conflict
Imagine integrating two libraries that have a class with the same name:
Library A:
package com.libA.model;
public class User {
// Implementation A
}
Library B:
package com.libB.model;
public class User {
// Implementation B
}
If you try to import both into your project, you might face an issue like this:
import com.libA.model.User; // Importing User from Library A
import com.libB.model.User; // This will cause a conflict!
You could resolve this by using fully qualified names or by aliasing, but these techniques can clutter the code and reduce readability.
Strategies for Resolving Namespace Conflicts
1. Use Fully Qualified Names
Using fully qualified names helps avoid ambiguity. Instead of just writing User
, you specify it as com.libA.model.User
or com.libB.model.User
.
public class UserService {
public void createUser(com.libA.model.User user) {
// Implementation to create a user from Library A
}
public void createUser(com.libB.model.User user) {
// Implementation to create a user from Library B
}
}
2. Refactor Libraries
If you have control over the libraries, consider renaming classes or creating different packages for conflicting classes. This is especially useful in custom libraries or private projects but may not always be practical for third-party libraries.
3. Avoid Static Imports
Static imports can lead to namespace collision, especially when you import methods or fields from classes that have the same name. Use conventional imports to minimize conflicts.
4. Create Utility Classes
To compartmentalize functionality and reduce namespace clutter, you can create utility classes. This is akin to leveraging the Singleton pattern but maintains separation.
package com.example.utils;
public class UserUtil {
public static void processUser(com.libA.model.User user) {
// Processing logic
}
public static void processUser(com.libB.model.User user) {
// Processing logic for Library B
}
}
5. Dependency Management Tools
Using tools like Maven or Gradle can help in managing conflicts better. They allow you to specify and resolve dependencies efficiently. For instance, defining dependencies in Maven pom.xml can fetch libraries with compatible versions, reducing the risk of conflicts.
<dependency>
<groupId>com.libA</groupId>
<artifactId>library-a</artifactId>
<version>1.0.0</version>
</dependency>
<dependency>
<groupId>com.libB</groupId>
<artifactId>library-b</artifactId>
<version>1.1.0</version>
</dependency>
Best Practices for Namespace Management
-
Organize Packages Logically: Have a clear structure for your packages that reflects their hierarchy and purpose.
-
Adopt Naming Conventions: Consistent naming conventions can help differentiate between similar classes. For instance, append strategic suffixes or prefixes based on the library source.
-
Regular Refactoring: As your codebase grows, regularly refactor and analyze the package structure for efficiency.
-
Thorough Documentation: Maintain detailed documentation about the packages and classes in your application. This practice aids in understanding and resolving namespace conflicts when they arise.
-
Use Community Resources: Reference guides like Resolving Namespace Conflicts in PHPAuth: A Guide can provide helpful insights, even when moving between programming languages.
Bringing It All Together
While namespace issues in Java frameworks can be a daunting challenge, understanding the underlying principles of namespaces, addressing potential conflicts proactively, and adhering to best practices can substantially ease the development process.
By applying strategies such as using fully qualified names, organizing packages systematically, and utilizing dependency management tools, you can mitigate the risks of namespace conflicts. Additionally, maintaining a well-documented and organized codebase will significantly enhance code readability and maintainability.
In a world filled with evolving libraries and frameworks, adopting these practices will empower you to navigate namespace issues effectively and focus on building exceptional applications.