Avoiding Java Microservices Pitfalls with Istio on EKS
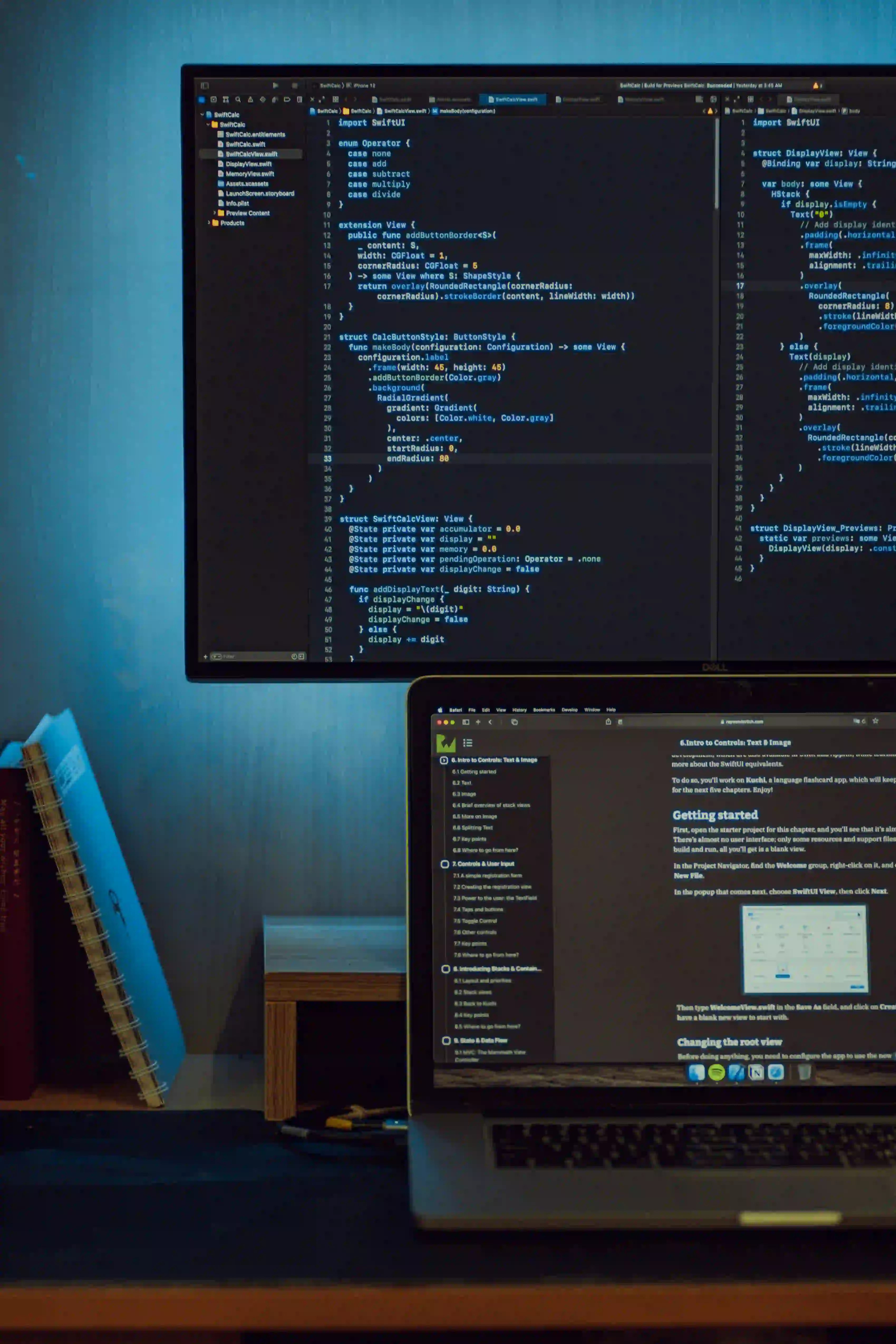
Avoiding Java Microservices Pitfalls with Istio on EKS
In the realm of cloud computing, microservices have become the de facto standard for developing scalable applications. Java, with its rich ecosystem, has remained a preferred choice for implementing these services. However, deploying Java microservices can lead to complications, particularly when using platforms like Amazon's Elastic Kubernetes Service (EKS). This is where Istio, a powerful service mesh, comes into play. In this article, we will guide you through the common pitfalls when deploying Java microservices with Istio on EKS and provide practical strategies to overcome them.
Understanding Microservices and Service Meshes
Microservices architecture allows developers to build applications as a set of loosely coupled services. This modularity facilitates independent development, deployment, and scaling. However, managing inter-service communication introduces complexity that can be efficiently handled by a service mesh like Istio.
Istio provides several key features that can improve microservices communication:
- Traffic Management: Directing traffic between services intelligently.
- Security Policies: Enforcing security through authentication and authorization.
- Observability: Collecting telemetry data for monitoring and troubleshooting.
By leveraging Istio with EKS, developers can deploy robust Java microservices while avoiding many common pitfalls.
Common Pitfalls in Java Microservices Deployment with Istio
1. Overlooking Istio's Sidecar Architecture
Istio injects a sidecar proxy (usually Envoy) alongside each service instance. This architecture facilitates inter-service communication, but developers often overlook this setup, assuming their service communicates directly.
Why it Matters: Failure to configure the sidecar can lead to performance issues and loss of features such as traffic management or security.
Implementation Example
Here’s a basic example of a Java microservice configured to work with Istio:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
@RestController
public class HelloWorldService {
public static void main(String[] args) {
SpringApplication.run(HelloWorldService.class, args);
}
@GetMapping("/hello")
public String sayHello() {
return "Hello from Java Microservice!";
}
}
Commentary: Ensure that your service is packaged with necessary annotations so Istio can intercept requests correctly. Any misconfigurations here can result in bypassing the Istio functionalities.
2. Neglecting Gateway Configuration
Istio utilizes gateways for managing ingress and egress traffic. New users often neglect to configure gateways properly, leading to failed requests and security vulnerabilities.
Why it Matters: Proper configuration ensures that services are accessible from outside the cluster and that security policies are enforced.
Implementation Example
Here is an example of an Istio gateway configuration:
apiVersion: networking.istio.io/v1beta1
kind: Gateway
metadata:
name: hello-world-gateway
namespace: default
spec:
selector:
istio: ingressgateway # use Istio's built-in ingress gateway
servers:
- port:
number: 80
name: http
protocol: HTTP
hosts:
- "*"
Commentary: This configuration allows any host to access the service over HTTP. You can refine the hosts section for more granular control.
3. Ignoring Circuit Breaker Patterns
When multiple microservices are interdependent, slow or failing services can cause a ripple effect. Istio provides circuit breaker patterns, but they are often ignored.
Why it Matters: Implementing circuit breakers prevents cascading failures and improves resilience.
Implementation Example
Here’s how to define a circuit breaker in your Istio configuration:
apiVersion: networking.istio.io/v1beta1
kind: DestinationRule
metadata:
name: hello-world-destination
spec:
host: hello-world-service
trafficPolicy:
outlierDetection:
maxConnections: 1
maxPendingRequests: 1
maxRetries: 3
minHealthPercent: 100
interval: 5s
baseEjectionTime: 30s
Commentary: This rule triggers an ejection when the service experiences an unhealthy state. Properly set metrics can prevent ongoing failures.
4. Misconfiguring Security Policies
Security is paramount when deploying microservices. Istio simplifies service-to-service authentication and authorization through its security policies. However, misconfigurations can lead to security loopholes.
Why it Matters: Failure to set up Policies correctly can expose your microservices to vulnerabilities.
Implementation Example
Consider the following configuration for mutual TLS (mTLS):
apiVersion: security.istio.io/v1beta1
kind: PeerAuthentication
metadata:
name: default
namespace: default
spec:
mtls:
mode: REQUIRE
Commentary: This ensures that all services within the namespace will use mTLS, adding an essential security layer to your communication.
5. Lacking Observability
Finally, it’s vital to implement observability through logging, tracing, and monitoring. Istio integrates nicely with tools like Prometheus, Grafana, and Jaeger.
Why it Matters: Lack of visibility into microservice interactions can lead to prolonged downtimes and undiagnosed issues.
Quick Setup Example
Here’s a snippet of how to enable metrics in your Istio configuration:
apiVersion: telemetry.istio.io/v1alpha1
kind: ServiceMonitor
metadata:
name: hello-world-monitor
namespace: istio-system
spec:
endpoints:
- port: http
interval: 30s
selector:
matchLabels:
app: hello-world
Commentary: This configuration exposes metrics to monitoring tools, allowing you to troubleshoot performance issues effectively.
Wrapping Up
Deploying Java microservices on EKS with Istio can be daunting, but by avoiding common pitfalls, you can create a robust, secure, and scalable application. Always keep in mind that the initial setup may require deeper insights into how Istio operates. Properly configuring sidecars, gateways, security protocols, and observability aspects can make the difference between success and frustrating errors.
For a deeper insight into potential obstacles when deploying Istio on EKS, check out the article "Common Pitfalls When Deploying Istio on EKS" at configzen.com/blog/pitfalls-istio-deploy-eks.
By ensuring you are well-versed in these configurations and considerations, you will pave the way for a smoother deployment process, allowing you to focus on building great Java microservices.
Additional Resources
Through these resources, you can continue to enhance your knowledge, ensuring that your journey into the microservices architecture effectively leverages all the benefits that modern tools offer. Happy coding!