Avoiding Common Pitfalls When Scaling Java Applications
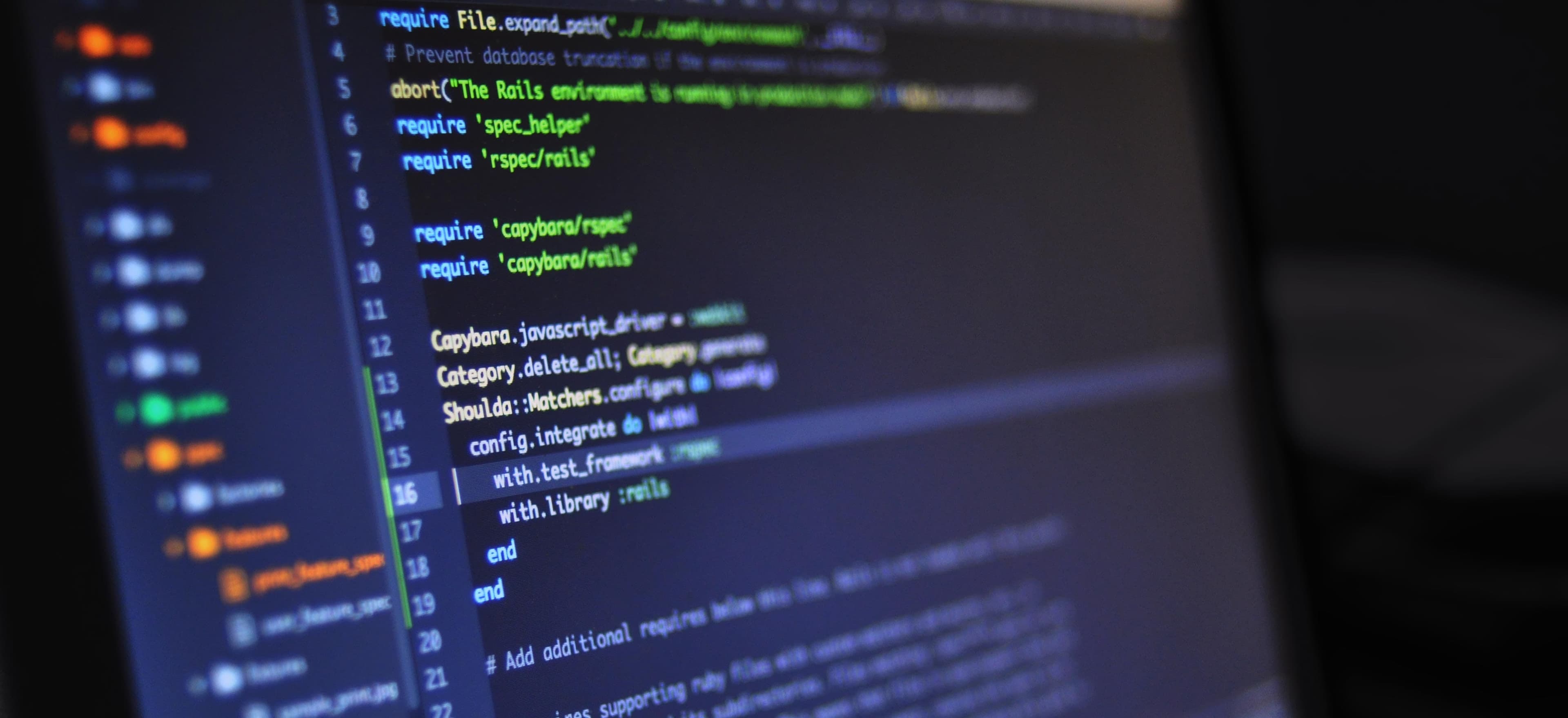
- Published on
Avoiding Common Pitfalls When Scaling Java Applications
Scaling applications is a crucial aspect of modern software development. With the increasing demand for robust and efficient applications, Java developers must navigate various challenges that arise during the scaling process. In this blog post, we’ll explore some common pitfalls in scaling Java applications and how to avoid them. We will also discuss best practices, along with sample code snippets for clarity and practical implementation.
Understanding Scalability
Before diving into the pitfalls, let's clarify what scalability means. Scalability is the capability of a system to handle a growing amount of work or its potential to accommodate growth. In the context of Java applications, it refers to the ability of the application to handle increased loads without performance degradation.
Common Pitfalls in Scaling Java Applications
1. Not Considering the Architecture
Why It Matters: The underlying architecture significantly affects how well an application can scale. A monolithic design can work well for smaller applications but can become a bottleneck as the application grows.
Best Practice: Use a microservices architecture that allows you to break down the application into smaller, independent services. This approach enables individual components to scale based on demand.
Example: Let's say you have an e-commerce application. Instead of having one monolithic application, you can separate it into different services like Product Service, Order Service, and User Service. This way, each service can be scaled independently.
@RestController
@RequestMapping("/products")
public class ProductService {
@GetMapping("/")
public List<Product> getAllProducts() {
// Logic to fetch and return all products
}
}
2. Ignoring Database Optimization
Why It Matters: A poorly optimized database can severely hinder your application's performance when scaled. As the application grows, query slowdowns can lead to a poor user experience.
Best Practice: Implement indexing, partitioning, and caching strategies. Use tools like Hibernate to optimize queries.
Example: Here's how you can use an indexed query with JPA to speed up data retrieval.
@Entity
@Table(name = "products")
public class Product {
@Id
private long id;
@Column(name = "product_name")
@Index(name = "product_name_index")
private String name;
// Other fields and methods...
}
3. Not Using Asynchronous Processing
Why It Matters: Using synchronous processing for tasks that do not require immediate results can lead to thread blocking and reduced performance, particularly under heavy load.
Best Practice: Employ asynchronous processing or background jobs for long-running tasks. This ensures that your application remains responsive.
Example: You can use the CompletableFuture
class to run tasks asynchronously.
public CompletableFuture<Order> processOrder(Order order) {
return CompletableFuture.supplyAsync(() -> {
// Process the order
return order;
});
}
4. Failing to Monitor Performance
Why It Matters: Without proper monitoring, identifying performance bottlenecks becomes challenging, especially as the application scales.
Best Practice: Use monitoring tools such as Prometheus, Grafana, or New Relic. These tools allow you to track metrics like response times, error rates, and system resource usage.
Example: You can integrate Micrometer with Spring Boot for easy metrics tracking.
import io.micrometer.core.instrument.MeterRegistry;
@RestController
public class UserController {
private final MeterRegistry meterRegistry;
public UserController(MeterRegistry meterRegistry) {
this.meterRegistry = meterRegistry;
}
@GetMapping("/users")
public List<User> getUsers() {
meterRegistry.counter("users.request.count").increment();
return userService.getAllUsers();
}
}
5. Neglecting Load Testing
Why It Matters: Jumping into production without proper load testing can lead to performance issues and application crashes due to unanticipated loads.
Best Practice: Use load-testing tools such as JMeter or Gatling to simulate high traffic and identify potential breaking points.
Example: You can set up a JMeter test plan to simulate multiple users accessing an API endpoint simultaneously, allowing you to measure response times under load.
6. Not Following Best Practices for Code Quality
Why It Matters: High-quality code is easier to maintain, scale, and test. Poor code quality can accumulate technical debt that becomes costly to address.
Best Practice: Implement best coding practices, including code reviews, consistent naming conventions, and adherence to SOLID principles.
Example: An example of using interfaces to adhere to the Dependency Inversion Principle:
public interface PaymentService {
void processPayment(Payment payment);
}
public class CreditCardPaymentService implements PaymentService {
public void processPayment(Payment payment) {
// Logic for processing credit card payment
}
}
7. Mismanaging Dependencies
Why It Matters: Overusing dependencies can increase complexity and lead to versioning conflicts. Failing to manage versions correctly may also lead to performance issues.
Best Practice: Keep dependencies updated and use dependency management tools like Maven or Gradle to handle versioning efficiently.
Example: Use Maven's "dependencyManagement" section to control versions across your application.
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
<version>2.6.0</version>
</dependency>
</dependencies>
</dependencyManagement>
8. Underestimating Network Considerations
Why It Matters: Network latency can hamper application performance, especially in distributed systems. Ignoring network efficiency can lead to slow communication between services.
Best Practice: Use network protocols and serialization formats that minimize latency, such as gRPC for microservices communication.
Example: Using gRPC in a Java application for efficient service-to-service communication:
// Service definition
service OrderService {
rpc CreateOrder(OrderRequest) returns (OrderResponse);
}
The Closing Argument
Scaling Java applications involves understanding the intricacies of architecture, database management, performance optimization, and monitoring. By avoiding common pitfalls and applying best practices, developers can enhance the scalability and robustness of their applications.
Remember, the lessons learned from scaling Java applications can parallel those found in other environments. For instance, the key takeaways from the article "Common Mistakes in Building Scalable Node.js Apps" reflect similar challenges faced by developers regardless of the programming framework they use.
Embrace these practices as you embark on your journey to build scalable Java applications, and ensure your applications meet the demands of users now and in the future. Happy coding!
Checkout our other articles