Streamline JavaFX Image Handling for Better Title Display
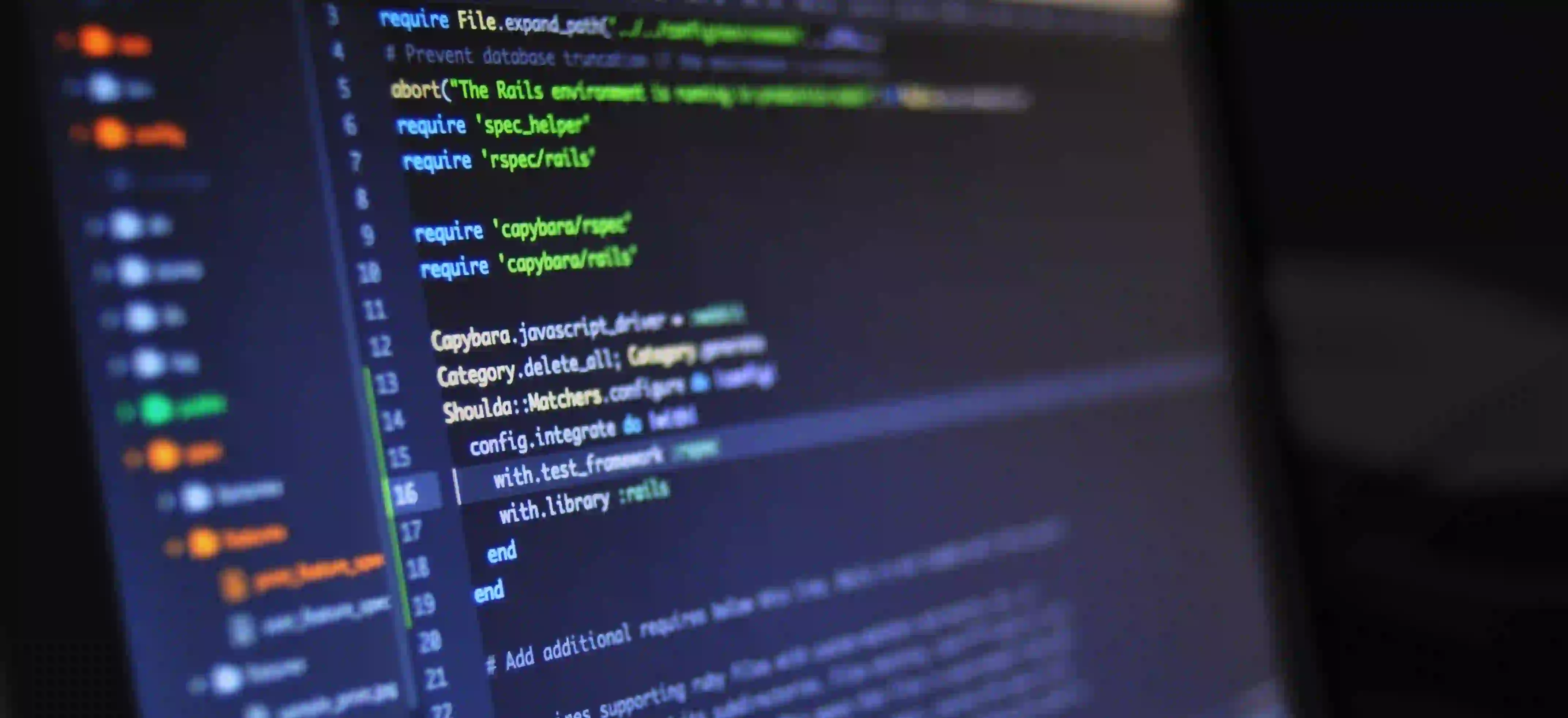
Streamline JavaFX Image Handling for Better Title Display
JavaFX is a powerful framework that provides a rich set of graphics and media tools to create modern desktop applications. One essential aspect is image handling, especially regarding how images and their titles are displayed to users. In this blog post, we will explore how to streamline JavaFX image handling for better title display, thus enhancing user experience. We will incorporate insights from the referenced article "Struggling with Chrome's Image Title Display? Fix It!" available at infinitejs.com/posts/chrome-image-title-fix, which highlights the importance of proper image title handling.
Understanding JavaFX and Image Display
JavaFX offers several classes for working with images. The Image
and ImageView
classes are the primary ones used for displaying images in an application. Here’s a quick look at the basic syntax for utilizing these classes:
Image image = new Image("path/to/image.png");
ImageView imageView = new ImageView(image);
Why Proper Image Handling Matters
Proper image handling goes beyond just displaying graphics. It can significantly affect how users perceive an application's usability and professionalism. A common issue that arises is the lack of context for images, especially if they lack titles or descriptions. This is particularly relevant when it comes to web browsers, where image titles can directly influence user interaction.
Optimizing Image Title Handling
Images often serve to convey messages or information. Thus, displaying the title or description effectively enhances user engagement. Here are some tips for optimizing image title handling in JavaFX.
1. Use Tooltips for Image Titles
One effective way to display image titles in a JavaFX application is by using tooltips. Tooltips provide context for images and can describe what an image represents. To implement tooltips, use the following code snippet:
ImageView imageView = new ImageView(image);
imageView.setTooltip(new Tooltip("This is the image title"));
Why Use Tooltips? Tooltips are non-intrusive and provide users with the option to learn more about the image without cluttering the interface. They appear dynamically, which keeps the UI clean.
2. Display Title Underneath the Image
Another method is to display the title directly underneath the image. This option can combine an ImageView
with a Label
to create a titled image representation.
VBox vbox = new VBox();
vbox.getChildren().addAll(imageView, new Label("Image Title"));
Why Use a VBox?
The VBox
layout manager allows you to stack components vertically, making it easy to associate the title with the image. This approach enhances visibility and allows users to immediately see what the image depicts.
Enhancing Image Loading with Progress Indicators
When dealing with large images or slow network conditions, it's vital to keep the user informed about what's happening. JavaFX allows us to include a progress indicator while the image is loading.
ImageView imageView = new ImageView();
ProgressIndicator progressIndicator = new ProgressIndicator();
progressIndicator.setVisible(true);
// Load the image on a separate thread
new Thread(() -> {
Image image = new Image("path/to/image.png");
Platform.runLater(() -> {
imageView.setImage(image);
progressIndicator.setVisible(false);
});
}).start();
VBox vbox = new VBox(progressIndicator, imageView);
Why Use a Progress Indicator? It enhances user experience by providing feedback that something is happening, thereby reducing uncertainty, especially in cases of delayed image loading.
Caching Images for Better Performance
To improve performance, especially in applications that require frequent image loading, JavaFX’s built-in caching mechanism can help. You can enable caching with:
imageView.setCache(true);
Or, if you need to manage a large number of images:
Image myImage = new Image("path/to/image.png", true);
ImageView imageView = new ImageView(myImage);
imageView.setCache(true);
Why Use Caching? Caching significantly improves loading times and performance for frequently accessed images. By ensuring that images don’t need to be reloaded from disk each time they are displayed, your application runs more smoothly.
Integrating with Web Technologies
JavaFX can integrate seamlessly with web technologies. For example, if you need to pull images from a web service and still display titles or descriptions, you can use libraries like Retrofit
or OkHttp
to make HTTP requests and retrieve images dynamically.
Here’s an example snippet illustrating how you might retrieve an image URL:
// Retrofit service would give you a list of image URLs
Service service = retrofit.create(Service.class);
Call<List<ImageData>> call = service.getImages();
call.enqueue(new Callback<List<ImageData>>() {
@Override
public void onResponse(Call<List<ImageData>> call, Response<List<ImageData>> response) {
for (ImageData data : response.body()) {
Image image = new Image(data.getUrl());
ImageView imageView = new ImageView(image);
imageView.setTooltip(new Tooltip(data.getTitle()));
// Add imageView to your layout
}
}
@Override
public void onFailure(Call<List<ImageData>> call, Throwable t) {
// Handle error
}
});
Why Integrate with APIs? Integrating with web services enables dynamic content management, allowing applications to reflect a continually changing data landscape, which is vital for user engagement.
Key Takeaways
Optimizing image handling in JavaFX is crucial for enhancing user experience through effective title display. By implementing tooltips, displaying titles, leveraging progress indicators, caching images, and integrating with web technologies, you can create a smoother, more professional-looking application.
We hope these tips enable you to improve your JavaFX applications significantly. For further reading on image title display issues in web environments, be sure to check out Struggling with Chrome's Image Title Display? Fix It!. It holds valuable insights that can help in bridging the gaps between JavaFX applications and web standards.
Additional Resources
In conclusion, JavaFX provides a wealth of tools to manipulate and display images effectively. With a few best practices, you can significantly enhance your application's visual appeal and functionality.