Boost Your Java PWA: Tackle Offline Functionality Issues
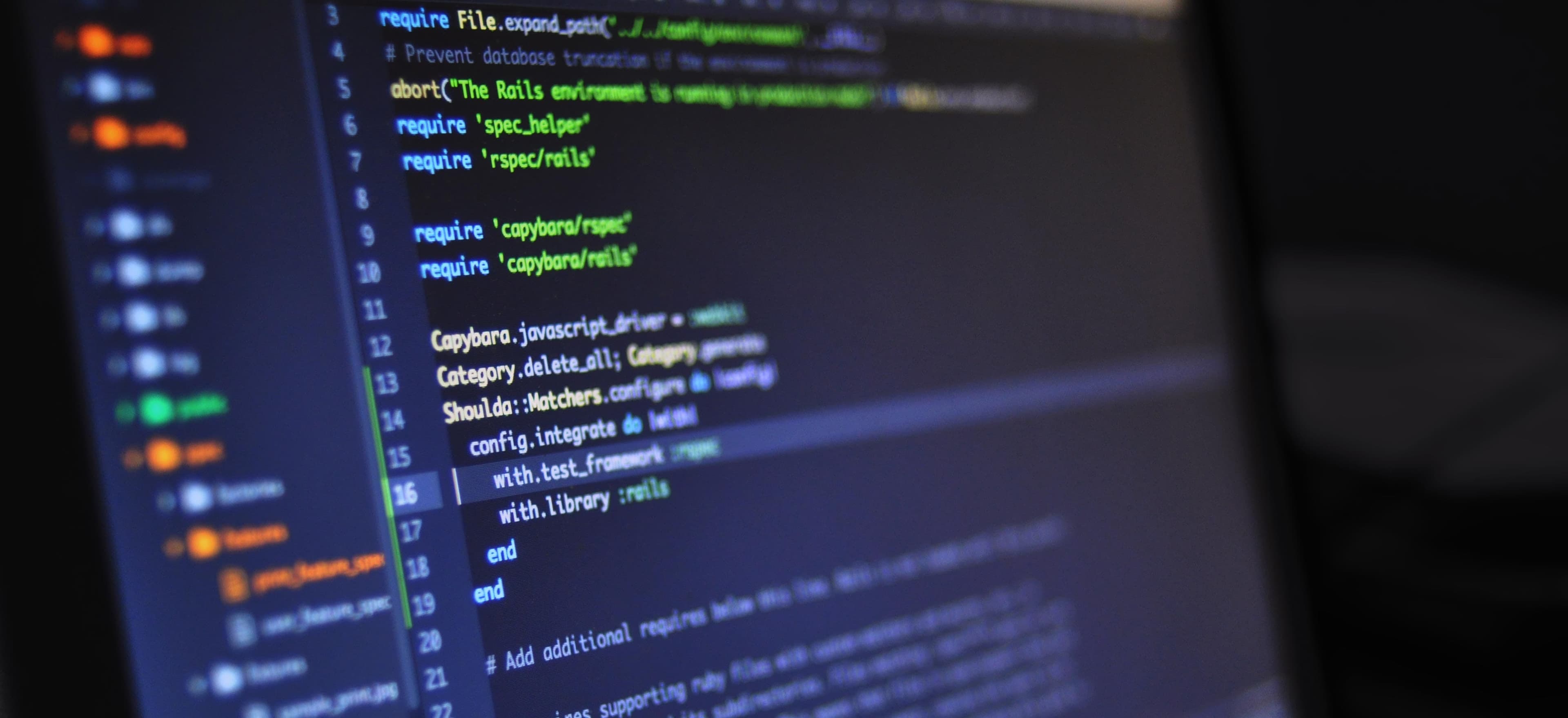
- Published on
Boost Your Java PWA: Tackle Offline Functionality Issues
Progressive Web Applications (PWAs) have revolutionized how we interact with web applications by offering features such as offline capabilities, push notifications, and fast loading times. However, implementing offline functionality can pose challenges, especially when leveraging Java on the backend. In this blog post, we will explore the intricacies of enhancing your Java-based PWA with effective offline functionality solutions. If you struggle with offline capabilities in PWAs, consider reviewing this insightful article titled Overcoming Offline Challenges in PWAs with JavaScript.
Understanding the Importance of Offline Functionality in PWAs
Offline functionality is crucial for ensuring that users can still access your application even without an internet connection. This capability not only improves user engagement but also enhances the application's reliability. With Java being a robust backend technology, Let's dive into how you can enhance your PWA's offline experience.
Core Concepts of Offline Functionality
When we discuss offline capabilities, several key concepts come into play:
-
Service Workers: These are scripts that run in the background, separate from your main web application. They act as a proxy between the network and your application, enabling caching, background sync, and more.
-
Caching Strategies: Understanding various caching strategies (e.g., Cache First, Network First) is vital for determining how resources are stored and retrieved when offline.
-
IndexedDB: This is a client-side storage solution for handling large amounts of structured data. It allows your app to store and retrieve data easily, even when offline.
Setting Up Your Java Backend
To implement offline capabilities effectively, you will need a robust backend. Java offers several frameworks, such as Spring Boot, to help you create RESTful APIs that your PWA can consume.
Here is a basic example of creating a Spring Boot REST API:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@SpringBootApplication
@RestController
public class PwaApplication {
public static void main(String[] args) {
SpringApplication.run(PwaApplication.class, args);
}
@GetMapping("/data")
public String getData() {
return "This is data from your Java backend!";
}
}
In this code snippet, we set up a simple Spring Boot application that serves data through the /data
endpoint. Why do we use Spring Boot? It simplifies the development process with its opinionated approach and built-in features, making it an excellent choice for creating REST APIs.
Caching Strategies for Frontend
Choosing the right caching strategy will determine how your app behaves offline. A common approach is to use the Cache First strategy for resources that don’t change often.
Let's set up a service worker to implement this strategy:
self.addEventListener('install', (event) => {
event.waitUntil(
caches.open('my-cache').then((cache) => {
return cache.addAll([
'/',
'/index.html',
'/styles.css',
'/script.js',
'/data'
]);
})
);
});
self.addEventListener('fetch', (event) => {
event.respondWith(
caches.match(event.request).then((response) => {
return response || fetch(event.request);
})
);
});
Code Commentary
-
Install Event: During the installation of the service worker, we open the cache and add essential resources to it. This ensures that these resources are available offline.
-
Fetch Event: When a network request is made, the code first checks if the request is in the cache. If so, it serves the cached resource. If not, it fetches the request from the network.
By opting for the Cache First strategy, we enhance the user experience, as resources are always readily available.
Leveraging IndexedDB for Offline Storage
To further boost offline functionality, storing user data locally is essential. IndexedDB is a powerful tool for this purpose. Using IndexedDB, you can store and retrieve large amounts of data efficiently.
Here is an example of using IndexedDB to store user preferences:
let db;
const request = indexedDB.open('myDatabase', 1);
request.onupgradeneeded = (event) => {
db = event.target.result;
db.createObjectStore('userPreferences', { keyPath: 'id' });
};
request.onsuccess = (event) => {
db = event.target.result;
saveUserPreference({ id: 1, theme: 'dark' });
};
const saveUserPreference = (preference) => {
const transaction = db.transaction(['userPreferences'], 'readwrite');
const store = transaction.objectStore('userPreferences');
store.put(preference);
};
Understanding the Code
-
Creating and Upgrading Database: The
onupgradeneeded
event fires when a database with a higher version number is opened. In this event, we create an object store for user preferences. -
Saving Data: The
saveUserPreference
function demonstrates how to store preferences in the IndexedDB.
Utilizing IndexedDB allows your PWA to maintain user preferences even when offline, significantly improving the user experience.
Testing Offline Functionality
Testing offline functionality can be easily accomplished using browser developer tools. Here’s a simple method:
- Open your application in Google Chrome.
- Press
F12
to open Developer Tools. - Go to the "Network" tab and check the "Offline" option.
- Navigate around your application to see if the cached resources load seamlessly.
A Final Look
Offline functionality is a vital component of PWAs, enhancing user engagement and reliability. By utilizing service workers, caching strategies, and IndexedDB, you can create a seamless experience for your users.
For more comprehensive strategies and challenges related to offline functionality, don't hesitate to check out the article titled Overcoming Offline Challenges in PWAs with JavaScript.
By following the outlined techniques, your Java PWA can achieve remarkable offline capabilities that not only meet the needs of your users but also elevate your application to a higher standard. Happy coding!