Crafting Java Questions: The Art of Effective Inquiry
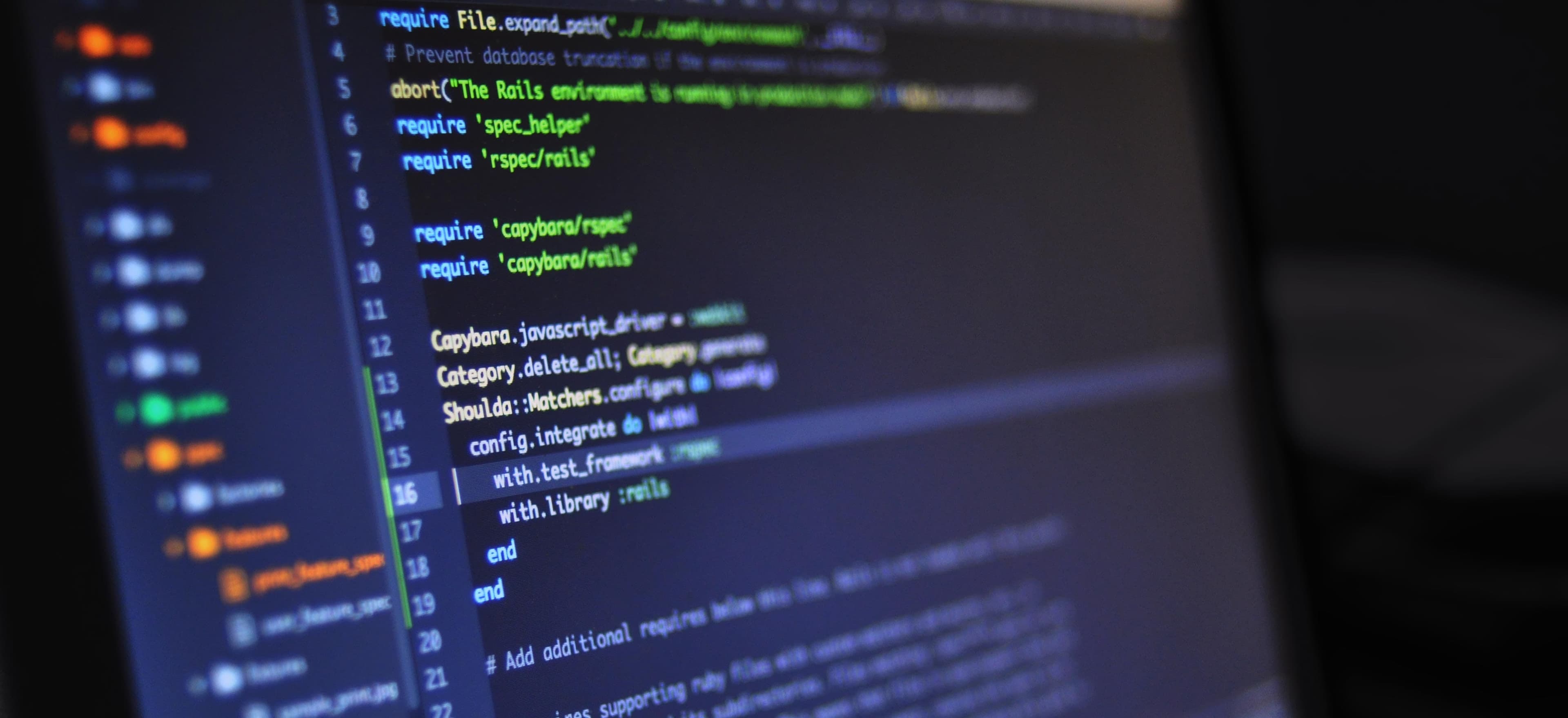
- Published on
Crafting Java Questions: The Art of Effective Inquiry
In the realm of programming and software development, the ability to ask the right questions is just as important as writing impeccable code. Whether you're working on a complex Java project, debugging an application, or seeking help from the development community, the quality of your inquiry significantly impacts the feedback you receive. In this blog post, we will explore strategies to formulate effective Java questions that can guide your learning process, improve collaboration, and ultimately enhance your coding skills.
Why Questions Matter
When engaging with peers or seeking assistance from forums, well-crafted questions can lead to insightful answers and solutions. Here are a few reasons why asking the right questions is essential:
- Clarifies Understanding: Formulating your question helps solidify your understanding of the problem.
- Saves Time: Precise inquiries are more likely to yield quick and relevant answers.
- Encourages Engagement: Engaging questions invite discussions and diverse opinions, enriching the learning experience.
Before we dive into how to structure your Java questions, let’s look at a framework that can help you clarify your thoughts.
The Problem-Solving Framework
A successful question often stems from a clear understanding of the problem. Here’s a framework you can use:
- Identify the Issue: What exactly is not working? Is it a code error, a design flaw, or a misunderstanding of a Java concept?
- Provide Context: What is the background information? Include details like the Java version, libraries you're using, and relevant configuration.
- Show Your Efforts: Share what you have tried so far. This shows initiative and allows responders to tailor their suggestions to what you've already attempted.
- Ask for Specifics: Clearly state what you're looking for. Are you seeking a code snippet, a conceptual explanation, or both?
Crafting Your Java Questions
Now, let's look at some templates to help you phrase your inquiries effectively.
1. The Syntax Error
If you encounter a syntax error in your Java code, providing clear details will help others help you. For instance:
Example Question:
I am getting a compilation error in my Java code:
public class Main { public static void main(String[] args) { int number = 10 System.out.println(number); } }
I forgot to add a semicolon after
int number = 10
. After correcting that, the code runs fine. However, I still don’t understand why syntax errors cause compilation issues. Can someone explain?
Why This Works:
- It presents the code snippet directly.
- It specifies the error (syntax error, missing semicolon).
- Lastly, it asks for clarity on the underlying concept.
2. Debugging Logic Issues
Logic errors can be tricky and often require you to communicate your thought process:
Example Question:
I wrote this method to reverse a string, but it isn't returning the expected output:
public String reverseString(String str) { String reversed = ""; for (int i = str.length(); i >= 0; i--) { reversed += str.charAt(i); } return reversed; }
The method seems to throw an IndexOutOfBoundsException. What might be causing this?
Why This Works:
- You provide a working example of your logic.
- You mention the specific exception which indicates where the issue lies.
- It prompts respondents to give feedback specifically on logic rather than syntax.
3. Asking for Best Practices
Inquiring about best practices can stimulate valuable discussion:
Example Question:
I am working on a Java application where multiple components need to share access to an object. Is it better to use singletons or dependency injection? What are the trade-offs?
Why This Works:
- It frames the inquiry around design choices.
- It invites opinions and fosters discussion on best practices.
Engaging with the Community
When you ask questions in community forums such as Stack Overflow, GitHub, or even Reddit, it’s crucial to engage with the responses you receive. Acknowledge the help, ask follow-up questions, and ensure you understand the answers. This not only improves your understanding but also fosters a collaborative environment.
Moreover, continuously learning is a core aspect of being a developer. For deeper insight into crafting inquiries and understanding perspectives, check out the article titled Tarotkarten: So stellst du die perfekte Frage. Although it discusses a different context, the principles of effective questioning remain the same.
Wrapping Up
Asking effective questions in Java involves a thoughtful approach to problem-solving. By understanding your issues, providing context, detailing your efforts, and being specific in your inquiries, you can greatly enhance the quality of help you receive.
Remember, the goal of asking questions is to learn, grow, and improve your craft. So, embrace every opportunity to inquire and engage with the Java community. Happy coding!
Further Reading
To expand your knowledge on effective strategies in coding, take a look at these resources:
- Java Programming Basics
- Debugging in Java
By incorporating these practices into your inquiry, you will not only become a better coder but also contribute positively to the programming community.