Mastering Java: How to Ask the Right Questions in Code
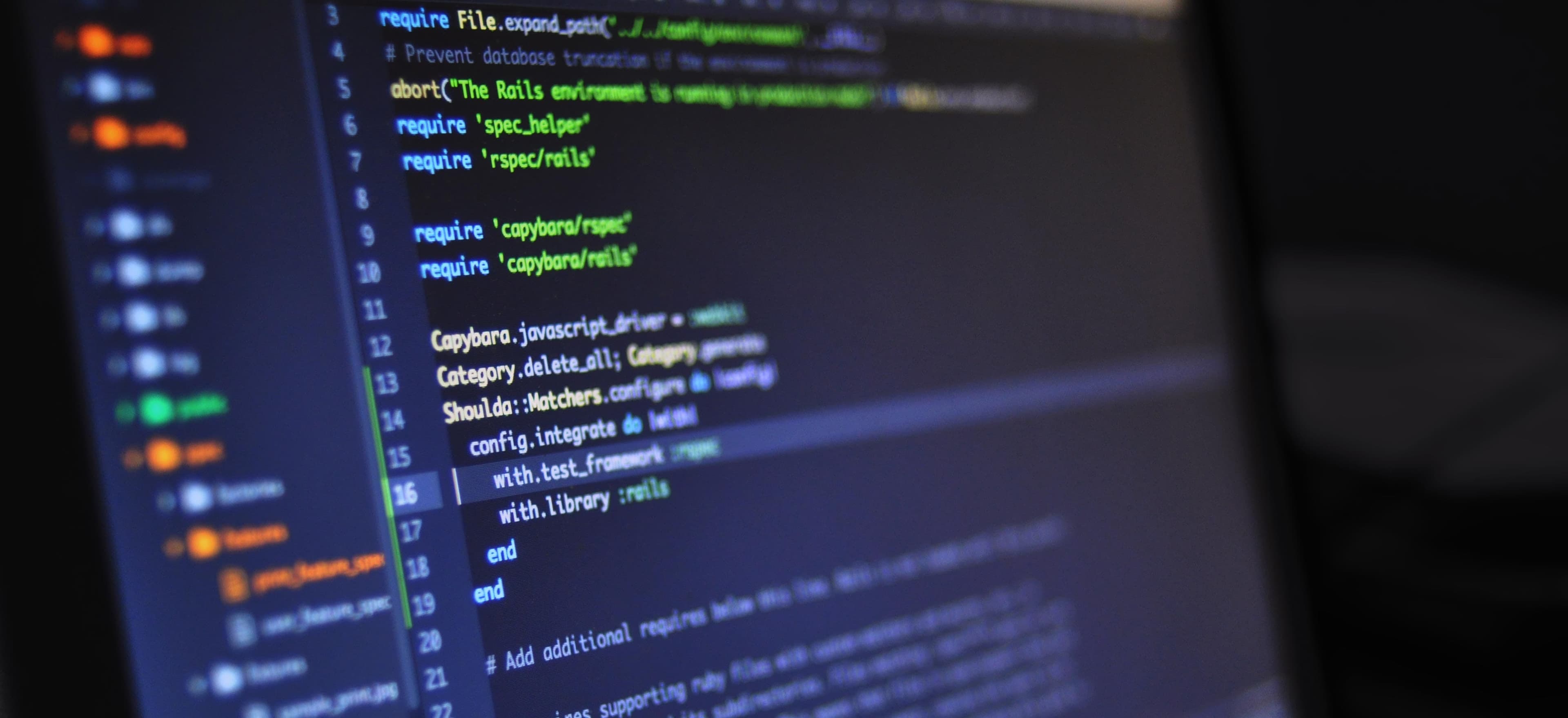
- Published on
Mastering Java: How to Ask the Right Questions in Code
In programming, asking the right questions can often lead you to the best solutions. Just as in tarot reading, where the inquiry is critical to interpret the cards effectively, in Java programming, the questions you pose—about your data, your structures, or your methods—guide your implementation strategy.
In this blog post, we'll explore the significance of asking the right questions in Java programming. We will delve into important concepts, provide practical code snippets, and discuss how they can enhance your coding practices. For those interested in understanding the importance of framing questions in a different context, you may find this article on Tarot cards insightful.
Table of Contents
- The Importance of Asking Questions
- How to Frame Questions in Java
- Example 1: Data Structures
- Example 2: Design Patterns
- Best Practices for Coding in Java
- Conclusion
The Importance of Asking Questions
When embarking on a new programming task, the questions you ask can shape your approach and determine the quality of your code. Questions help break down complex problems into manageable parts. They lead developers to explore various solutions, evaluate alternatives, and avoid potential traps.
In Java, asking the right questions is about understanding the problem fully before diving into your code. This practice can save time and effort and often leads to a more organized and clean codebase.
How to Frame Questions in Java
Example 1: Data Structures
When deciding which data structure to employ, the right questions will guide your choice.
Key Questions:
- What kind of data will I be storing?
- How often will the data be accessed and modified?
- Do I need sorted data?
Think of a scenario where we need to manage a list of student grades. Depending on the requirements, you might want to consider a List
, a Map
, or even a Set
.
Here's a code snippet demonstrating how to choose a Map
for storing student names associated with their grades:
import java.util.HashMap;
import java.util.Map;
public class StudentGrades {
private Map<String, Integer> grades;
public StudentGrades() {
this.grades = new HashMap<>();
}
public void addGrade(String name, int grade) {
grades.put(name, grade);
}
public Integer getGrade(String name) {
return grades.get(name);
}
public static void main(String[] args) {
StudentGrades sg = new StudentGrades();
sg.addGrade("Alice", 95);
System.out.println("Alice's Grade: " + sg.getGrade("Alice")); // Output: Alice's Grade: 95
}
}
Commentary:
In this example, we utilize a HashMap
to store students' names and grades. Here:
- Why a Map?: It allows us to retrieve grades efficiently by key (student name), which is crucial for performance when accessing data frequently.
- Why HashMap?: It provides average O(1) time complexity for get and put operations, making it suitable for our needs.
Example 2: Design Patterns
Another area where framing questions is vital is in design patterns. Consider the Singleton pattern, which ensures a class has only one instance.
Key Questions:
- Do I need a global point of access for this object?
- Is maintaining a single instance crucial for performance?
Here's how to implement a Singleton class in Java:
public class Singleton {
private static Singleton instance;
private Singleton() {
// private constructor
}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
public void displayMessage() {
System.out.println("Hello from Singleton!");
}
public static void main(String[] args) {
Singleton singleton = Singleton.getInstance();
singleton.displayMessage(); // Output: Hello from Singleton!
}
}
Commentary:
In this code:
- Private Constructor: Prevents instantiation from outside the class, ensuring a single instance.
- getInstance Method: Lazy instantiation is implemented to create the instance when it's first needed, efficiently handling resource allocation.
Best Practices for Coding in Java
- Keep It Simple: Avoid overcomplications. Aim for clarity.
- Modularization: Break your code into smaller, reusable functions.
- Document Your Code: Use comments and documentation to clarify your thought process.
- Ask for Feedback: Engaging with peers can help refine your code and thought process.
- Test Your Code: Regularly test for bugs—it’s vital for understanding how your solution works and how it can be improved.
The Value of Asking Questions in Code
The habits of asking questions lead to better decision-making and a deeper understanding of programming concepts. They prevent most developers from falling into the trap of simply coding without a clear strategy, resulting in inefficient and possibly error-prone solutions.
Final Thoughts
In the world of programming, much like in the mystic world of tarot readings, the quality of your inquiries shapes your understanding and decision-making process. By framing the right questions in Java—whether it be regarding data structures, design patterns, or best practices—you clear the path for better coding practices and more efficient solutions.
Remember to take inspiration from resources like the article titled Tarotkarten: So stellst du die perfekte Frage, where the quality of questions can lead to insightful outcomes.
By mastering the art of inquiry in your coding practices, you will not only enhance your programming skills but also pave the way for becoming a more proficient and thoughtful developer. Continue exploring, asking, and learning, and enjoy the journey through the intricate world of Java programming!