Java Solutions for Enhancing PWA Offline Capabilities
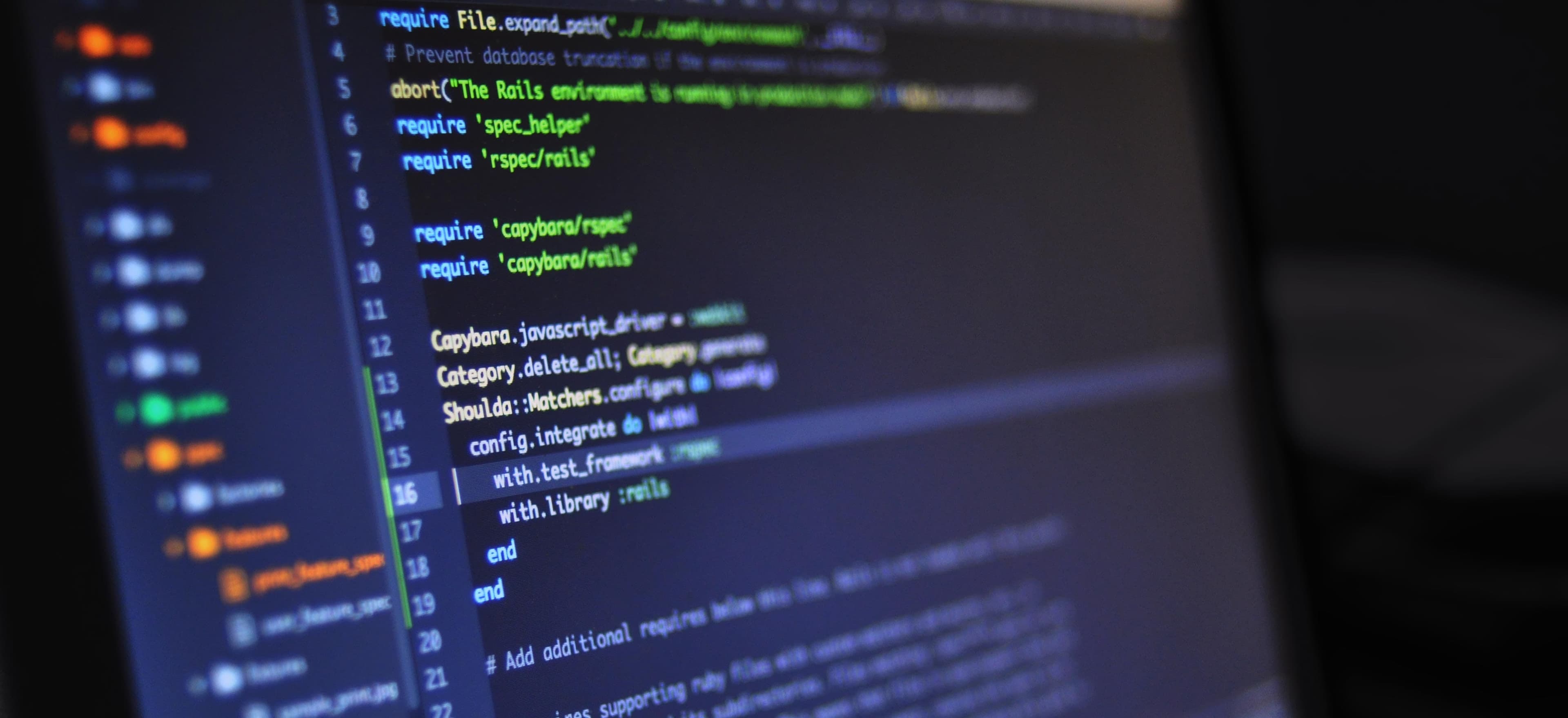
- Published on
Java Solutions for Enhancing PWA Offline Capabilities
Progressive Web Applications (PWAs) have transformed the way we experience web apps. They blend the best of both web and mobile apps, providing users with fast load times, offline access, and increased engagement. However, one challenge that developers face when building PWAs is managing offline capabilities. This blog post will delve into how you can leverage Java to enhance the offline capabilities of your PWA, ensuring users have seamless interactions even without an internet connection.
Why Offline Capabilities Matter
In an era where mobile connectivity can be spotty or congested, having a robust offline capability is crucial. Users expect applications to function seamlessly, regardless of their connection status. The offline capabilities empower users by allowing them to view content, make edits, and perform tasks that would otherwise require an internet connection.
In the article titled Overcoming Offline Challenges in PWAs with JavaScript, the author emphasizes various methods for achieving offline functionalities using JavaScript. However, Java can also be a powerful ally in this pursuit. By utilizing Java's strong backend services and capabilities, we can effectively address the challenges associated with offline operations in a PWA.
Setting Up Your PWA
Before diving into Java's role in improving offline capabilities, it is essential to understand the PWA's basic structure. A standard PWA consists of three main components:
- Service Workers: Scriptable network proxies that manage caching and offline access.
- Web App Manifest: Ensures the web app looks and feels like a native app on mobile devices.
- Responsive Design: Adapts the user interface to different screen sizes.
Establishing a Service Worker
Service workers are a key element of a PWA's offline capabilities. They intercept network requests and can cache responses, allowing your app to serve cached content when offline. Here’s a simplified service worker setup:
// sw.js - Service Worker File
self.addEventListener('install', (event) => {
event.waitUntil(
caches.open('v1').then((cache) => {
return cache.addAll([
'/',
'/index.html',
'/style.css',
'/app.js'
]);
})
);
});
self.addEventListener('fetch', (event) => {
event.respondWith(
caches.match(event.request).then((response) => {
return response || fetch(event.request);
})
);
});
Why This Matters
- Caching: The code above caches the essential files to ensure they are readily available even when offline.
- Fetch Interception: The fetch event handles requests. If a request is found in the cache, it serves that; otherwise, it goes online.
Now, while this is fundamental JavaScript work, integrating Java can bolster your backend services.
Using Java for Server-Side Caching
Integrating Java on the server-side can complement your PWA’s offline functionality by enabling backend caching mechanisms that can keep data available when offline. For instance, you could implement a RESTful service that stores user data in a local database or on the device while syncing it with your server when a connection is re-established.
Here’s a basic example using Spring Boot to create a RESTful service for user data:
@RestController
@RequestMapping("/api/users")
public class UserController {
@Autowired
private UserService userService;
@PostMapping
public ResponseEntity<User> createUser(@RequestBody User user) {
User createdUser = userService.saveUser(user);
return new ResponseEntity<>(createdUser, HttpStatus.CREATED);
}
@GetMapping
public List<User> getAllUsers() {
return userService.getAllUsers();
}
}
Why Java?
- Ease of Management: Java’s frameworks, like Spring, provide a structure for managing server-side logic.
- Integration With Databases: Java works well with various data stores, ensuring data can be persistently retrieved and sent when online.
Implementing Local Storage
While server-side caching is important, there are times when you want to store data directly on the client-side. JavaScript's localStorage
or IndexedDB
can be useful here for storing small to moderate amounts of data.
This is where your PWA can shine through Java's interplay with the front end. For instance, upon creating a user offline, you can store the data in localStorage
while queuing an HTTP request to send to the server when the user is online again.
Example
Here’s an example of user creation logic that taps into both frontend storage and backend services:
function saveUser(user) {
if (navigator.onLine) {
// If online, send to the server
fetch('/api/users', {
method: 'POST',
body: JSON.stringify(user),
headers: {
'Content-Type': 'application/json'
}
}).then(response => response.json())
.then(data => console.log('User saved:', data));
} else {
// If offline, save to localStorage
const users = JSON.parse(localStorage.getItem('users')) || [];
users.push(user);
localStorage.setItem('users', JSON.stringify(users));
console.log('User saved to local storage:', user);
}
}
The Importance of This Example
- Adaptive Design: This function checks the user’s online status and responds accordingly.
- User Experience: Users can still interact with the PWA even when connectivity is limited.
Offline Sync Strategies
For robust offline capabilities, a strategy for syncing data when a connection is restored is crucial. You may consider implementing a queue system that batches requests when the user reconnects.
Example of Sync Function
Here’s a simplistic approach to sync local changes when the user gets back online:
function syncUsers() {
const users = JSON.parse(localStorage.getItem('users')) || [];
for (const user of users) {
fetch('/api/users', {
method: 'POST',
body: JSON.stringify(user),
headers: {
'Content-Type': 'application/json'
}
}).then(response => {
// Removing successfully synced user from localStorage
if (response.ok) {
// Perform cleanup
onUserSynced(user);
}
});
}
}
function onUserSynced(user) {
let users = JSON.parse(localStorage.getItem('users'));
users = users.filter(u => u.id !== user.id); // Assuming user has a unique ID
localStorage.setItem('users', JSON.stringify(users));
}
Why Syncing is Essential
- Data Consistency: Improves reliability by ensuring the data across online and offline states aligns.
- User Trust: Users appreciate knowing their actions aren’t lost due to connectivity issues.
Wrapping Up
Building a PWA with enhanced offline capabilities requires a blend of technologies and strategies. By leveraging Java's powerful backend services alongside client-side JavaScript solutions, you can create a seamless user experience.
Your users are bound to appreciate a PWA that remains functional in various network conditions, driving engagement and satisfaction. With the strategies discussed in this post and a careful approach to the integration of Java and JavaScript, you can effectively tackle the offline challenges in your PWAs.
For additional insights on managing offline functionality with JavaScript, be sure to refer to the article on Overcoming Offline Challenges in PWAs with JavaScript.
By taking advantage of both Java backend services and frontend technologies, you enhance your PWA’s capabilities dramatically. Adapt to technological advancements and user expectations, and your PWA will resonate well with its audience.