Mastering Offline Capabilities in Java Apps with PWAs
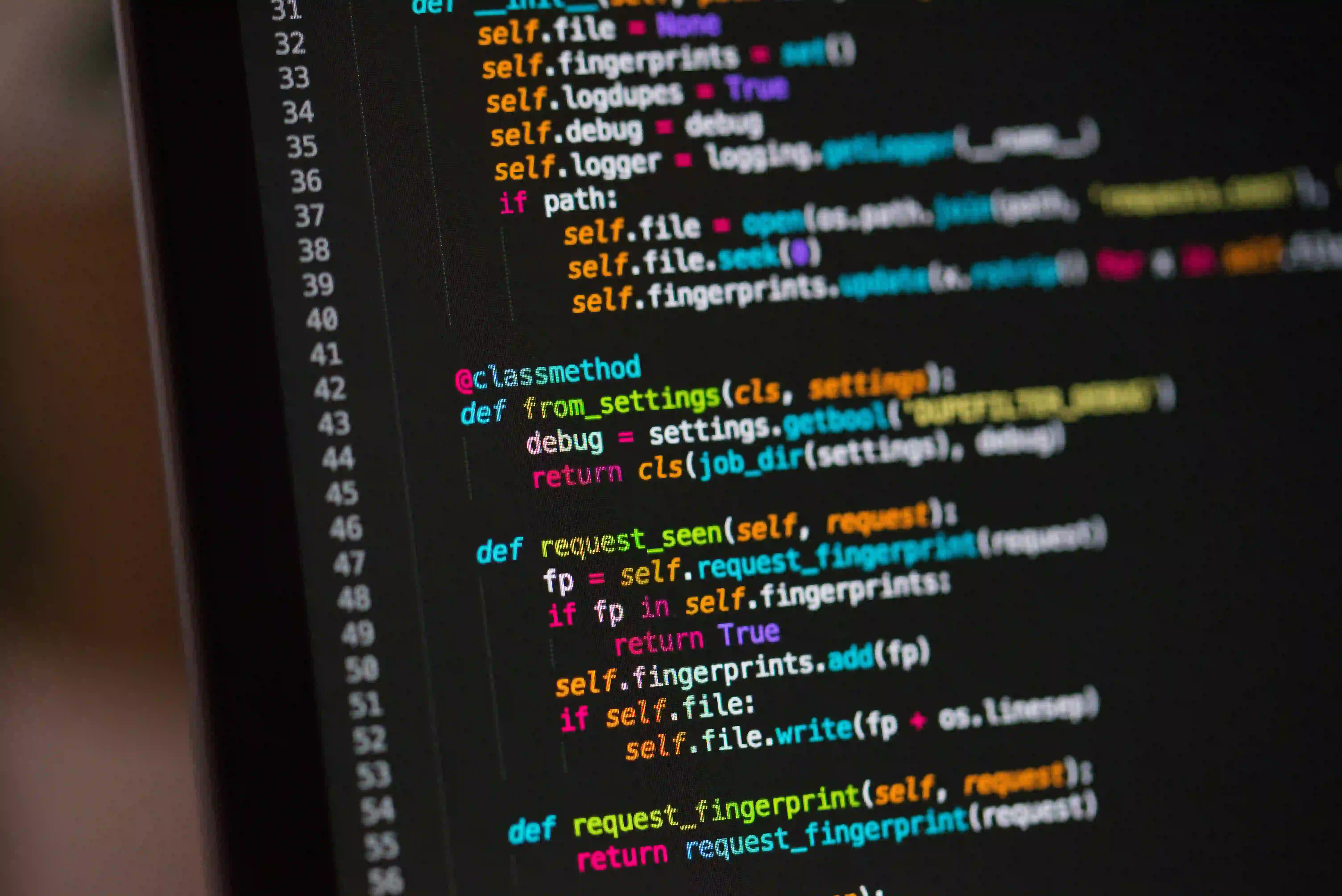
Mastering Offline Capabilities in Java Apps with PWAs
In a world where connectivity is anything but guaranteed, Progressive Web Apps (PWAs) have emerged as a solution to enhance user experiences, especially in offline scenarios. This post delves into the intricacies of building Java applications that leverage PWA technology to maintain functionality even when users are offline.
What Are Progressive Web Apps?
PWAs are web applications that utilize modern web capabilities to deliver an app-like experience to users. These applications are fast, reliable, and engaging. They employ service workers, a critical aspect of their architecture, which allow them to intercept network requests and cache content, creating offline capabilities.
Why PWAs Matter
- User Retention: With offline capabilities, users can still interact with the app, which reduces bounce rates.
- Performance: PWAs load quickly, even on slow networks, enhancing user experience.
- Accessibility: Users can access the app from various devices and platforms, not limited to a specific operating system.
Setting Up Your Java PWA
To get started, ensure that your Java development environment is properly configured. You’ll need:
- Java Development Kit (JDK): Ensure you have the latest version.
- A Web Framework: Spring Boot is a popular choice that simplifies the development of web applications in Java.
- Frontend Technologies: Familiarity with HTML, CSS, and JavaScript is essential for PWA implementation.
Initializing Your Java Project with Spring Boot
Here’s how to set up a basic Spring Boot application:
@SpringBootApplication
public class PwaApplication {
public static void main(String[] args) {
SpringApplication.run(PwaApplication.class, args);
}
}
Why Spring Boot?
Spring Boot simplifies the process of setting up and configuring Java web applications. It uses an opinionated approach to reduce boilerplate code.
Project Structure
Adopt a clear structure for your PWA project:
pwa-application/
│
├── src/
│ ├── main/
│ │ ├── java/
│ │ └── resources/
│ └── test/
└── pom.xml
Adding PWA Features
1. Service Workers
Service Workers act as a proxy between your web application and the network. They enable caching and background data synchronization.
Here’s a basic service worker example:
self.addEventListener('install', (event) => {
console.log('Service Worker installing.');
event.waitUntil(
caches.open('v1').then((cache) => {
return cache.addAll([
'/',
'/index.html',
'/styles.css',
'/app.js'
]);
})
);
});
Explanation: During the installation phase, the service worker caches necessary resources, ensuring that they are available offline.
2. Caching Strategies
Deploying the right caching strategy is crucial for maximizing offline performance. Here’s a popular strategy called "Cache First":
self.addEventListener('fetch', (event) => {
event.respondWith(
caches.match(event.request).then((response) => {
return response || fetch(event.request);
})
);
});
Why Use Cache First?
This approach first looks for resources in the cache, resulting in faster load times and reducing requests to the network.
3. Web App Manifest
The Web App Manifest is a JSON file that provides information about your application, like name, icons, and display mode. Here’s a sample manifest:
{
"name": "My Java PWA",
"short_name": "JavaPWA",
"start_url": "/",
"display": "standalone",
"icons": [
{
"src": "images/icon-192x192.png",
"sizes": "192x192",
"type": "image/png"
},
{
"src": "images/icon-512x512.png",
"sizes": "512x512",
"type": "image/png"
}
]
}
Importance of the Manifest: The manifest enables the app to be installed on the device’s home screen, thereby offering a native app experience.
4. Offline Fallback Pages
It's advisable to implement an offline fallback page to enhance the user experience during connectivity issues. Create a simple HTML page to inform users of the offline status:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Offline</title>
</head>
<body>
<h1>You are Offline</h1>
<p>Check your internet connection and try again.</p>
</body>
</html>
Conclusion on Fallback Pages: Providing clear communication when offline is key to maintaining user trust.
5. Syncing Data When Back Online
Minimizing data loss when transitioning between offline and online modes is a common challenge. Here’s an approach using the Background Sync API:
self.addEventListener('sync', (event) => {
if (event.tag === 'sync-data') {
event.waitUntil(syncData());
}
});
async function syncData() {
const data = await getDataFromIndexedDB();
await fetch('/api/data', {
method: 'POST',
body: JSON.stringify(data),
});
}
Why Background Sync?
This allows you to defer actions until the user has a stable internet connection, thus ensuring data consistency.
Real-World Application
For practical insights on improving offline capabilities, check out the article “Overcoming Offline Challenges in PWAs with JavaScript” found at infinitejs.com/posts/overcoming-offline-challenges-pwas-javascript. This resource dives deeper into strategies specifically for JavaScript, offering valuable lessons adaptable for Java-based PWAs.
Bringing It All Together
Implementing PWAs in Java allows you to deliver robust offline capabilities, ensuring seamless user experiences regardless of connectivity issues. By leveraging service workers, caching strategies, and effective data synchronization techniques, you can create an engaging application that provides value to users even in challenging network conditions.
As you embark on building your PWA with Java, remember that user experience is paramount. A well-implemented offline mode not only enhances usability but also builds a loyal user base. Happy coding!