How Java Can Enhance Image Title Handling in Browsers
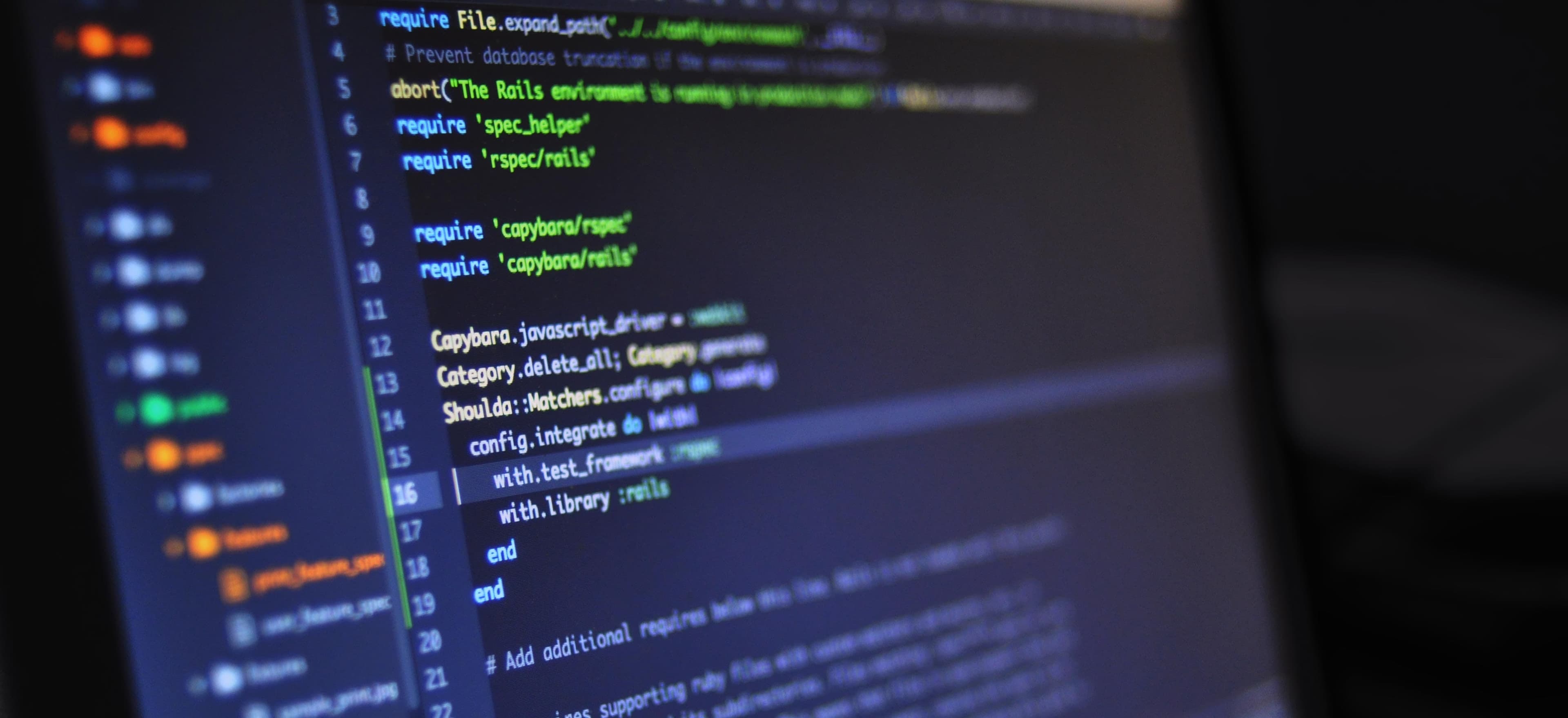
- Published on
How Java Can Enhance Image Title Handling in Browsers
Modern web applications rely heavily on images, not only for aesthetics but also for functional purposes. As a developer, ensuring that images are displayed correctly and that users have a seamless experience is vital. One common issue that web developers face is how images are titled and displayed in browsers. This becomes especially pertinent when dealing with user-uploaded images or dynamically generated content.
If you've encountered issues with Chrome's image title display, you might want to check out the article titled Struggling with Chrome's Image Title Display? Fix It!. Here, we'll discuss how leveraging Java on the server side can improve how images and their titles are managed in browsers, ensuring a better user experience.
Understanding Image Title Display Issues
Before diving into how Java can help, let's explore some common image title display issues that arise in browsers.
- Inconsistent Display: Different browsers may handle image titles inconsistently. What looks good on one browser might appear broken on another.
- User Experience: Titles are often displayed when users hover over images. If the titles are missing, vague, or inaccurate, it can detract from user experience.
- SEO Implications: Proper image titles contribute to search engine optimization (SEO). When browsers fail to display them correctly, it can negatively impact SEO.
The Role of Java
Java, a versatile programming language, is often used in web development for backend processes. It can significantly enhance how image titles are handled, ensuring they are accurate and displayed consistently across browsers. Below are some proven methods to achieve this.
1. Managing Image Metadata
In Java, you can manage image metadata, including titles, using libraries like Apache Commons Imaging. This enables you to embed essential information directly into the image file.
Example Code Snippet
import org.apache.commons.imaging.Imaging;
import org.apache.commons.imaging.ImageMetadata;
import org.apache.commons.imaging.ImageMetadata.Item;
import java.io.File;
import java.io.IOException;
public class ImageTitleManager {
public static void setImageTitle(File imageFile, String title) {
try {
ImageMetadata metadata = Imaging.getMetadata(imageFile);
// Adding the title as a new metadata item
metadata.addItem(new Item("Title", title));
// Save the metadata back to the image file
Imaging.writeImageWithMetadata(imageFile, Imaging.getBufferedImage(imageFile), null, metadata);
System.out.println("Title set successfully: " + title);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Commentary
This code snippet demonstrates how to embed a title into an image file using Apache Commons Imaging. By managing image metadata on the server side, web developers can ensure that image titles are preserved, improving the experience across different browsers.
2. Dynamic Title Generation
For dynamically generated images—such as those created by users through forms or applications—Java can be employed to programmatically generate titles based on user input or image content.
Example Code Snippet
public class DynamicTitleGenerator {
public static String generateTitle(String baseTitle, String userId) {
return baseTitle + " - Uploaded by User: " + userId + " on " + java.time.LocalDate.now();
}
}
Commentary
This code generates a dynamic title for images. By including the user's ID and the upload date, it personalizes the image title, which can improve user engagement. This practice not only helps users remember their uploaded content but also aids in organizing images efficiently.
3. Using Java Servlets for Image Handling
Java Servlets can be instrumental in handling image uploads. With a servlet, you can process the uploaded image and its title, ensuring the title is correctly stored and retrieved.
Example Code Snippet
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.Part;
@WebServlet("/uploadImage")
public class ImageUploadServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String title = request.getParameter("title");
Part filePart = request.getPart("image");
// Save the image file and its title
// Assume saveImageFile() is a method that stores the image
saveImageFile(filePart, title);
response.getWriter().println("Image uploaded successfully with title: " + title);
}
private void saveImageFile(Part filePart, String title) {
// Implementation to save the image file on the server
}
}
Commentary
In this servlet example, we process an image upload and its associated title. This architecture allows you to maintain a clear separation of concerns while adequately handling image data. By ensuring that titles are stored alongside the images, you can facilitate better workflows and enhance users' experiences.
4. Validating and Sanitizing Titles
It's essential to validate and sanitize the image titles that come from user input to prevent issues like XSS (Cross-Site Scripting) attacks or injection attacks.
Example Code Snippet
import java.util.regex.Pattern;
public class TitleValidator {
private static final Pattern TITLE_PATTERN = Pattern.compile("^[a-zA-Z0-9\\s]+$");
public static boolean isTitleValid(String title) {
return TITLE_PATTERN.matcher(title).matches();
}
}
Commentary
This code provides a basic validation for image titles using regular expressions. Ensuring that titles are valid helps maintain security and data integrity, which is a top priority for web applications.
Final Thoughts
In today's web development landscape, managing image titles effectively can significantly improve user experience and optimize SEO. Using Java and the various libraries and techniques discussed in this article, developers can ensure that image titles are accurately stored, dynamically generated, and displayed consistently across different browsers.
Navigating the complexities of web development can be challenging, especially regarding aspects like image title handling. If you're facing issues with image titles in Chrome, consider reading the article Struggling with Chrome's Image Title Display? Fix It! for more insights.
By applying these Java strategies, you're not only improving how your web application interacts with images but also creating a more robust, user-centered platform. As you continue to develop and optimize your applications, don’t hesitate to explore additional Java resources and libraries that can further enhance your image handling capabilities.