Avoiding Scalability Pitfalls in Java Microservices
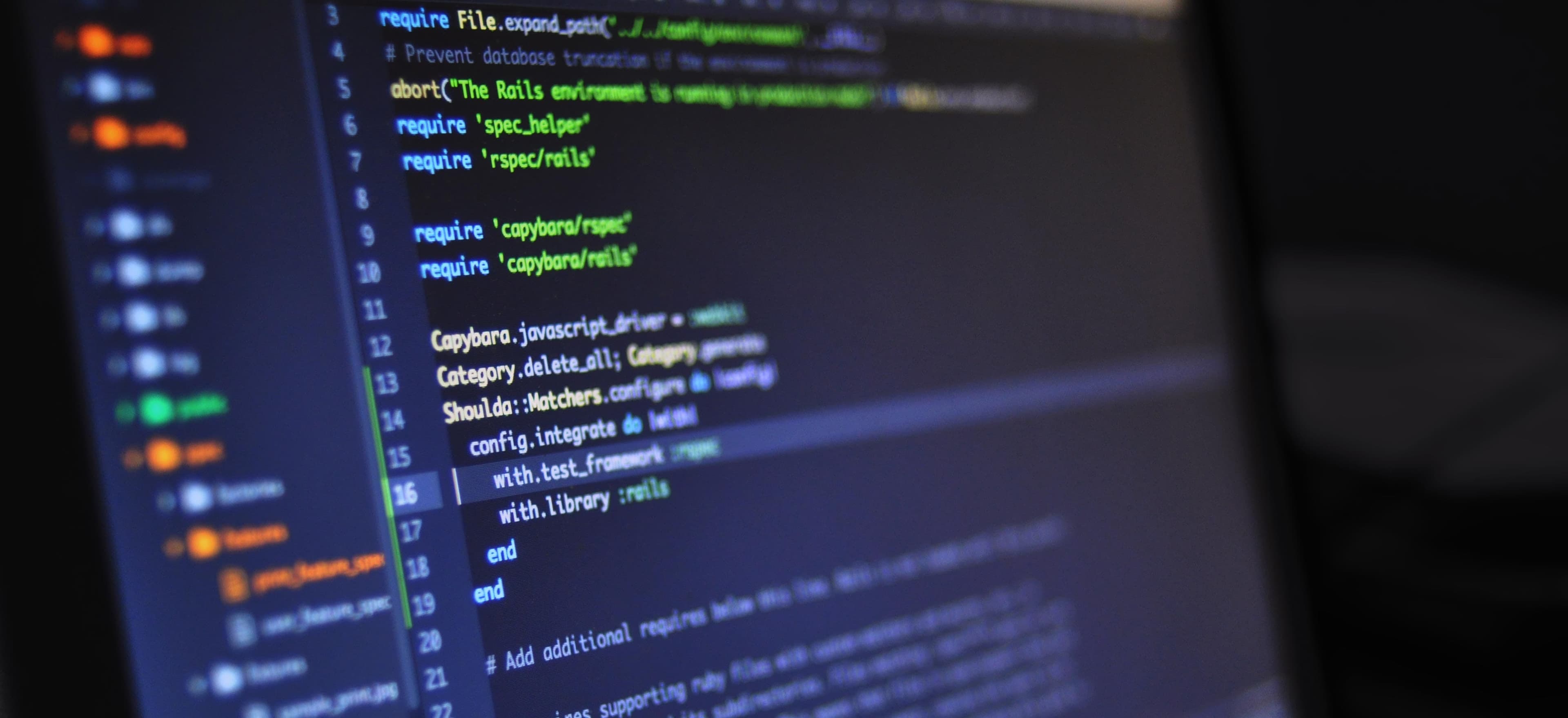
- Published on
Avoiding Scalability Pitfalls in Java Microservices
In the ever-evolving world of software development, building scalable applications is a priority for companies aiming to meet increasing user demands. While Node.js has gained popularity for its ability to handle concurrent requests, Java remains a powerhouse for enterprise applications, especially when it comes to microservices architecture. In this blog post, we will explore best practices for avoiding scalability pitfalls specifically in Java microservices. For a broader understanding of scalability issues, check out this article on Common Mistakes in Building Scalable Node.js Apps, which discusses strategies that can also apply to Java.
Understanding Microservices Architecture
Before diving into the specifics of scalability in Java microservices, let's briefly define what microservices are.
Microservices is an architectural style where an application is divided into small, independent services that can be deployed and operated separately. This approach allows teams to develop, deploy, and scale each service independently, making it a popular choice in modern software developments.
Why Java for Microservices?
Java provides robust features suitable for building scalable and maintainable microservices. With popular frameworks like Spring Boot, the development process becomes streamlined, allowing developers to focus on writing code rather than configuration. Moreover, Java's strong static typing, extensive ecosystem, and community support facilitate robust application development.
Common Scalability Pitfalls in Java Microservices
While building microservices can lead to enhanced scalability, certain pitfalls can hinder performance. Let's look at how to avoid these issues.
1. Ineffective Resource Management
Problem
Many developers overlook resource management, leading to resource leaks, particularly with database connections and file I/O. These leaks can deplete server resources, resulting in slower response times and eventual application crashes.
Solution
Use connection pooling to manage database connections effectively. A connection pool limits the total number of database connections created and reuses existing connections, helping to reduce overhead.
import javax.sql.DataSource;
import org.apache.commons.dbcp2.BasicDataSource;
public class DataSourceConfig {
public DataSource dataSource() {
BasicDataSource ds = new BasicDataSource();
ds.setUrl("jdbc:mysql://localhost:3306/mydb");
ds.setUsername("user");
ds.setPassword("password");
// Set pool size
ds.setInitialSize(5);
ds.setMaxTotal(20);
return ds;
}
}
Why: By managing database connections effectively, you enhance application responsiveness and prevent resource exhaustion. The aforementioned code ensures a limited number of database connections are created, optimizing performance.
2. Synchronous Communication
Problem
Relying on synchronous communication between microservices can lead to performance bottlenecks. If one service lags, dependent services may also slow down, leading to sluggish response times.
Solution
Implement asynchronous communication wherever possible. Java's CompletableFuture or message brokers like RabbitMQ can help segregate service calls.
import java.util.concurrent.CompletableFuture;
public class UserService {
public CompletableFuture<User> getUserAsync(Long userId) {
return CompletableFuture.supplyAsync(() -> {
// Simulates a database operation
return database.findUserById(userId);
});
}
}
Why: Asynchronous communication uncouples service dependencies, allowing independent operations. This promotes better resource utilization while enhancing overall responsiveness.
3. Tight Coupling
Problem
Creating microservices that are too dependent on each other can lead to a monolithic-like structure. Tight coupling can hinder independent scaling and slow down development.
Solution
Aim for loose coupling by defining clear interfaces and using API gateways. Consider using technologies like Spring Cloud to facilitate inter-service communication.
@RestController
@RequestMapping("/api/users")
public class UserController {
private final UserService userService;
public UserController(UserService userService) {
this.userService = userService;
}
@GetMapping("/{id}")
public ResponseEntity<User> getUser(@PathVariable Long id) {
User user = userService.findById(id);
return ResponseEntity.ok(user);
}
}
Why: This controller defines a clear interface for interacting with the UserService, promoting loose coupling. Changes in UserService will not drastically impact the UserController, allowing independent modifications.
4. Ignoring Distributed Tracing
Problem
Debugging microservices can be challenging due to their distributed nature. If you don't implement logging and monitoring, identifying bottlenecks becomes problematic.
Solution
Use distributed tracing tools like Spring Cloud Sleuth or Zipkin. These tools allow you to visualize requests as they pass through your microservices.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@Service
public class ProductService {
private static final Logger logger = LoggerFactory.getLogger(ProductService.class);
public Product getProductById(Long productId) {
logger.info("Fetching product with ID: {}", productId);
// Business logic
return productRepository.findById(productId);
}
}
Why: Logging helps in tracking issues and optimizing performance across microservices. With proper tracing, you gather essential data that assists in diagnosing performance problems.
5. Scaling Issues with Statefulness
Problem
Microservices should ideally be stateless. Making them stateful can create challenges for scalability, especially in load-balanced environments.
Solution
Avoid storing state within your microservices. Instead, use external storage solutions like Redis or database systems for state management.
@Service
public class CartService {
private final RedisTemplate<String, Cart> redisTemplate;
public CartService(RedisTemplate<String, Cart> redisTemplate) {
this.redisTemplate = redisTemplate;
}
public void addToCart(String userId, Product product) {
Cart cart = redisTemplate.opsForValue().get(userId);
cart.addProduct(product);
redisTemplate.opsForValue().set(userId, cart);
}
}
Why: By leveraging external storage, you make your services stateless, enhancing scalability and simplifying load distribution across your service cluster.
6. Underestimating Testing and Performance Benchmarking
Problem
Failing to perform adequate testing can lead to unforeseen scalability issues once components are in production.
Solution
Implement rigorous unit tests and performance benchmarks using tools like JMeter. Monitoring tools can provide insights into performance metrics that help you identify slow operations.
@Test
public void testAddToCartPerformance() throws Exception {
// Setup
long startTime = System.currentTimeMillis();
// Execution
cartService.addToCart("userId", new Product("productId"));
// Assertions
long elapsedTime = System.currentTimeMillis() - startTime;
assertTrue(elapsedTime < 1000); // Ensure the operation is performed in under a second
}
Why: Testing early and often helps identify performance issues before they impact users, thereby enhancing application stability.
Final Thoughts
Building scalable Java microservices involves navigating through various pitfalls. By focusing on effective resource management, asynchronous communication, loose coupling, distributed tracing, managing state, and rigorous testing, your microservices architecture can thrive.
Throughout this article, we’ve highlighted essential strategies to build performant Java microservices. For a deeper understanding of scalability challenges across different technologies, consider exploring Common Mistakes in Building Scalable Node.js Apps.
The world of microservices offers limitless possibilities, and understanding how to avoid common pitfalls will ensure your Java applications can grow smoothly alongside user expectations. Happy coding!
Checkout our other articles