Avoid These Common Pitfalls When Scaling Java Applications
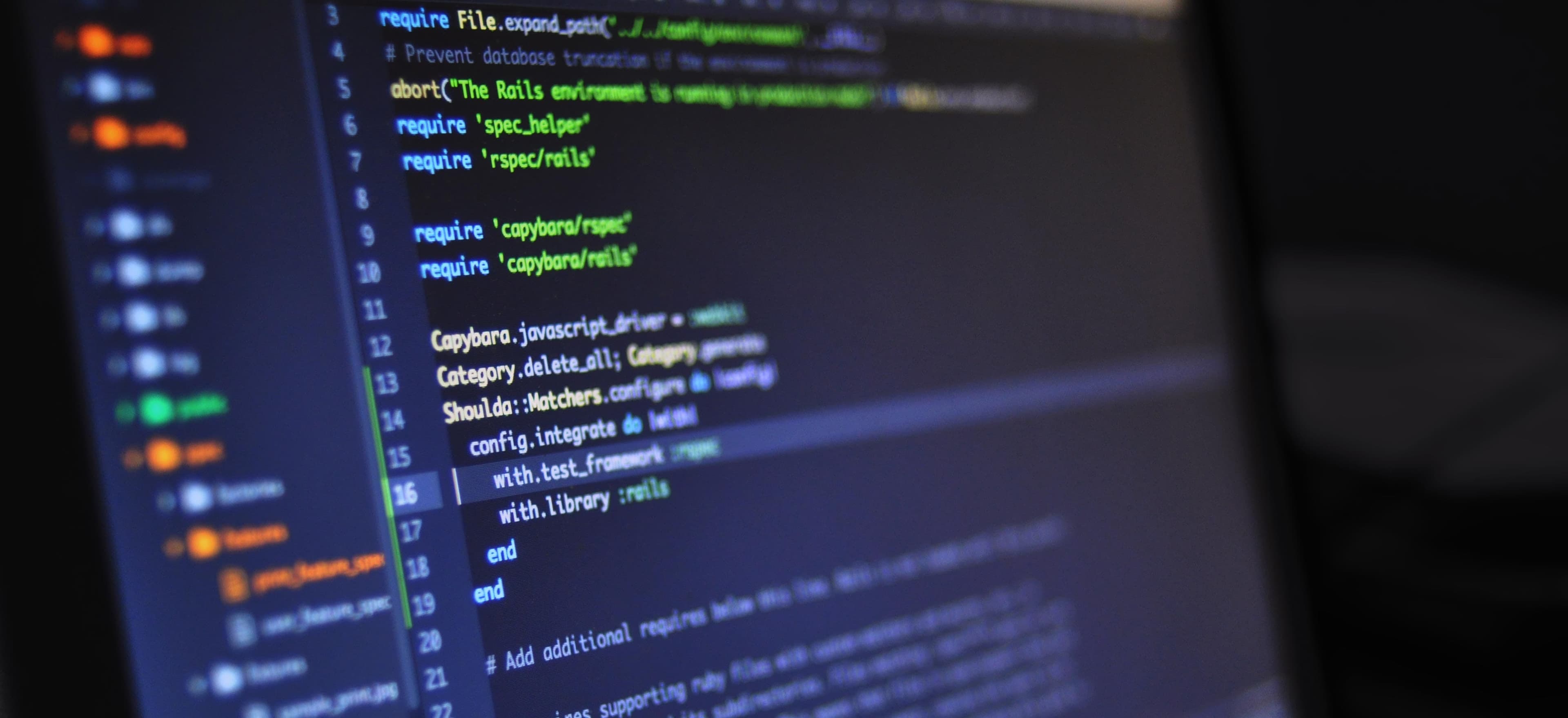
- Published on
Avoid These Common Pitfalls When Scaling Java Applications
Java has long been the go-to language for building scalable applications. Its robust frameworks, extensive libraries, and platform independence make it a favorite among developers. However, like any other technology, it comes with its set of challenges, especially when it comes to scaling. In this article, we will explore common pitfalls in scaling Java applications and provide practical solutions to avoid them.
Understanding Scalability in Java
Scalability is the ability of an application to handle a growing amount of work, or its potential to accommodate growth efficiently. This can be achieved in two primary ways:
- Vertical Scaling (Scaling Up): Adding more resources (CPU, RAM) to a single machine.
- Horizontal Scaling (Scaling Out): Adding more machines to distribute the load.
With Java, both methods can be employed effectively, but they each come with their own sets of challenges.
Pitfall 1: Ignoring Performance Testing
Performance testing is crucial for understanding how your application behaves under various loads. Many developers skip this step, thinking their application will handle anything thrown at it.
Solution: Implement Testing Early
Utilize tools such as JMeter or Gatling for load testing. By simulating multiple users, you can identify bottlenecks and optimize your code before production.
// Example of a simple JUnit test for performance
@Test
public void testPerformance() {
long startTime = System.currentTimeMillis();
performHeavyOperation(); // Simulate a heavy operation
long endTime = System.currentTimeMillis();
assertTrue("Performance test failed", (endTime - startTime) < 1000);
}
In this snippet, we measure the time taken to execute a hypothetical heavy operation. The key is to set reasonable threshold values based on your application’s needs, ensuring that the performance remains within acceptable limits.
Pitfall 2: Not Optimizing Database Queries
Inefficient database queries can lead to immense delays and ultimately bottleneck your application. Transactions that take too long can cripple your service and degrade user experience.
Solution: Use ORM Wisely
Java applications often leverage Object-Relational Mapping (ORM) frameworks like Hibernate. While these can simplify database interactions, misuse or over-reliance on them can result in performance issues.
Session session = sessionFactory.openSession();
Transaction transaction = session.beginTransaction();
List<Employee> employees = session.createQuery("FROM Employee", Employee.class).list();
transaction.commit();
session.close();
While the above Hibernate code is simple, fetching all records at once can be inefficient. Use pagination and lazy loading to ensure only necessary data is loaded.
Pitfall 3: Neglecting Caching Strategies
In the rush to build features, caching mechanisms may be overlooked. Without caching, your application might be performing redundant work, querying the database for information that rarely changes.
Solution: Implement Effective Caching
You can use caching solutions like Redis or Ehcache to avoid repeated database access for frequently-requested data.
public class CacheService {
private Map<String, Object> cache = new HashMap<>();
public Object getFromCache(String key) {
return cache.getOrDefault(key, null);
}
public void putInCache(String key, Object value) {
cache.put(key, value);
}
}
This simple cache service stores key-value pairs in memory. The benefit is that it significantly reduces the number of database hits, enhancing performance.
Pitfall 4: Poor Thread Management
Thread management in Java can be tricky. An application using too many threads can lead to context switching overhead and degrade performance. Conversely, using too few threads may result in underutilization of system resources.
Solution: Utilize Java Concurrency Tools
Java provides several concurrent libraries and utilities. The Executor framework is a great starting point.
ExecutorService executorService = Executors.newFixedThreadPool(10);
executorService.execute(() -> {
// Your concurrent task here
});
executorService.shutdown();
Using a thread pool minimizes the overhead of creating threads and can improve application throughput.
Pitfall 5: Overcomplicated Microservices Architecture
While microservices can enhance modularity, a poorly designed architecture can lead to unnecessary complexity. For Java applications, not having a proper understanding of service communication can create problems.
Solution: Clear API Contracts
Maintain well-defined API contracts between services. Utilize OpenAPI or Swagger to define endpoints clearly, enhancing understandability and reducing integration issues.
Pitfall 6: Not Utilizing Asynchronous Processing
Synchronous processing can lead to longer response times and user dissatisfaction. Not making use of asynchronous processing can limit your application’s responsiveness.
Solution: Leverage Asynchronous Methods
Java’s CompletableFuture class allows you to run tasks asynchronously without blocking the main thread.
CompletableFuture.supplyAsync(() -> {
// Your heavy task here
}).thenAccept(result -> {
// Process the result
});
Using asynchronous tasks can help your application respond to user requests more rapidly, improving overall user experience.
Pitfall 7: Failing to Monitor Application Performance
Monitoring applications in production environments is crucial for maintaining performance. Without it, identifying and resolving issues would be nearly impossible.
Solution: Implement Monitoring Tools
Use tools such as Spring Boot Actuator or Micrometer to gather metrics and health information about your application.
@RestController
public class MonitoringController {
@GetMapping("/metrics")
public ResponseEntity<Metrics> getMetrics() {
return ResponseEntity.ok(monitoringService.getMetrics());
}
}
Monitoring allows you to understand how your application behaves in real-time. You can also set alerts for critical performance degradation, enabling proactive maintenance.
Closing the Chapter
Scaling Java applications comes with its own unique set of challenges, making it crucial to understand the common pitfalls. By applying the solutions outlined above, from performance testing to efficient thread management, you can significantly improve your application’s scalability.
To deepen your knowledge further, consider reading Common Mistakes in Building Scalable Node.js Apps. While Node.js and Java differ in many ways, the principles of scalability often parallel across different programming environments, providing valuable insights regardless of the language employed.
By continuously optimizing and refining your Java applications, you ensure they remain robust, efficient, and ready to handle future growth. Happy coding!
Checkout our other articles