Avoiding Conditional Logic Pitfalls in Java with Best Practices
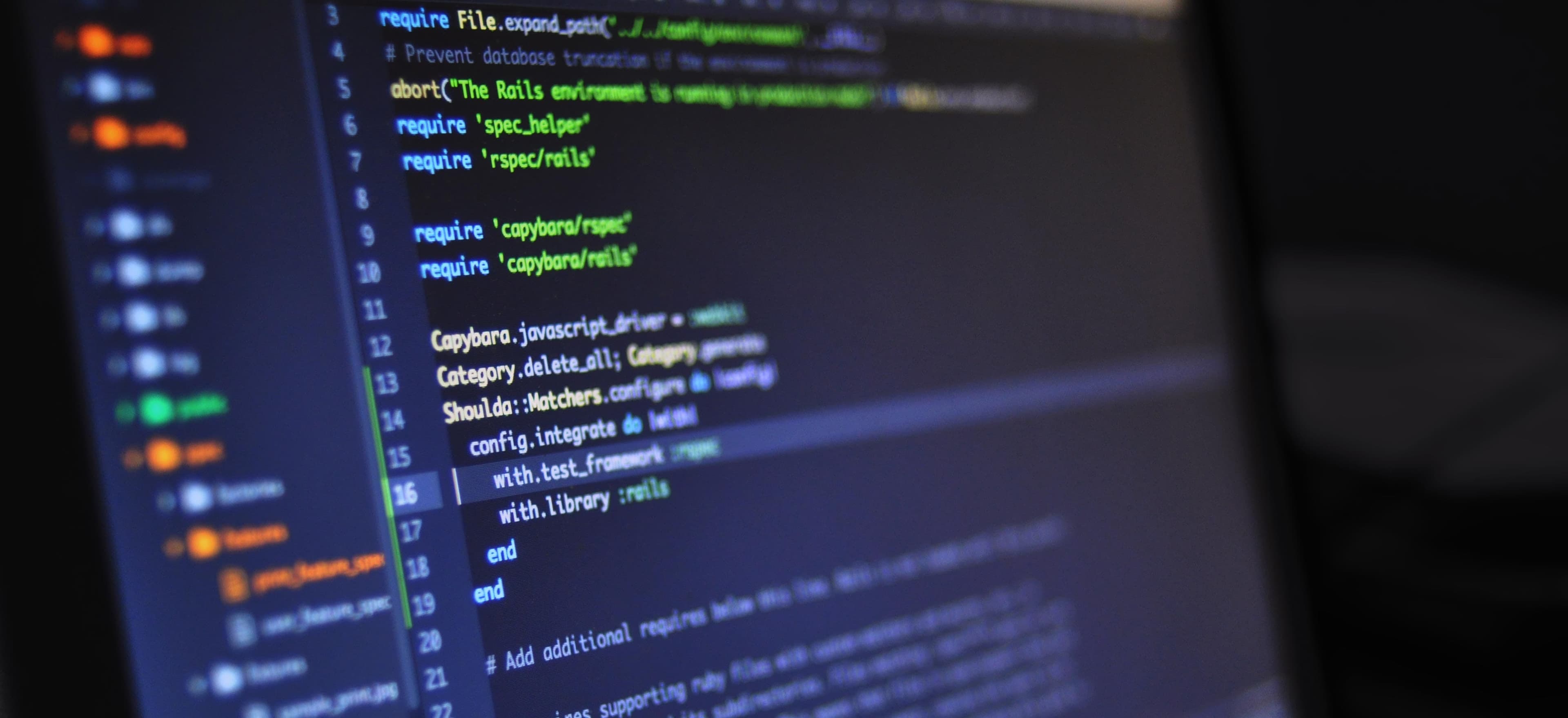
- Published on
Avoiding Conditional Logic Pitfalls in Java with Best Practices
Java is a robust, versatile programming language that is widely used for developing various applications—from web servers to mobile apps. One of the fundamental concepts in programming is conditional logic, permitting your code to make decisions based on given conditions. However, improper handling of conditional statements can lead to pitfalls that affect code readability and maintainability.
In this blog post, we will explore some of the best practices for managing conditional logic in Java. We'll dive deep into common mistakes, practical examples, and how to implement conditional statements effectively. We’ll also draw some parallels with JavaScript, referencing the article “Common Mistakes When Adding Conditions in JavaScript” found at tech-snags.com/articles/common-mistakes-adding-conditions-javascript.
Understanding Conditional Logic
Conditional logic allows a program to execute different code based on certain conditions. In Java, this is typically done using if
, else
, and switch
statements.
The Basic Syntax
Here’s a simple example:
int number = 10;
if (number > 0) {
System.out.println("The number is positive.");
} else if (number < 0) {
System.out.println("The number is negative.");
} else {
System.out.println("The number is zero.");
}
In this example, the logical conditions check the value of the variable number
. It’s a straightforward implementation, showing the foundation of conditional expressions. However, with increasing complexity comes the risk of unclear logic and unintended bugs.
Common Pitfalls in Conditional Logic
1. Nested Conditionals
One common mistake is overusing nested conditionals, which can make code harder to read and maintain. When you nest more than two conditions, the logic becomes convoluted.
Instead of:
if (condition1) {
if (condition2) {
// Logic
}
}
Consider using boolean variables or methods to simplify:
boolean isCondition1Met = checkCondition1();
boolean isCondition2Met = checkCondition2();
if (isCondition1Met && isCondition2Met) {
// Logic
}
This approach reduces nesting and enhances readability.
2. Using Magic Numbers
Magic numbers are numerical constants in your code that lack context, making it challenging to understand their purpose.
Instead of:
if (value == 42) {
// Logic
}
Define a constant:
public static final int ANSWER_TO_LIFE = 42;
if (value == ANSWER_TO_LIFE) {
// Logic
}
Using descriptive constants clarifies your code by providing context for the values being compared.
3. Inefficient switch
Statements
Java switch
statements can become cumbersome if not utilized efficiently. If your cases involve complex conditions, consider using a more streamlined format.
For example:
switch (color) {
case "red":
// Logic
break;
case "blue":
// Logic
break;
case "green":
// Logic
break;
default:
// Logic
}
This structure may lead to multiple comparisons. Instead, leverage a Map
for cleaner code:
Map<String, Runnable> colorActions = new HashMap<>();
colorActions.put("red", () -> {/* Logic for red */});
colorActions.put("blue", () -> {/* Logic for blue */});
colorActions.put("green", () -> {/* Logic for green */});
colorActions.getOrDefault(color, () -> {/* Logic for default */}).run();
This makes your code more fluid and maintainable, particularly as the number of conditions grows.
Best Practices for Handling Conditional Logic
1. Use Early Returns
Avoid deep nesting by implementing early returns in your methods. This can drastically enhance readability.
For example, instead of:
if (condition) {
// Logic
} else {
// Logic
}
Utilize:
if (!condition) {
return;
}
// Logic
This reduces complexity and keeps the fundamental logic at the forefront.
2. Prefer Immutability
Many issues arise from changing state all over your application. By favoring immutable data structures and returns from conditional checks, you can reduce bugs.
Consider this:
public String getStatus(int score) {
String status;
if (score >= 90) {
status = "A";
} else if (score >= 80) {
status = "B";
} else {
status = "C";
}
return status;
}
In this case, we declare the status
variable right before its use. It makes logical processing clearer and avoids potential misuse of a variable declared multiple times.
3. Descriptive Naming Conventions
Incorporate clear, descriptive methods for complex logic conditions. The goal is to make your code self-explanatory.
For example, instead of:
if (user.isActive() && user.hasSubscription()) {
// Logic
}
Utilize:
if (isUserEligibleForService(user)) {
// Logic
}
private boolean isUserEligibleForService(User user) {
return user.isActive() && user.hasSubscription();
}
The condition's meaning becomes instantly clearer, resulting in easier code review and collaboration.
4. Leverage Functional Programming Concepts
Java has embraced functional programming concepts with the introduction of streams and lambda expressions. Conditions can now be expressed in a more functional manner:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
numbers.stream()
.filter(n -> n > 3) // Only keep numbers greater than 3
.forEach(System.out::println);
This makes your intention clear, significantly enhancing readability and conciseness.
5. Maintain Consistency
Consistency in how conditions are structured is crucial. Align with conventions across your codebase to reduce confusion. Adhering to established patterns reduces friction when other developers review or contribute to the code.
The Bottom Line
Conditional logic is an essential aspect of programming in Java. By following best practices and avoiding common pitfalls, we can ensure our code remains readable and maintainable. Techniques such as early returns, immutability, descriptive naming, and functional approaches elevate your code quality.
While Java and JavaScript share similarities in handling conditions, each language has its peculiarities. For further elaboration on mistakes you can encounter in JavaScript, check the article “Common Mistakes When Adding Conditions in JavaScript” at tech-snags.com/articles/common-mistakes-adding-conditions-javascript.
By optimizing your use of conditional logic, you're not just writing code—you're crafting a reliable foundation for solid, long-term software development. Happy coding!
Checkout our other articles