Avoiding Common Pitfalls When Using Java with Conditions
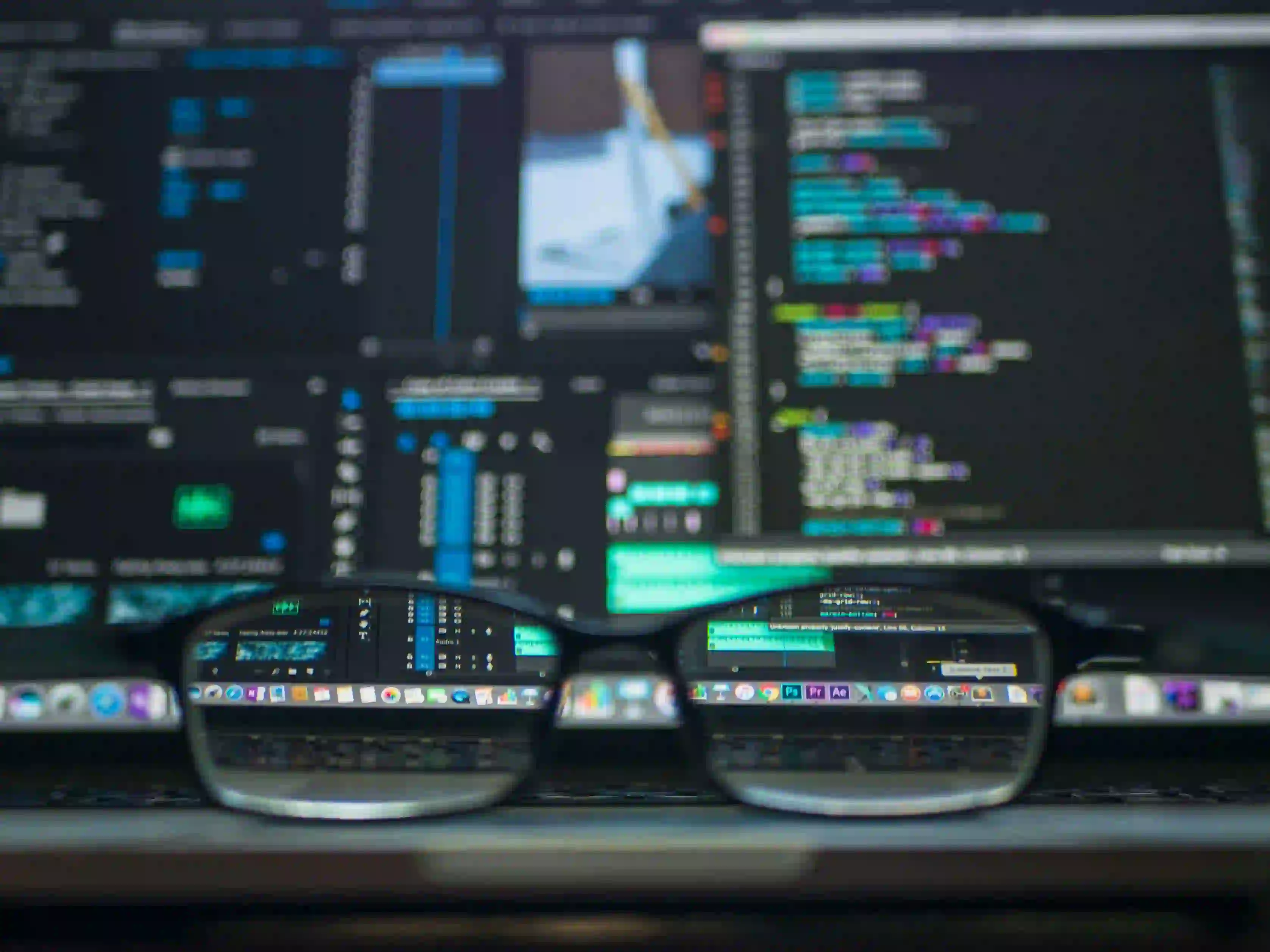
Avoiding Common Pitfalls When Using Java with Conditions
Java is a powerful programming language known for its versatility, but like any language, it comes with its own set of challenges. One area where many developers stumble is when using conditions in their Java code. This article will explore common pitfalls related to conditional statements and offer solutions to enhance your coding practices.
Understanding Conditional Statements
Conditional statements allow your program to make decisions. In Java, the if
, else if
, and else
statements, as well as switch
statements, are crucial for executing different actions based on varying conditions.
Here’s a simple breakdown of how an if
statement works:
int score = 85;
if (score >= 90) {
System.out.println("Grade: A");
} else if (score >= 80) {
System.out.println("Grade: B");
} else {
System.out.println("Grade: C");
}
Commentary
- Simple Structure: The code is straightforward and checks the value of
score
to determine the grade. - Readability: The conditions are clear and easy to understand, reflecting the logic needed for grading.
However, it's important to recognize that developing logic through conditions isn’t always smooth sailing. Let's unpack some common pitfalls.
Common Pitfalls in Java Conditional Statements
1. Neglecting to Handle Edge Cases
In programming, edge cases refer to the extreme circumstances that might not be encountered during typical operation but can still lead to errors. Ignoring these cases can cause unexpected behavior.
Example
int score = 110; // Invalid score
if (score < 0 || score > 100) {
System.out.println("Score is not valid.");
} else if (score >= 90) {
System.out.println("Grade: A");
}
Commentary
Here's why this check matters:
- Input Validation: We must validate inputs thoroughly to ensure our program behaves correctly.
- User Experience: Giving feedback for invalid inputs enhances user experience.
2. Overly Complex Conditions
Sometimes, developers combine multiple conditions in a single statement, leading to confusion.
Example
if (age > 18 && hasLicense == true || isEmployed) {
System.out.println("Eligible for loan.");
}
Commentary
Break complex conditions down:
- Readability: Aim for clarity. This condition could be confusing at a glance. It requires multiple mental operations to understand.
- Debugging: If there is an error in this logic, debugging becomes laborious.
Instead, consider refactoring as such:
boolean isEligibleForLoan = (age > 18 && hasLicense) || isEmployed;
if (isEligibleForLoan) {
System.out.println("Eligible for loan.");
}
This separation enhances clarity.
3. Relying on Floating-Point Comparisons
Java treats floating-point numbers in a way that can introduce inaccuracies. Avoid direct comparisons on floats and doubles.
Example
double a = 0.1 + 0.2;
double b = 0.3;
if (a == b) {
System.out.println("Equal!");
}
Commentary
Floating-point precision may lead to unexpected results:
- Precision Issues: It's common that
a
will not equalb
. Instead of direct equality, consider using a small threshold for comparison.
Refactor your code as follows:
final double EPSILON = 1e-10;
if (Math.abs(a - b) < EPSILON) {
System.out.println("Equal!");
}
4. Misusing the Ternary Operator
The ternary operator offers a compact way to write simple conditional statements, but can lead to unreadable code if misused.
Example
String status = (age >= 18) ? "Adult" : "Minor";
Commentary
While this code is acceptable, ensure its complexity does not increase. If nested, the readability may diminish:
String status = (age >= 18) ? (isStudent ? "Adult Student" : "Adult") : "Minor";
Refactor it for clarity:
String status;
if (age >= 18) {
status = isStudent ? "Adult Student" : "Adult";
} else {
status = "Minor";
}
5. Lack of Comments
As with any programming language, neglecting to comment your logic can result in confusion, especially as the codebase grows.
Java Commenting Practices
// This condition checks if the user is eligible based on age and license status
if (age > 18 && hasLicense) {
System.out.println("You are eligible to drive.");
}
Commentary
Comments provide insights into the logic:
- Context: They help someone new to the code (including future you) understand the intention behind the conditions.
- Maintenance: Well-commented code is easier to maintain.
Best Practices for Handling Conditions in Java
To avoid the aforementioned pitfalls when dealing with conditions in Java, consider the following best practices:
- Validate Inputs: Always check for valid input ranges, especially in user-driven applications.
- Keep Conditions Simple: Break complex conditions into simpler, clearer blocks of code.
- Avoid Floating-Point Comparisons: Always use thresholds when dealing with floats and doubles.
- Use Readable Constructs: Opt for clarity over compactness when using operators like the ternary.
- Comment Your Code: Regularly use comments to clarify more complex logic and share your thought process.
Maintaining a high standard helps prevent issues that could arise from mismanaged conditions.
Key Takeaways
Java is a robust language providing essential tools for conditional logic. However, it can also lead to common pitfalls that developers need to navigate. By effectively applying best practices through input validation, simplifying conditional expressions, and thoroughly commenting, you can create more legible, maintainable, and bug-free code.
For additional reading on similar issues in different contexts, consider checking out other articles focused on conditions, such as Common Mistakes When Adding Conditions in JavaScript.
As you continue your journey in Java, keep these tips in mind to avoid common mistakes and elevate your coding skills. Happy coding!