Avoiding Java Condition Errors: Common Pitfalls to Watch
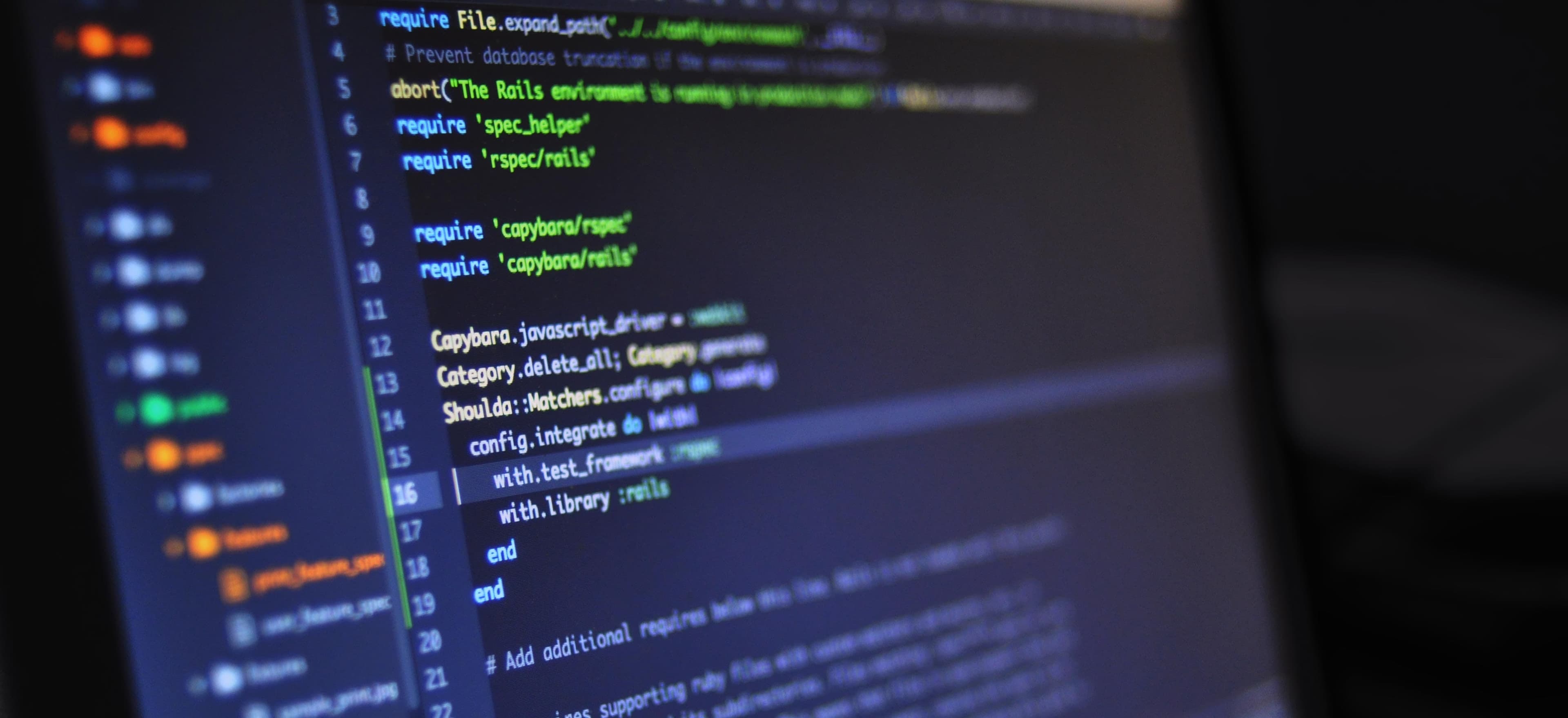
- Published on
Avoiding Java Condition Errors: Common Pitfalls to Watch
When programming in Java, one of the most critical aspects to keep in mind is how we manage conditions and control flow. While the language offers immense flexibility, it also presents several common pitfalls that can lead to bugs, inefficient code, or unintended behavior. In this blog post, we will explore these pitfalls, share best practices, and provide code snippets to illustrate the concepts. This will not only improve your Java programming skills but also help you avoid the condition errors that often plague developers.
Understanding Conditions in Java
Java uses conditions within various control flow statements such as if
, else
, switch
, and loops (for
, while
). A condition typically evaluates to either true
or false
. Understanding how to properly write and implement these conditions is vital.
Example of a Simple Condition
public class ConditionExample {
public static void main(String[] args) {
int number = 10;
if (number > 0) {
System.out.println("The number is positive.");
} else {
System.out.println("The number is not positive.");
}
}
}
In this example, the condition number > 0
checks if the variable number
holds a positive integer. If it does, we print a corresponding message.
Common Mistakes When Adding Conditions
In the programming realm, mistakes can happen anytime. For a more comprehensive understanding, you might find insightful information in the article Common Mistakes When Adding Conditions in JavaScript. Although this article touches on JavaScript specifically, it indirectly informs us about similar pitfalls in Java.
1. Using the Wrong Operator
One of the common mistakes is using assignment =
instead of comparison ==
. This can lead to misleading results.
Incorrect Code Example:
int a = 5;
if (a = 10) { // Mistake: using = instead of ==
System.out.println("a is 10");
}
Correct Code Example:
int a = 5;
if (a == 10) { // Correct: using ==
System.out.println("a is 10");
}
Why It Matters: Using =
assigns the value 10 to a
and the if condition always evaluates to true. This can lead to infinite loops or unintended behavior.
2. Neglecting to Use Curly Braces
Java allows for single-statement blocks without curly braces, but this can lead to confusion and bugs when multiple statements are intended.
Incorrect Code Example:
if (a > 0)
System.out.println("a is positive");
System.out.println("This line runs regardless.");
Correct Code Example:
if (a > 0) {
System.out.println("a is positive");
System.out.println("This line runs only if a is positive.");
}
Why It Matters: Not using curly braces can lead to logical errors when adding more statements later, as the programmer may assume only the first statement is controlled by the condition.
3. Improper Use of Logical Operators
Combining conditions with logical operators (AND &&
, OR ||
) can yield unexpected results if misunderstood.
Example with Logical Operators:
public class LogicalOperators {
public static void main(String[] args) {
int age = 18;
boolean hasID = true;
if (age >= 18 && hasID) {
System.out.println("Access granted.");
} else {
System.out.println("Access denied.");
}
}
}
Why It Matters: Ensure that the conditions you are combining with logical operators logically make sense. Misplacing parentheses or misunderstanding operator precedence can cause the entire condition to evaluate incorrectly.
4. Forgetting the Type Promotions
In Java, when using mixed types in conditions, type promotion can lead to unexpected behavior.
Incorrect Code Example:
double d = 5.0;
int number = 5;
if (number == d) { // Implicitly promoted!
System.out.println("Number and double are equal.");
}
Why It Matters: While Java handles this by promoting the int
to double
, relying on it can lead to precision issues in more complex calculations.
5. Short-Circuit Evaluation
Java evaluates conditions from left to right and uses short-circuit evaluation in logical expressions. This can lead to unexpected results or performance impacts if not understood properly.
Example with Short-Circuit Evaluation:
public class ShortCircuit {
public static void main(String[] args) {
int a = 10;
if (a < 5 && (++a > 10)) {
System.out.println("This won't execute.");
}
System.out.println(a); // Outputs: 10
}
}
Why It Matters: The ++a
is never evaluated because a < 5
is false. Knowing this can help in optimizing the performance of your conditions.
The Last Word
Coding in Java opens the door to incredible functionality and flexibility. However, overlooking simple condition errors can quickly lead to substantial issues down the line. By being aware of common pitfalls, such as misusing operators, neglecting curly braces, and misunderstanding type promotion, you can avoid many headaches.
To reinforce your understanding, take a moment to review your existing code and see where these best practices can be implemented. The strategies shared here should empower you to write cleaner, more efficient Java code, and minimize those pesky condition errors.
For additional insights into conditions in programming, check out Common Mistakes When Adding Conditions in JavaScript. Happy coding, and remember: a little attention to detail goes a long way!
Checkout our other articles