Avoiding Deployment Pitfalls: Istio on EKS with Java APIs
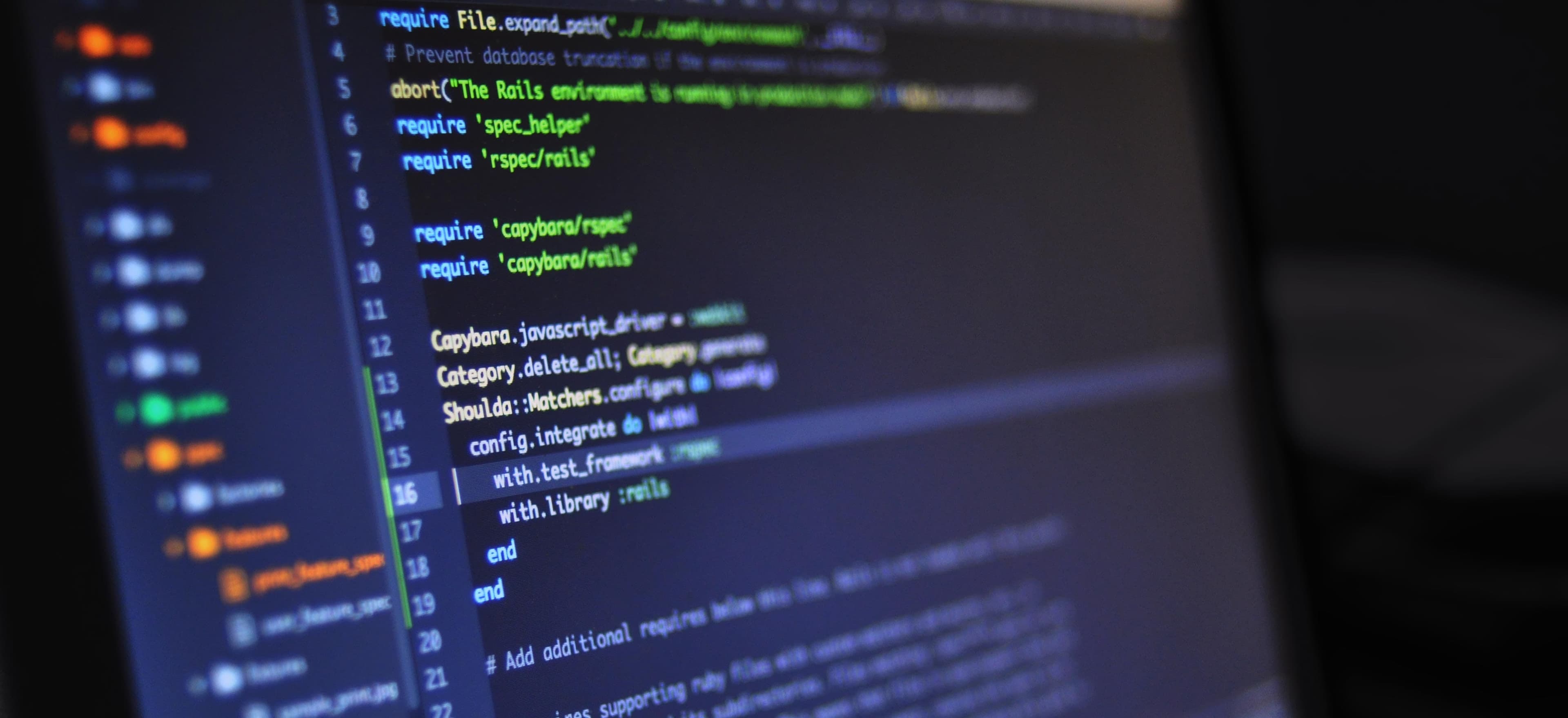
- Published on
Avoiding Deployment Pitfalls: Istio on EKS with Java APIs
When it comes to deploying microservices, the combination of Kubernetes and Istio is a trend that cannot be overlooked. This powerful duo allows developers to create more robust applications with features like traffic management, security, and observability out of the box. However, deploying Istio on Amazon EKS (Elastic Kubernetes Service) comes with its own set of challenges that can trip up even seasoned developers. In this blog post, we’ll examine potential pitfalls and focus on how to effectively utilize Java APIs while integrating with Istio on EKS.
The What and Why of Istio
Before we dive into the potential pitfalls, let's briefly touch on what Istio is and why it matters. Istio is an open-source service mesh that provides a way to control how microservices share data with one another. It acts as a layer of infrastructure, separating the business logic in your applications from the necessary networking logic.
Key Features of Istio:
- Traffic Management: Control the flow of traffic and API calls between services.
- Security: Manage authentication, authorization, and encryption for your services.
- Observability: Monitor and trace your microservices for better diagnostics.
This leads us to our focus: deploying Istio on EKS with Java APIs. Let’s explore some common pitfalls.
The Common Pitfalls
1. Inadequate Resource Allocation
One of the most frequent mistakes developers make when deploying Istio on EKS is inadequate resource allocation. Proper resource limits and requests for CPU and memory should be set in your Kubernetes YAML files.
Example YAML Configuration:
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-java-service
spec:
replicas: 3
selector:
matchLabels:
app: my-java-service
template:
metadata:
labels:
app: my-java-service
spec:
containers:
- name: my-java-service
image: my-repo/my-java-image:latest
resources:
requests:
memory: "512Mi"
cpu: "500m"
limits:
memory: "1Gi"
cpu: "1"
Why this Matters:
Allocating resources appropriately prevents application crashes and performance degradation. EKS auto-scaling works optimally only when resource requests are clearly defined.
2. Misconfigured Sidecar
Istio uses a "sidecar" proxy that intercepts traffic to and from your services. Misconfiguration of this sidecar can lead to routing issues.
Example Sidecar Configuration:
apiVersion: v1
kind: Service
metadata:
name: my-java-service
spec:
ports:
- port: 80
targetPort: 8080
selector:
app: my-java-service
---
apiVersion: networking.istio.io/v1alpha3
kind: VirtualService
metadata:
name: my-java-service
spec:
hosts:
- my-java-service
http:
- route:
- destination:
host: my-java-service
port:
number: 80
Why this Matters:
Without a properly configured sidecar, traffic may not reach your services correctly, leading to timeouts or failures. Please refer to this resource for more information: Common Pitfalls When Deploying Istio on EKS.
3. Forgetting the Ingress Gateway
Utilizing Istio’s Ingress Gateway allows external traffic to enter your services. Many developers overlook this crucial component.
Example Ingress Configuration:
apiVersion: networking.istio.io/v1alpha3
kind: Gateway
metadata:
name: my-java-service-gateway
spec:
selector:
istio: ingressgateway
servers:
- port:
number: 80
name: http
protocol: HTTP
hosts:
- "*"
Why this Matters:
The Ingress Gateway manages access. Ignoring it may lead to services being exposed without control, creating security vulnerabilities.
Leveraging Java APIs with Istio
Now that we’ve identified some pitfalls, let’s explore how Java APIs can effectively coexist with Istio on EKS.
1. Creating REST Services
Java can be used to build RESTful services that will communicate through Istio. You can use frameworks like Spring Boot to set this up seamlessly.
Example of a Simple REST Endpoint:
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class HelloController {
@GetMapping("/hello")
public String hello() {
return "Hello from the Java API!";
}
}
How it Works:
This endpoint, when deployed and routed through Istio, will seamlessly adapt according to traffic management policies defined in your VirtualService.
2. Securing Endpoints
Implementing security measures to protect your Java APIs can be done using JWT (JSON Web Tokens) and Istio's authentication policies.
Example of Adding Authentication:
apiVersion: security.istio.io/v1beta1
kind: AuthorizationPolicy
metadata:
name: my-java-service-policy
spec:
rules:
- from:
- source:
requestPrincipals: ["*"]
to:
- operation:
methods: ["GET"]
paths: ["/hello"]
Significance:
Setting up authorization policies within Istio ensures that only authenticated requests can access your Java APIs.
Debugging and Monitoring
Debugging and monitoring are equally important when dealing with Istio. Utilizing tools like Kiali or Jaeger can provide visibility into your services and their interactions.
Example of Setting Up Jaeger:
apiVersion: install.istio.io/v1alpha1
kind: IstioOperator
metadata:
namespace: istio-system
spec:
components:
tracing:
enabled: true
provider: jaeger
Why Monitoring is Crucial:
Monitoring allows you to quickly identify performance issues, making it far easier to address potential pitfalls before they escalate.
The Bottom Line
Deploying Istio on AWS EKS while utilizing Java APIs can be a robust solution for managing microservices. However, it’s essential to keep in mind the common pitfalls that can derail your efforts. Ensure proper resource allocation, sidecar configuration, and implement security measures effectively.
For further reading, consider checking out the article on Common Pitfalls When Deploying Istio on EKS.
Employing best practices and utilizing Java frameworks properly will ultimately lead to a more resilient microservices architecture, streamlining your deployment processes and maintaining service availability and security. Happy deploying!
Checkout our other articles