Avoiding Java-Based Pitfalls When Deploying Istio on EKS
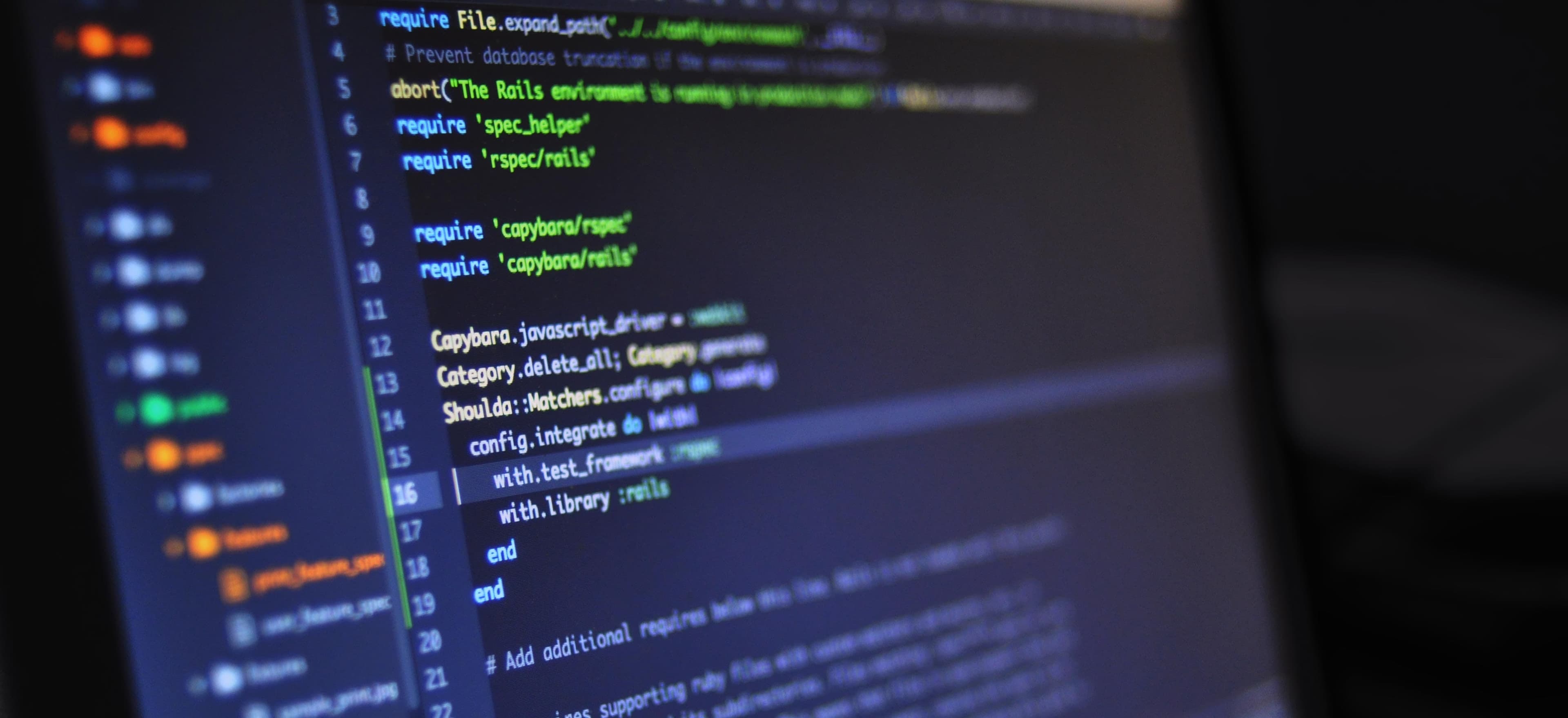
- Published on
Avoiding Java-Based Pitfalls When Deploying Istio on EKS
Deploying Istio on Amazon Elastic Kubernetes Service (EKS) can significantly enhance your application's resilience and security features. However, there are several pitfalls, particularly when integrating Java applications with Istio. This article aims to provide insights, solutions, and best practices for avoiding those pitfalls, ensuring your deployment is smooth and efficient.
Understanding Istio and EKS
First, let’s briefly discuss what Istio and EKS are.
- Istio is an open-source service mesh that provides a way to control how microservices share data with one another. It offers features such as traffic management, security, and observability.
- EKS (Elastic Kubernetes Service) is a managed service that makes it easy to run Kubernetes on AWS without needing to install and operate your own Kubernetes control plane.
When combined, these tools can create a robust architecture that is both powerful and flexible. However, deploying a Java application using this architecture can present unique challenges.
Common Pitfalls When Deploying Istio on EKS
When deploying Java-based applications with Istio on EKS, developers can run into several issues. These range from performance bottlenecks to configuration errors. Many of these pitfalls stem from the inherent differences between how Java applications function and the requirements of Istio.
1. Understanding JVM Overhead
The Java Virtual Machine (JVM) introduces overhead that can impact the performance of your microservices.
import java.util.concurrent.*;
public class PerformanceTest {
public static void main(String[] args) {
int numThreads = Runtime.getRuntime().availableProcessors();
ExecutorService executor = Executors.newFixedThreadPool(numThreads);
for (int i = 0; i < numThreads; i++) {
executor.submit(() -> {
// Simulating computation
for (int j = 0; j < 1000000; j++) {
Math.sin(Math.random());
}
});
}
executor.shutdown();
try {
executor.awaitTermination(1, TimeUnit.MINUTES);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("All tasks completed!");
}
}
Why This Matters
Understanding JVM behavior is critical. The overhead generated by threading and garbage collection can affect latency when Istio is managing traffic. Use a profiler to identify bottlenecks caused by the JVM and tune your application accordingly.
2. Misconfigured Gateway and Virtual Services
One common mistake is the misconfiguration of Istio gateways and virtual services. Mistakes in the YAML configuration can lead to 404 errors or service failures.
Here’s an example of how to set up a simple virtual service.
apiVersion: networking.istio.io/v1alpha3
kind: VirtualService
metadata:
name: my-service
spec:
hosts:
- my-service.default.svc.cluster.local
http:
- match:
- uri:
prefix: /api
route:
- destination:
host: my-service
port:
number: 8080
Why This Matters
Make sure that the service name, port number, and matching criteria align with your actual service endpoints. This small mistake can often lead to significant deployment blockers.
3. Ignoring Health Checks
Health checks are essential for managing service availability. Without properly configured liveness and readiness probes, Istio may route traffic to a service that is not ready to handle it.
apiVersion: v1
kind: Pod
metadata:
name: my-java-app
spec:
containers:
- name: java-container
image: my-java-app:latest
readinessProbe:
httpGet:
path: /actuator/health
port: 8080
initialDelaySeconds: 5
periodSeconds: 10
Why This Matters
By correctly setting these probes, you ensure that Istio only routes traffic to instances of your service that can handle requests, thereby minimizing errors and improving performance.
4. Lack of Observability
Another significant pitfall is neglecting observability. Istio offers a rich set of telemetry features, but failing to integrate them into your Java application can lead to blind spots in your monitoring and debugging efforts.
Use libraries like Micrometer or Spring Cloud Sleuth to automatically report metrics and tracing information.
Code Snippet for Micrometer Integration
import io.micrometer.core.instrument.MeterRegistry;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class MyService {
private final MeterRegistry meterRegistry;
@Autowired
public MyService(MeterRegistry meterRegistry) {
this.meterRegistry = meterRegistry;
}
public void performOperation() {
// Logic for operation
meterRegistry.counter("my_service.operation.count").increment();
}
}
Why This Matters
Implementing observability enables you to gain insights into your application's behavior, performance, and health. Without it, you're left troubleshooting problems in the dark.
5. Overlooking Traffic Policies
Istio allows for advanced traffic management features like traffic splitting, circuit breaking, and more. However, not utilizing these features can lead to inefficient resource usage or unexpected downtime during deployments.
apiVersion: networking.istio.io/v1alpha3
kind: DestinationRule
metadata:
name: my-service
spec:
host: my-service
trafficPolicy:
connectionPool:
tcp:
maxConnections: 100
http:
http1MaxPendingRequests: 200
http2MaxRequests: 200
Why This Matters
By defining traffic policies, you can ensure that your Java service handles varying loads gracefully, protecting your application from sudden spikes in traffic.
Best Practices for Deploying Java Applications in Istio on EKS
- Monitor JVM Performance: Use profiling tools to keep an eye on your Java performance.
- Validate Configurations: Always double-check your YAML files for any inadvertent errors or misconfigurations.
- Use Observability Tools: Integrate services like Prometheus and Grafana.
- Test Thoroughly: Use automated tests to simulate various traffic patterns before going live.
- Read Best Practices: Before deploying, consult articles like “Common Pitfalls When Deploying Istio on EKS” for detailed insights on avoiding common mistakes (https://configzen.com/blog/pitfalls-istio-deploy-eks).
Final Thoughts
Deploying Java applications on EKS using Istio can enhance your application's capabilities significantly. However, it is essential to navigate the various pitfalls specific to Java applications. By following the strategies discussed in this article, you can pave the way for a successful deployment.
For more insights on deploying Istio on EKS, be sure to check out additional resources and articles featured throughout the community. Happy coding!
Checkout our other articles