Integrating Java for Enhanced E-commerce Spice Marketing
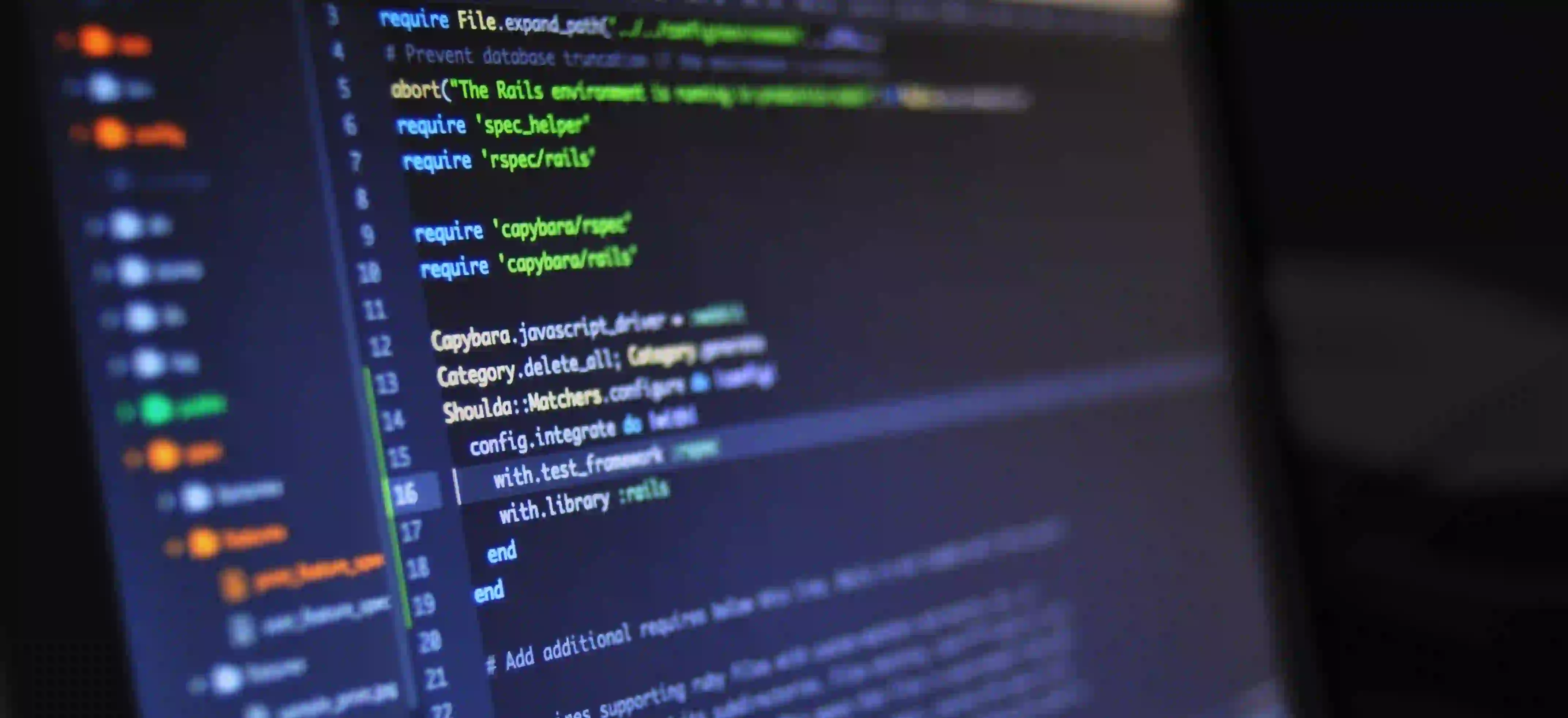
Integrating Java for Enhanced E-commerce Spice Marketing
As the e-commerce landscape continues to expand, businesses are seeking innovative ways to stand out in a crowded market. One industry that has gained particular attention is the spice market, where products like Gewürznelken (cloves) play a significant role. By leveraging the power of Java, businesses can enhance their online presence and streamline their operations in the spice trade. In this blog post, we'll explore how Java can be utilized for e-commerce applications, along with some practical coding examples.
Why Choose Java for E-commerce?
Java is a versatile programming language that has been a staple in the software development industry for decades. Here are a few reasons why Java is an excellent choice for e-commerce applications:
-
Platform Independence: Java applications can run on any device with a Java Virtual Machine (JVM). This feature is particularly useful for an international e-commerce business that requires accessibility across diverse environments.
-
Robustness and Security: Java is known for its reliability. Its strong memory management and exception handling contribute to the stability of e-commerce applications, essential for handling transactions securely.
-
Scalability: As your business grows, Java applications can scale effortlessly, accommodating higher traffic and larger data volumes without compromising performance.
-
Rich Ecosystem: With a wide range of frameworks and libraries, Java offers additional functionalities that can enhance e-commerce platforms.
All these features make Java an appealing option for businesses looking to integrate comprehensive e-commerce solutions.
Understanding the E-commerce Architecture
Before diving into programming, it’s crucial to understand the architecture of an e-commerce application. Generally, a typical e-commerce application is structured as follows:
-
Presentation Layer: This is the user interface where customers browse and select products, including spices like cloves. It involves web pages, mobile apps, and any other points of customer interaction.
-
Application Layer: This layer contains business logic, including product management, inventory control, and order processing.
-
Database Layer: This viable backend stores all the records of products, transactions, and users.
Coding for E-commerce: Basic Snippet
Let’s start with a straightforward Java code snippet that illustrates how to manage products in an e-commerce application.
Creating a Product Class
public class Product {
private String name;
private double price;
private String category;
public Product(String name, double price, String category) {
this.name = name;
this.price = price;
this.category = category;
}
public String getName() {
return name;
}
public double getPrice() {
return price;
}
public String getCategory() {
return category;
}
@Override
public String toString() {
return "Product{name='" + name + '\'' + ", price=" + price + ", category='" + category + '\'' + '}';
}
}
Explanation
- Product Class: This class represents a product in the inventory. It contains attributes for
name
,price
, andcategory
. - Constructor: Initializes the properties of the product when a new instance is created.
- Getters: Provides access to the product details from other classes.
This class acts as the backbone for managing spices, making it easier to process and display items like cloves.
Managing Products with Collections
Once you have defined your product, you need a way to manage a collection of them. Using collections like ArrayList will allow you to dynamically handle numerous products.
Managing Product List Example
import java.util.ArrayList;
import java.util.List;
public class ProductManager {
private List<Product> products;
public ProductManager() {
products = new ArrayList<>();
}
public void addProduct(Product product) {
products.add(product);
}
public List<Product> getProducts() {
return products;
}
public void displayProducts() {
for (Product product : products) {
System.out.println(product);
}
}
}
Explanation
- ProductManager Class: This class manages a list of products.
- ArrayList: We utilize the ArrayList for dynamic sizing. As you add products, the list grows automatically.
- Display Method: A convenient way to print out all the products in the inventory, which can be useful for debugging or interface purposes.
Integrating Payment Systems
For any e-commerce application, a secure payment gateway is essential. Let's look at a basic implementation of a payment processing class.
Payment Processing Example
public class PaymentProcessor {
public boolean processPayment(String cardNumber, double amount) {
// Simulating payment processing logic
if (isValidCard(cardNumber) && amount > 0) {
System.out.println("Payment of " + amount + " processed successfully.");
return true;
}
System.out.println("Payment failed.");
return false;
}
private boolean isValidCard(String cardNumber) {
// Simple validation logic for demo purposes
return cardNumber.length() == 16;
}
}
Explanation
- PaymentProcessor Class: Simulates a payment gateway.
- Payment Method: Accepts a card number and amount to process payments but includes validation logic to ensure the card number is the correct length.
While this is a basic illustration, integrating a real payment gateway can enhance security and user experience.
Optimizing Your Application for SEO
A well-optimized e-commerce website can significantly enhance visibility in search engine results. Here are some Java-focused tips:
- Readable URLs: Structure URLs to include keywords (e.g., www.yourstore.com/spices/cloves).
- Dynamic Meta Tags: Use Java servlets to dynamically generate meta tags based on user search queries.
- Mobile Optimization: Ensure your Java applications are responsive, catering to users on various devices.
For example, if you're selling Gewürznelken, your application should dynamically adjust the content based on the user's interaction, which can lead to higher engagement and better rankings.
For more in-depth insights on spice marketing, refer to the article titled "Gewürznelken: Kleine Kostbarkeiten mit großer Wirkung" at auraglyphs.com/post/gewuerznelken-kleine-kostbarkeiten-wirkung.
The Bottom Line
Integrating Java into your e-commerce strategy can significantly enhance your capability to manage products and process transactions. Leveraging the reliability, scalability, and security of Java allows businesses, especially in niche markets like spices, to create robust applications that meet customer needs. With Java's rich ecosystem, you're well-equipped to tackle the challenges of the e-commerce landscape.
As the market continues to evolve, embracing modern programming practices will ensure your business remains competitive. Whether you're developing the front-end interface or managing the backend logic, Java offers the tools that can streamline your processes and enhance the overall shopping experience.