Mitigating Namespace Conflicts in Java Frameworks
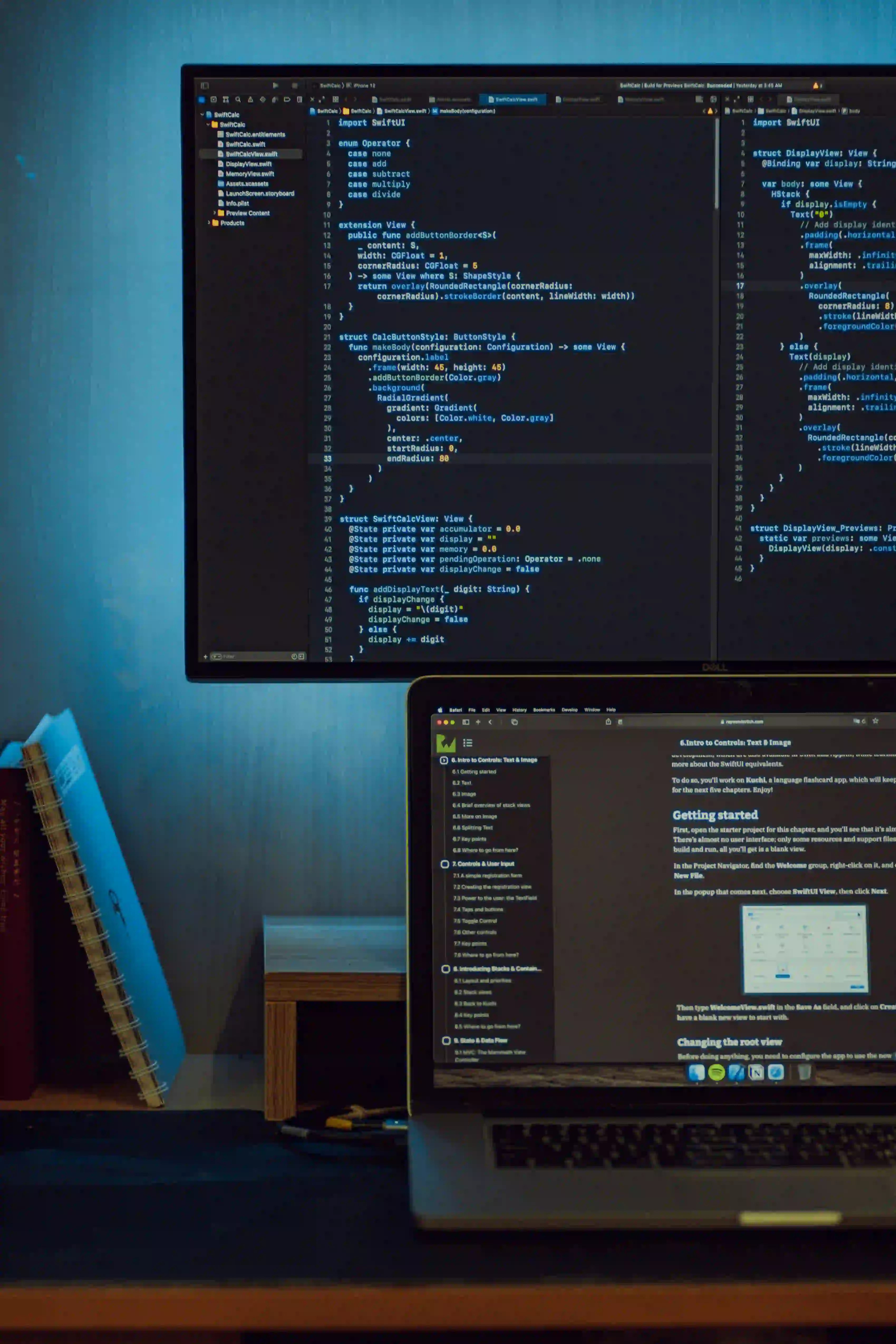
Mitigating Namespace Conflicts in Java Frameworks
Working with Java frameworks can be a powerful experience. However, developers often face the challenge of namespace conflicts, particularly when scaling applications or integrating third-party libraries. In this blog post, we’ll explore best practices to mitigate namespace conflicts in Java, ensuring cleaner and more maintainable code. By the end, you'll have a solid toolkit for avoiding and resolving namespace conflicts in your Java applications.
What are Namespace Conflicts?
In programming, a namespace is a container that holds a set of identifiers such as variable names, function names, and class names. In Java, namespaces are primarily organized through packages. A namespace conflict occurs when two identifiers share the same name within the same scope, leading to ambiguity in code execution.
Why are Namespace Conflicts Problematic?
Namespace conflicts can lead to:
- Compilation Errors: The Java compiler may fail to compile the code due to ambiguity.
- Runtime Errors: If a conflict is not caught at compile time, it can lead to bugs that are hard to debug.
- Maintenance Challenges: Code readability and maintainability suffer, as it becomes difficult to determine which identifier is being referenced.
Best Practices to Mitigate Namespace Conflicts
1. Use Unique Package Names
A good starting point is adopting a unique package naming convention. The Java package naming convention recommends using the reverse of your organization’s domain name. For instance, if your domain is mycompany.com
, your packages might start with com.mycompany
.
Example:
package com.mycompany.appname.module;
This reduces the risk of conflicts with open-source libraries or other teams.
2. Modularize Your Code
By splitting applications into modules or libraries, each with its own package structure, you can significantly reduce potential conflicts. For instance, consider a large e-commerce application split into various microservices. Each service can maintain its own namespace, lowering conflict chances.
3. Leverage Classpath Location
When importing external classes, ensure you are importing from the correct package or class path. Use fully qualified names when necessary.
Pointed Example:
import com.google.common.collect.Lists; // Avoids conflicts with local Lists class
In this case, using the fully qualified name clarifies which Lists
class is being used.
4. Utilize Aliases with Imports
When you are forced to import classes with the same name, aliasing can help maintain clarity.
Example:
import java.util.List; // java.util.List
import org.example.model.List as ModelList; // Alias for custom List
public class Example {
private List<String> stringList; // java.util.List
private ModelList modelList; // Custom model List
}
5. Avoid Wildcard Imports
Using wildcard imports (e.g., import java.util.*;
) can clutter your namespace and potentially lead to conflicts. Be explicit about your imports and only include what you need.
import java.util.List; // Preferred
import java.util.ArrayList; // Preferred
6. Regular Refactoring
Code refactoring is crucial. As projects grow, frequent refactoring helps manage namespace and organize code more intuitively. Aim to remove unused imports, extract common functionalities, and rename packages or classes that could be prone to conflicts.
7. Document Your Code - Javadoc
Documentation plays a significant role in understanding the purpose and function of classes and methods, which can help avoid misinterpretation. Writing comprehensive Javadoc comments will ensure that the intent behind a class or method is clear.
Example:
/**
* Represents a service for managing user accounts.
*/
public class UserAccountService {
// Service methods go here
}
8. Consider Dependency Management Tools
Using tools like Maven or Gradle can help manage your project’s dependencies more effectively. These tools can automatically resolve version conflicts, minimizing potential namespace issues.
<dependency>
<groupId>org.example</groupId>
<artifactId>example-artifact</artifactId>
<version>1.0.0</version>
</dependency>
These practices will guide you cleanly through the dependencies you manage, reducing risk.
Additional Considerations
In addition to the practices mentioned, understanding how namespaces work in Java can give you insight into preventing conflicts. Java uses hierarchical namespaces, which means a more specific package can exist alongside a more general package without conflict.
However, keep in mind the importance of readability and maintainability over merely resolving conflicts. When changing namespaces, consider the broader impact on the codebase, ensuring that your team can effortlessly collaborate.
Final Considerations
Namespace conflicts in Java frameworks can be daunting, but by following these best practices, you can greatly mitigate their occurrence. Unique package naming, modularization, leveraging classpath locations, and avoiding wildcard imports are fundamental strategies every developer should adopt.
For those who are also working with other programming languages, such as PHP, learning how to resolve namespace conflicts can further your understanding of this concept. An excellent resource on this matter is the article titled "Resolving Namespace Conflicts in PHPAuth: A Guide," which you can find at tech-snags.com/articles/resolving-namespace-conflicts-phpauth-guide.
By equipping yourself with these strategies, you're not just avoiding problems, but you're also paving the way for creating cleaner, more manageable Java applications. Keep coding and improving your craft!