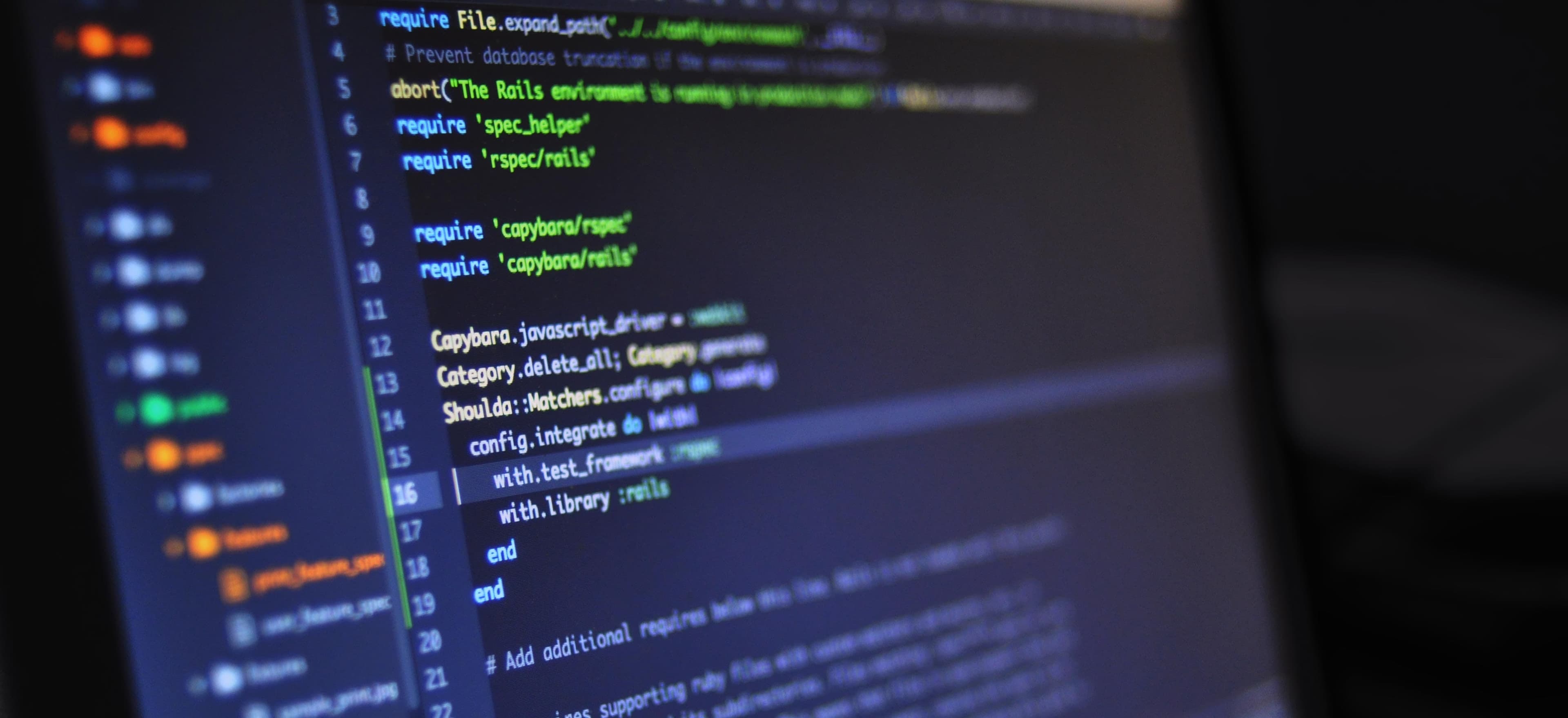
- Published on
Are you tired of oversleeping and being late for work or appointments? Do you want to create an alarm clock that suits your specific needs and schedule? Java can be the perfect tool to help solve this dilemma. In this blog post, we will create a simple yet effective alarm clock application using Java. We will cover the essential functionalities of an alarm clock such as setting alarms, displaying the current time, and handling alarm events. So, let's dive into the world of Java programming and create our own custom alarm clock!
Understanding the Requirements
Before we start coding, it's crucial to understand the requirements of our alarm clock application. We want to build an application that allows users to:
- Set multiple alarms.
- Display the current time.
- Have the option to snooze or dismiss alarms.
- Play a sound when an alarm is triggered.
- Have a user-friendly interface.
Now that we have a clear set of requirements, let's start building our Java alarm clock application.
Creating the Alarm Class
The first step is to create a class to represent an alarm. We'll define the attributes of an alarm, such as the time it is set for and whether it is currently active. We'll also include methods to set the alarm time, check if it is time for the alarm to go off, and toggle the active status of the alarm.
public class Alarm {
private LocalTime alarmTime;
private boolean isActive;
public void setAlarmTime(LocalTime time) {
this.alarmTime = time;
}
public boolean isTimeToRing(LocalTime currentTime) {
return isActive && currentTime.equals(alarmTime);
}
public void toggle() {
isActive = !isActive;
}
}
In the Alarm
class, we use the LocalTime
class from the java.time
package to handle time-related operations. Using LocalTime
provides better clarity and precision compared to using traditional Date
and Calendar
classes.
Building the Alarm Clock Application
Now that we have our Alarm
class, we can proceed to build the main AlarmClock
application. We'll use Java's Swing framework to create a simple graphical user interface (GUI) for our alarm clock.
import javax.swing.*;
import java.awt.*;
import java.time.LocalTime;
public class AlarmClock extends JFrame {
private JLabel timeLabel;
private JButton setAlarmButton;
private Alarm[] alarms;
public AlarmClock() {
// Initialize alarms array
alarms = new Alarm[5];
// Set up the GUI
setTitle("Java Alarm Clock");
setSize(300, 200);
setDefaultCloseOperation(EXIT_ON_CLOSE);
setLayout(new FlowLayout());
timeLabel = new JLabel();
setAlarmButton = new JButton("Set Alarm");
add(timeLabel);
add(setAlarmButton);
// Update the time label every second
Timer timer = new Timer(1000, e -> {
LocalTime currentTime = LocalTime.now();
timeLabel.setText(currentTime.toString());
// Check if any alarm should go off
for (Alarm alarm : alarms) {
if (alarm != null && alarm.isTimeToRing(currentTime)) {
// Trigger the alarm (play sound, show notification, etc.)
}
}
});
timer.start();
// Set up the set alarm button action
setAlarmButton.addActionListener(e -> {
// Logic to set a new alarm based on user input
});
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
new AlarmClock().setVisible(true);
});
}
}
In this AlarmClock
class, we utilize Swing components like JFrame
, JLabel
, and JButton
to construct the GUI. We also incorporate a Timer
to update the current time displayed every second and check if any alarms need to go off. The set alarm button's action is left as a placeholder for the logic to set a new alarm based on user input.
Implementing Alarm Actions
To complete our alarm clock application, we need to implement the actions to be taken when an alarm goes off. This can involve playing a sound, displaying a notification, or any other desired behavior. For simplicity, let's create a method to print a message to the console when an alarm is triggered.
public class Alarm {
// ... (previous code remains the same)
public void trigger() {
System.out.println("Alarm is going off!");
}
}
In the Alarm
class, we add a trigger
method to handle the action when an alarm is triggered.
To Wrap Things Up
In this blog post, we've explored how to create a simple alarm clock application using Java. We've covered the essential steps of defining the alarm class, building the alarm clock application with a user interface, and implementing alarm actions. You can further enhance this application by adding features like snooze, alarm sound, and a more sophisticated user interface.
Java's versatility and robustness make it a great choice for developing desktop applications like an alarm clock. Whether you're a beginner or an experienced Java developer, creating a custom alarm clock can be a fun and practical way to hone your programming skills.
Now that you have a solid understanding of the fundamentals, feel free to extend the functionality of our Java alarm clock or explore other Java GUI frameworks like JavaFX for more advanced desktop applications.
Start your Java journey today and wake up to a brighter, more punctual future with your custom-built alarm clock!