Efficient File Filtering in Java 7 Using NIO: Performance Boost
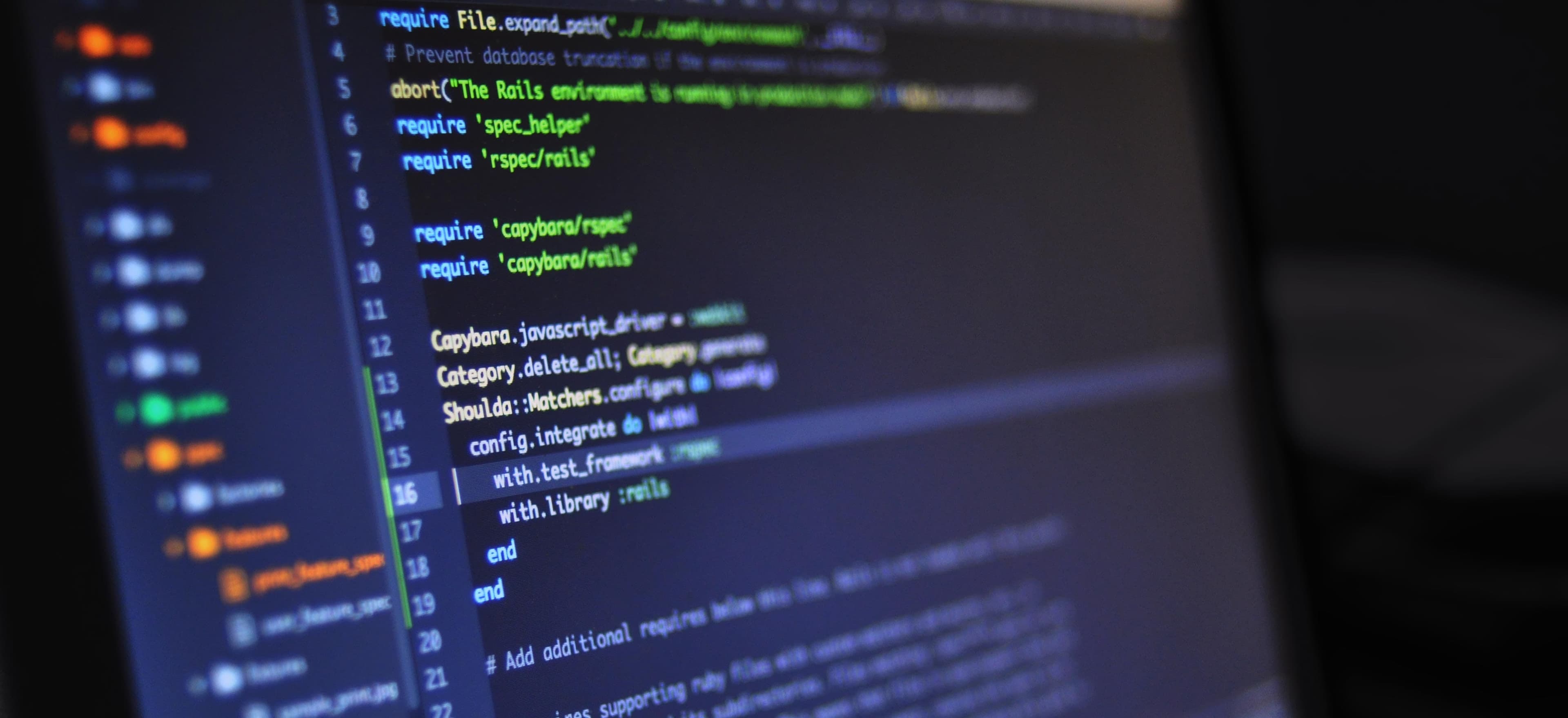
- Published on
Efficient File Filtering in Java 7 Using NIO: Performance Boost
When it comes to manipulating files in Java, efficiency and performance are always at the top of the priority list. With the introduction of the NIO (New Input/Output) package in Java 7, developers gained powerful tools for handling file operations with improved performance. In this article, we will explore how to leverage NIO for efficient file filtering in Java 7, and the performance benefits it brings to the table.
The Traditional Approach
Before diving into NIO, let's take a brief look at the traditional file filtering approach using File
and FileFilter
in Java.
File directory = new File("path/to/directory");
File[] matchingFiles = directory.listFiles(new FileFilter() {
@Override
public boolean accept(File pathname) {
return pathname.getName().endsWith(".txt");
}
});
While this approach gets the job done, it has limitations and performance drawbacks, especially when dealing with a large number of files. Each file operation involves interacting with the underlying file system, which can result in decreased performance.
Introducing NIO for File Filtering
Java NIO provides a more efficient way to perform file operations, including filtering. The Path
and Files
classes offer methods for filtering files based on various criteria.
Let's take a look at how we can use NIO to filter files based on their extension:
Path directory = Paths.get("path/to/directory");
try (DirectoryStream<Path> stream = Files.newDirectoryStream(directory, "*.txt")) {
for (Path file : stream) {
// Process the matching files
}
} catch (IOException e) {
// Handle file I/O exception
}
In this example, we use Files.newDirectoryStream()
to obtain a stream of paths that match the specified glob pattern, "*.txt". Using try-with-resources ensures that the stream is closed after use, avoiding resource leaks.
Performance Benefits of NIO File Filtering
The NIO approach offers several performance benefits compared to the traditional approach:
1. Reduced System Calls
NIO minimizes the number of system calls required to manipulate files, resulting in improved performance, especially when dealing with a large number of files.
2. Asynchronous File I/O
NIO introduces support for asynchronous file I/O operations, allowing for non-blocking I/O, which can lead to significant performance gains in certain scenarios.
3. Efficient Memory Mapping
NIO provides memory-mapped files, allowing direct access to the file content from the memory, eliminating the need for frequent disk reads.
4. Improved File Watching
With NIO, developers can efficiently monitor file system events, such as file creation, deletion, and modification, without the need for polling, leading to efficient handling of file changes.
Best Practices for NIO File Filtering
While NIO brings significant performance benefits, it's essential to follow best practices to maximize its potential:
1. Use Proper Error Handling
Always handle file I/O exceptions gracefully to prevent unexpected failures in production environments.
2. Leverage Try-with-Resources
Utilize try-with-resources to ensure that resources are properly closed after use, preventing resource leaks and potential performance degradation.
3. Optimize DirectoryStream Filters
Make use of more complex filters with DirectoryStream.Filter
to refine file selection criteria, enhancing the efficiency of file filtering operations.
4. Consider Asynchronous File Channels
For high-throughput file I/O, consider leveraging asynchronous file channels for non-blocking operations, especially in scenarios where performance is critical.
In Conclusion, Here is What Matters
In conclusion, leveraging NIO for file filtering in Java 7 can provide a significant performance boost compared to the traditional approach. By minimizing system calls, enabling asynchronous I/O, and offering efficient memory mapping, NIO empowers developers to handle file operations with enhanced performance and scalability.
As always, it's crucial to understand the nuances of NIO and follow best practices to harness its full potential while developing efficient file filtering solutions in Java.
Start implementing NIO for your file filtering tasks and experience the performance and efficiency gains firsthand in your Java projects!