The Challenge of Generating Efficient Source Code
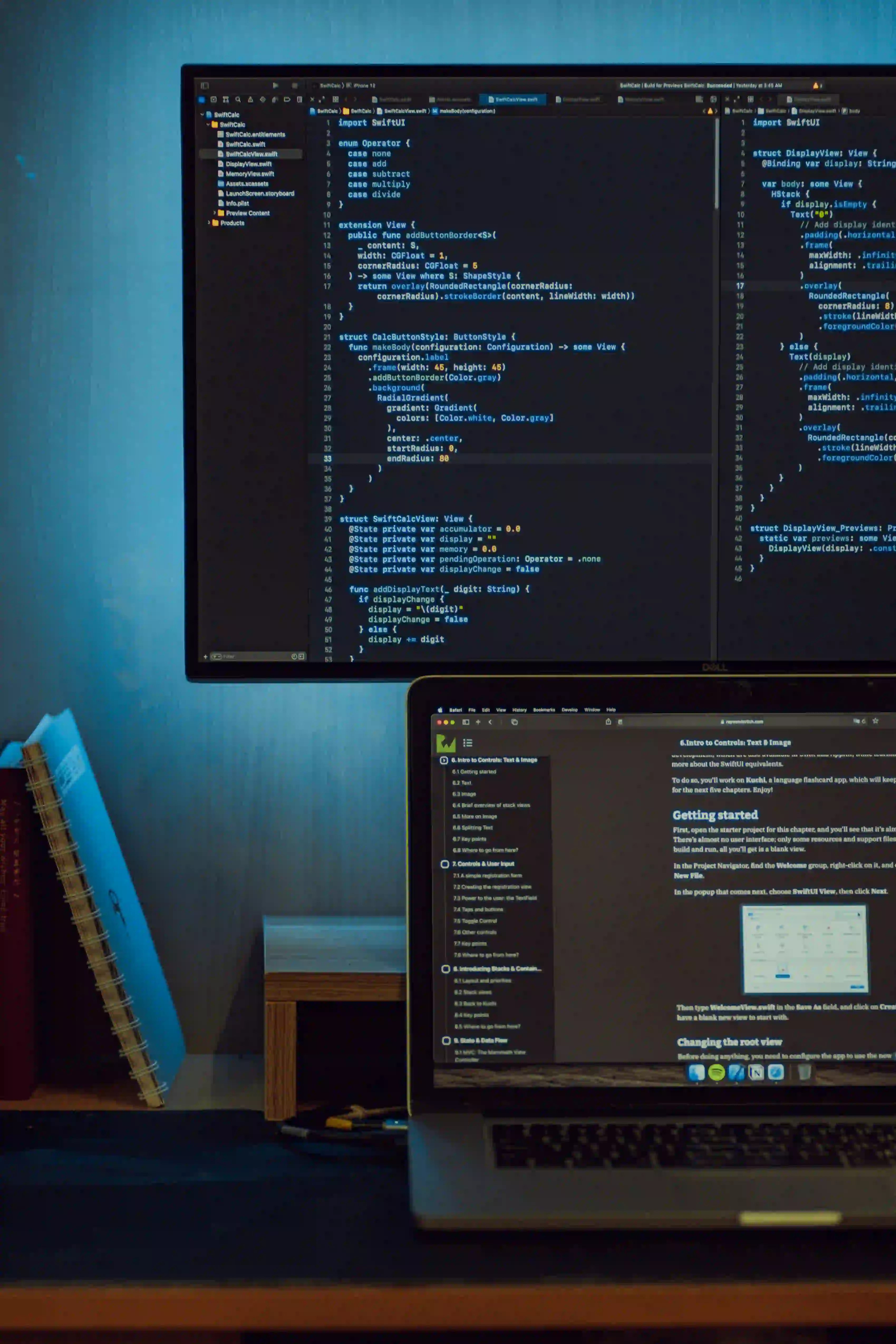
The Challenge of Generating Efficient Source Code
Writing efficient source code is a crucial aspect of software development. Efficient code not only enhances the performance of the application but also minimizes resource consumption. In Java, a popular and versatile programming language, writing efficient code is a multi-faceted challenge that involves considerations such as algorithm optimization, memory management, and proper utilization of language features.
Algorithm Optimization
One of the fundamental aspects of writing efficient source code is algorithm optimization. The choice of algorithms and data structures directly impacts the performance of the application. For instance, when dealing with large datasets, using efficient sorting algorithms like quicksort or mergesort can significantly improve the overall performance compared to less efficient algorithms like bubble sort.
// Example of using Arrays.sort() for efficient sorting
int[] arr = {5, 2, 8, 1, 9};
Arrays.sort(arr);
In the above Java code snippet, using Arrays.sort()
leverages the efficient sorting algorithm implemented in the Java standard library.
Memory Management
Memory management plays a crucial role in writing efficient Java code. Improper memory allocation and deallocation can lead to memory leaks and unnecessary overhead. Utilizing features like object pooling and proper utilization of data types can contribute to efficient memory management.
// Example of object pooling for efficient memory management
class ObjectPool {
private static final int MAX_OBJECTS = 100;
private static List<SomeObject> pool = new ArrayList<>();
public SomeObject getObject() {
if (pool.isEmpty()) {
return new SomeObject();
} else {
return pool.remove(0);
}
}
public void releaseObject(SomeObject obj) {
if (pool.size() < MAX_OBJECTS) {
pool.add(obj);
}
}
}
In the above Java code snippet, the ObjectPool
class demonstrates object pooling to efficiently manage the creation and reuse of objects, thus reducing memory allocation overhead.
Utilization of Language Features
Java offers a broad range of language features that, when used appropriately, can lead to the creation of efficient source code. For example, leveraging Java's multithreading capabilities for parallel processing of tasks can significantly improve the performance of applications that require concurrent execution of operations.
// Example of multithreading for parallel processing
class ParallelProcessor {
public void processInParallel() {
ExecutorService executor = Executors.newFixedThreadPool(4);
for (int i = 0; i < 10; i++) {
Runnable task = new SomeTask(i);
executor.submit(task);
}
executor.shutdown();
}
}
In the above Java code snippet, the ParallelProcessor
class utilizes ExecutorService
and multithreading to process tasks in parallel, effectively utilizing system resources for improved performance.
Bringing It All Together
Writing efficient source code in Java involves a combination of algorithm optimization, memory management, and judicious utilization of language features. By carefully considering these aspects during the development process, developers can create high-performance and resource-efficient applications.
In conclusion, the challenge of generating efficient source code in Java is multi-faceted, requiring a deep understanding of algorithmic complexity, memory management, and effective utilization of language features. By addressing these challenges with precision and care, developers can produce code that not only meets functional requirements but also delivers optimal performance and resource utilization.
For further exploration, you can delve into Java performance optimization techniques using tools such as JMH (Java Microbenchmarking Harness) and profiling tools like VisualVM, which can aid in identifying performance bottlenecks and optimizing Java code for efficiency.
Efficient source code is the cornerstone of high-performance applications, and mastering the art of writing efficient Java code is a rewarding endeavor for any developer.