Microservices: Managing Data Consistency with Couchbase
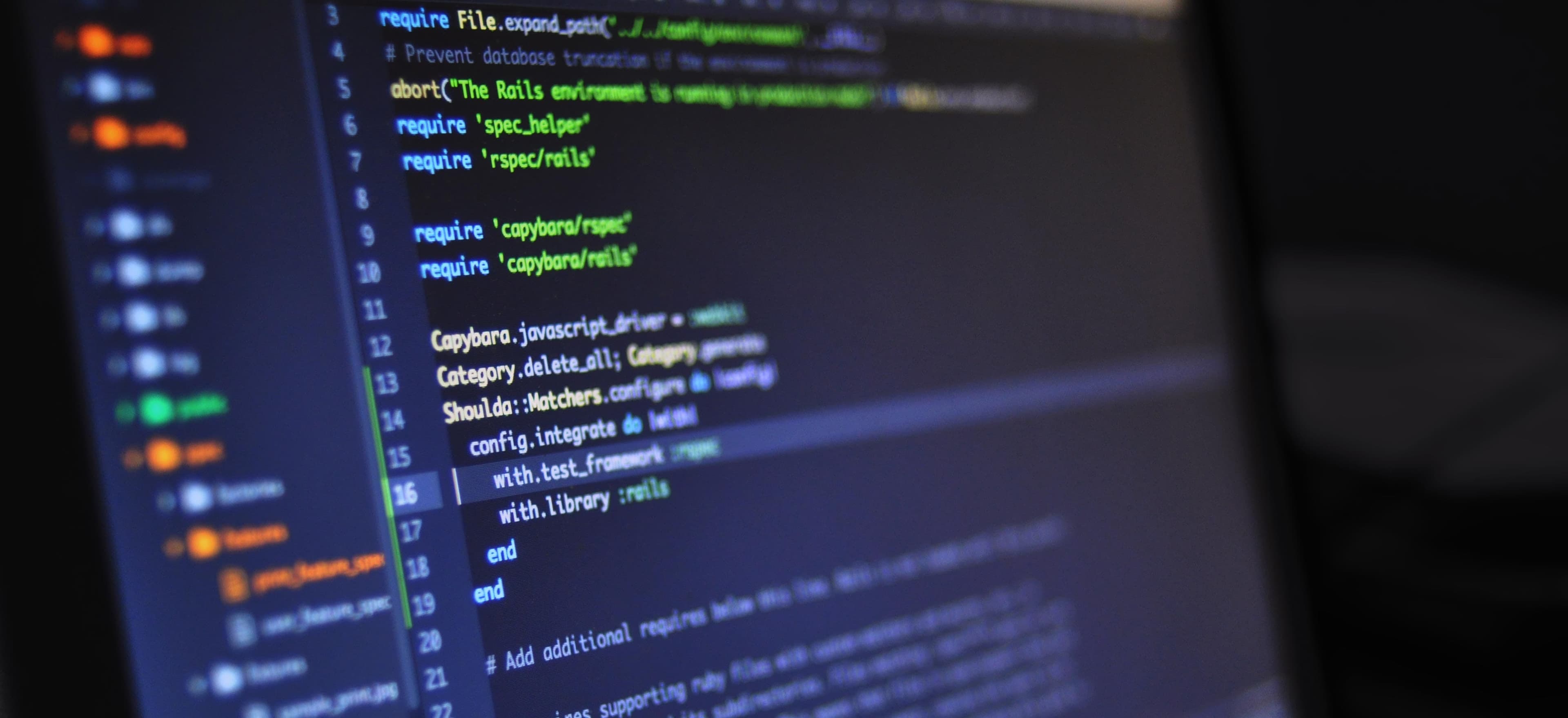
- Published on
Managing Data Consistency in Microservices Architecture with Couchbase
In a microservices architecture, maintaining data consistency across different services can be a significant challenge. Each microservice has its own database, and ensuring that data remains consistent and in sync across all services is crucial. In this blog post, we will explore how Couchbase, a popular NoSQL database, can be used to manage data consistency in a microservices environment.
Understanding the Challenge
In a microservices architecture, each service typically has its own database, which can lead to data inconsistencies when updates are made to related data within different services. For example, consider a scenario where an e-commerce application has separate microservices for managing orders, inventory, and customer information. When a new order is placed, the order service needs to update the inventory and customer information services, ensuring that data remains consistent across all services.
Using Couchbase for Data Consistency
Couchbase is a NoSQL database that provides a flexible data model, distributed architecture, and built-in support for transactions, making it well-suited for managing data consistency in a microservices environment.
JSON Data Model
Couchbase uses a JSON data model, which allows for flexible and schema-less data storage. This flexibility is particularly useful in a microservices architecture where different services may have varying data requirements. With Couchbase, each microservice can store data in a way that makes the most sense for its specific needs, without being constrained by a rigid schema.
// Example of storing JSON data in Couchbase
JsonObject document = JsonObject.create()
.put("id", "123")
.put("name", "John Doe")
.put("email", "john.doe@example.com");
bucket.defaultCollection().upsert("user::123", document);
Distributed Architecture
Couchbase is designed with a distributed architecture, allowing data to be spread across multiple nodes. This makes it well-suited for microservices, as each service can have its own dedicated instance of Couchbase, and data can be distributed and replicated as needed for high availability and scalability.
// Example of configuring Couchbase connection
Cluster cluster = Cluster.connect("localhost", "username", "password");
Bucket bucket = cluster.bucket("my_bucket");
Eventing and Change Data Capture
Couchbase provides eventing and change data capture capabilities, allowing microservices to react to changes in the database. This enables services to stay in sync with each other and maintain data consistency by reacting to data changes in real-time.
// Example of using Couchbase eventing function
function OnOrderCreated(event) {
// Update inventory and customer information
}
Built-in Transactions
Couchbase 7.0 introduces support for distributed ACID transactions, allowing multiple database operations to be performed atomically across different documents and collections. This is particularly beneficial for maintaining data consistency in a microservices environment where complex transactions may span multiple services.
// Example of performing a transaction in Couchbase
cluster.query(
"START TRANSACTION; " +
"UPDATE inventory SET quantity = quantity - 1 WHERE product_id = $product_id; " +
"INSERT INTO order_history (customer_id, product_id) VALUES ($customer_id, $product_id); " +
"COMMIT;"
);
Final Considerations
Managing data consistency in a microservices architecture is crucial for maintaining the integrity of an application's data. Couchbase's flexible data model, distributed architecture, eventing capabilities, and support for transactions make it a compelling choice for addressing the challenges of data consistency in a microservices environment.
By leveraging Couchbase, microservices can ensure that data remains consistent and in sync across different services, ultimately leading to a more robust and reliable application.
To learn more about using Couchbase for microservices, check out the Couchbase documentation for Java SDK and explore the various features that support data consistency and synchronization in a microservices architecture.