Maximizing Efficiency: Spring Hibernate Performance
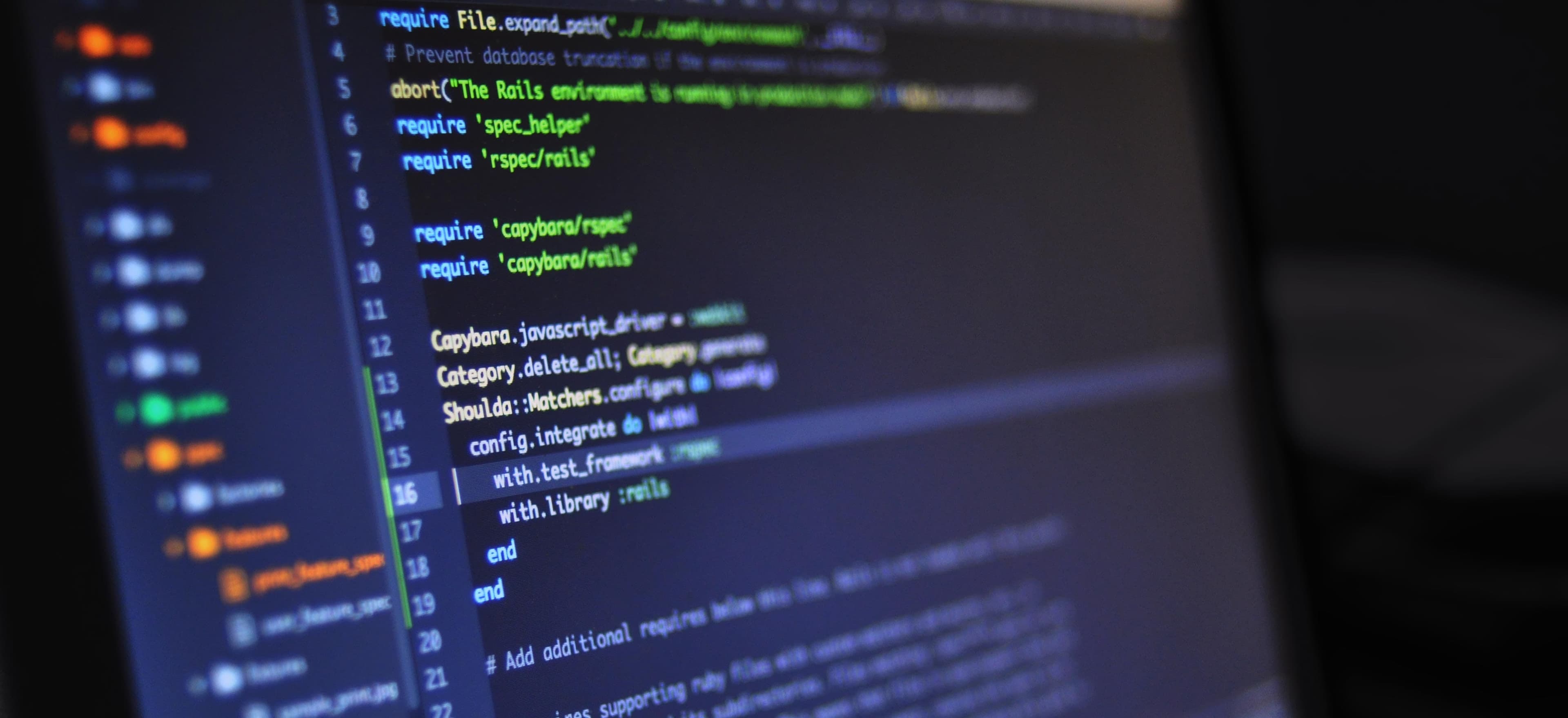
- Published on
Search engine optimization (SEO), when combined with the powerful capabilities of Java, can lead to the optimal performance of web applications. In this blog post, we will delve into the importance of maximizing efficiency through Spring Hibernate performance in Java programming, offering valuable insights and best practices that can significantly enhance the overall user experience.
The emergence of Spring and Hibernate has revolutionized the way Java applications are developed, with both frameworks playing pivotal roles in simplifying the process and improving overall performance. As a Java developer, understanding and optimizing the performance of Spring and Hibernate is crucial for building high-performing, scalable, and responsive applications.
Importance of Performance Optimization in Java
Before delving into Spring Hibernate performance, it is imperative to understand why performance optimization is crucial for Java applications. In the modern digital landscape, user experience and application responsiveness are non-negotiable aspects. Delayed responses, sluggish rendering, or unoptimized database operations can lead to users abandoning the application, impacting user retention, conversion rates, and the overall success of the product.
Leveraging Spring for Optimized Performance
Spring is a powerful framework that provides comprehensive support for building robust Java applications. There are several key strategies and best practices to maximize performance when leveraging Spring in Java development:
-
Dependency Injection:
@Autowired private ExampleService exampleService;
Leveraging dependency injection through annotations like
@Autowired
reduces boilerplate code, simplifies unit testing, and ultimately enhances the modularity of the application. -
Aspect-Oriented Programming (AOP):
@Aspect public class LoggingAspect { @Before("execution(* com.example.service.*.*(..))") public void beforeServiceMethodExecution() { // Log method execution } }
AOP enables cross-cutting concerns such as logging, security, and transaction management, effectively promoting modular application development and improving maintainability.
-
Caching:
@Cacheable("products") public Product getProductById(Long productId) { // Retrieve product from database }
Utilizing Spring's caching annotations can drastically reduce database load and enhance application responsiveness by caching frequently accessed data.
Optimizing Hibernate for Enhanced Performance
Hibernate, as a powerful object-relational mapping (ORM) framework, significantly eases database interactions in Java applications. Here are some optimization strategies to boost Hibernate performance:
-
Entity Mapping:
@Entity @Table(name = "products") public class Product { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; // Other fields and methods }
Efficiently mapping entities to database tables while considering indexes, relationships, and data types is crucial for optimized database interactions.
-
Batch Processing:
session.doWork(connection -> { try (PreparedStatement ps = connection.prepareStatement("...")) { // Execute batch operations } });
Utilizing batch processing for bulk database operations minimizes round trips to the database, reducing overhead and improving performance.
-
Optimized Fetching Strategies:
@OneToMany(mappedBy = "product", fetch = FetchType.LAZY) private List<Order> orders;
Carefully selecting fetching strategies such as lazy loading and eager loading can prevent unnecessary data retrieval, enhancing application responsiveness.
Performance Monitoring and Tuning
Continuous performance monitoring and tuning are critical for maintaining the efficiency of Java applications using Spring and Hibernate. Tools like Java VisualVM, JProfiler, and New Relic provide valuable insights into memory usage, CPU profiling, and database query performance, enabling developers to identify bottlenecks and optimize application performance.
Final Thoughts
Efficiently maximizing Spring Hibernate performance in Java applications is not just a matter of best practices; it is fundamental to the success and competitiveness of modern web applications. By leveraging the optimization strategies discussed in this post, developers can create high-performing, responsive, and scalable applications that deliver exceptional user experiences and achieve business objectives.
In the ever-evolving realm of Java development, staying abreast of the latest trends, best practices, and performance optimization techniques is paramount. Implementing efficient Spring Hibernate performance not only elevates application responsiveness but also solidifies the foundation for building successful, competitive, and user-centric Java applications.
By embracing the power of Spring and Hibernate while upholding a commitment to performance optimization, Java developers can unleash the full potential of their applications, setting new benchmarks for user experience and operational excellence.
Always remember, when it comes to Java programming, optimizing performance is not just a choice; it's a necessity.