Optimizing Object Creation with Factory Method Pattern
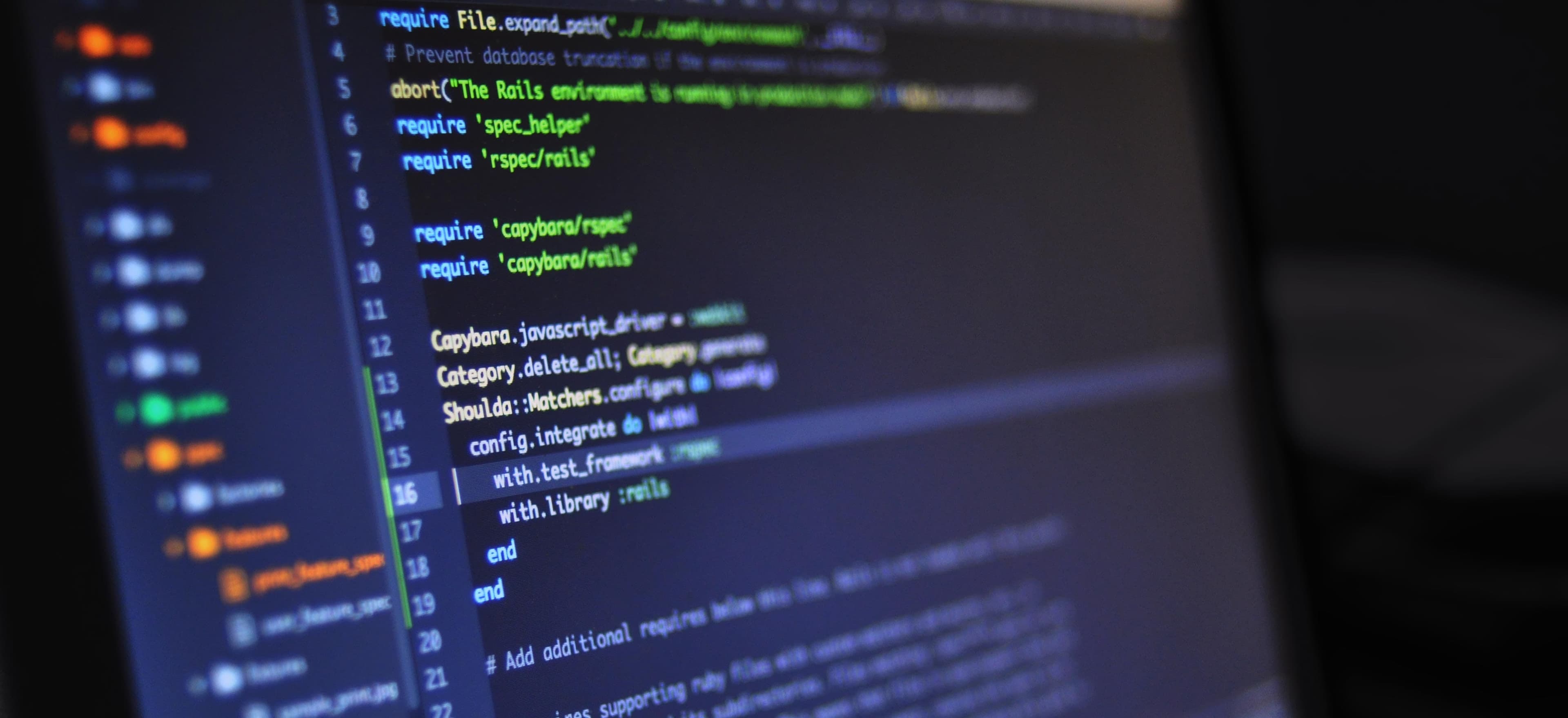
- Published on
Optimizing Object Creation with Factory Method Pattern
When it comes to object creation in Java, there are various design patterns that can help optimize the process. One such pattern is the Factory Method Pattern, which provides a way to delegate the instantiation logic to child classes. This not only promotes loose coupling but also enhances flexibility and maintainability in the codebase.
In this article, we will explore the Factory Method Pattern in Java, discuss its benefits, and provide practical examples to demonstrate its usage.
Understanding the Factory Method Pattern
The Factory Method Pattern falls under the category of creational design patterns and is used to create objects without specifying the exact class of object that will be created. It defines an interface for creating an object, but allows the subclasses to alter the type of objects that will be created.
The key components of the Factory Method Pattern include:
- Product: Defines the interface of objects that the factory method creates.
- Concrete Product: Implements the Product interface.
- Creator: Declares the factory method, which returns an object of type Product.
- Concrete Creator: Overrides the factory method to return an instance of a Concrete Product.
Benefits of Using Factory Method Pattern
Promotes Loose Coupling
The Factory Method Pattern promotes loose coupling by allowing the code to work with an interface rather than concrete implementations. This means that the client code does not need to be aware of the specific class of objects it creates, enhancing flexibility and maintainability.
Enhanced Extensibility
By delegating the object creation to subclasses, the Factory Method Pattern allows for easy extension and addition of new product types without modifying existing client code. This makes the codebase more adaptable to future changes and expansions.
Encourages Consistent Object Creation
The factory method encapsulates the object creation logic, ensuring that all created objects undergo a consistent creation process. This can be especially beneficial when dealing with complex object creation requirements.
Implementation of Factory Method Pattern in Java
Let's dive into a practical example to understand how to implement the Factory Method Pattern in Java. Suppose we have a scenario where we need to create different types of shapes such as circles and squares.
Product Interface: Shape
public interface Shape {
void draw();
}
Concrete Products: Circle and Square
public class Circle implements Shape {
@Override
public void draw() {
System.out.println("Drawing Circle");
}
}
public class Square implements Shape {
@Override
public void draw() {
System.out.println("Drawing Square");
}
}
Creator Interface: ShapeFactory
public interface ShapeFactory {
Shape createShape();
}
Concrete Creators: CircleFactory and SquareFactory
public class CircleFactory implements ShapeFactory {
@Override
public Shape createShape() {
return new Circle();
}
}
public class SquareFactory implements ShapeFactory {
@Override
public Shape createShape() {
return new Square();
}
}
Usage:
public class Main {
public static void main(String[] args) {
ShapeFactory circleFactory = new CircleFactory();
Shape circle = circleFactory.createShape();
circle.draw();
ShapeFactory squareFactory = new SquareFactory();
Shape square = squareFactory.createShape();
square.draw();
}
}
In the example above, the Shape
interface represents the product, while Circle
and Square
represent the concrete products. The ShapeFactory
interface declares the factory method, and CircleFactory
and SquareFactory
serve as concrete creators that override the factory method to create instances of the respective shapes.
When the Main
class uses the factory methods to create shapes, it does so without having to know the specific implementation details of the shapes, thus achieving loose coupling and flexibility.
Closing the Chapter
In conclusion, the Factory Method Pattern in Java offers an elegant way to delegate object creation responsibilities to subclasses, promoting loose coupling, enhancing extensibility, and ensuring consistent object creation. By understanding and implementing this pattern, developers can improve the maintainability and flexibility of their codebase, making it easier to manage changes and additions in the future.
Incorporating the Factory Method Pattern in appropriate scenarios can lead to cleaner, more modular, and adaptable code, ultimately contributing to the overall efficiency and effectiveness of Java applications.
By leveraging design patterns like the Factory Method Pattern, developers can continuously refine and optimize their code, driving the advancement of software development practices and contributing to the evolution of the Java ecosystem.
So why not start implementing the Factory Method Pattern in your Java projects and experience the benefits firsthand? Remember, promoting loose coupling and enhancing extensibility are key factors in designing robust and scalable Java applications.
In conclusion, the Factory Method Pattern is a valuable tool in the design patterns arsenal for Java developers, offering a powerful way to optimize object creation and elevate the quality of software systems. Embracing this pattern can lead to more modular, maintainable, and future-proof codebases, establishing a solid foundation for the development of robust Java applications. So, don't hesitate to integrate the Factory Method Pattern into your Java projects and witness the positive impact it can bring to your software architecture and development workflow.