Improving Data Transfer Performance for REST Messages
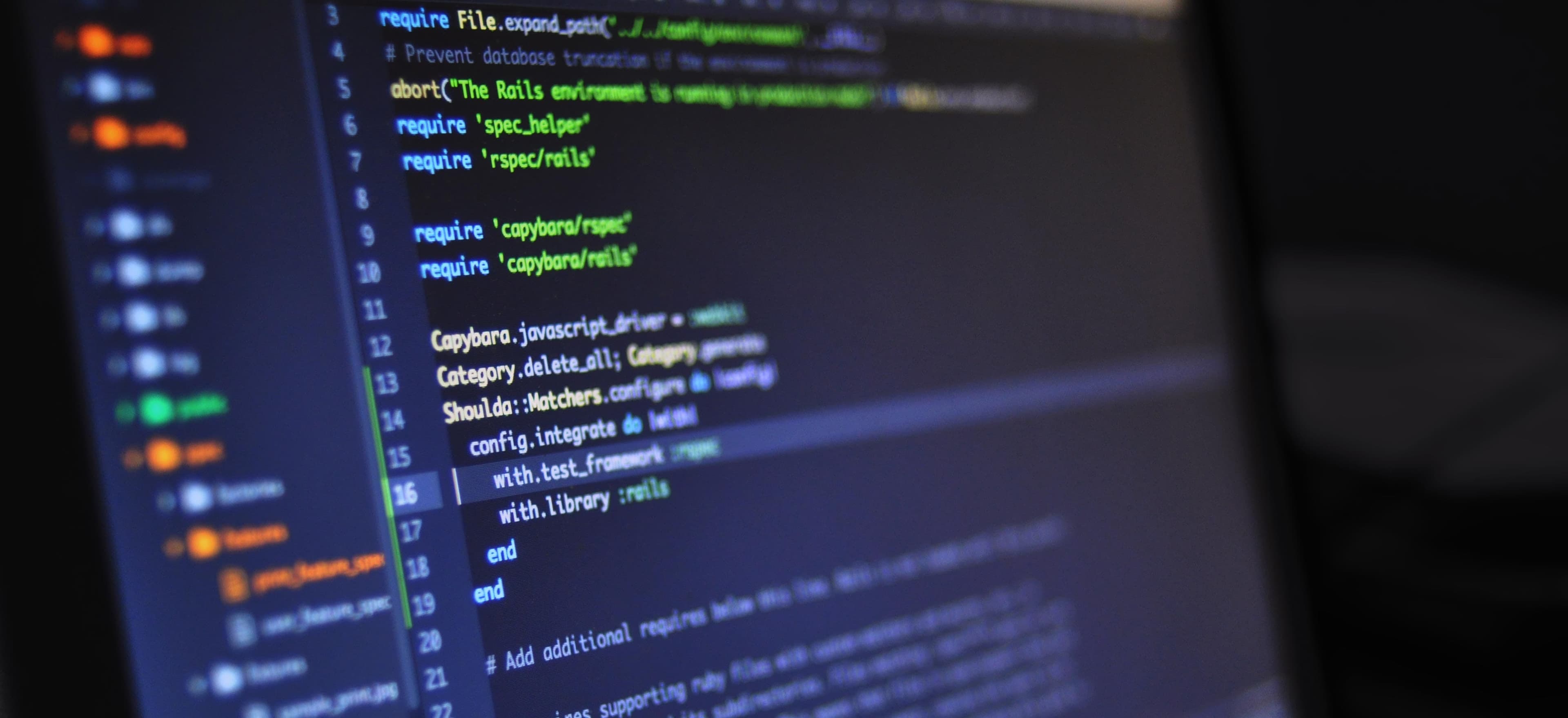
- Published on
Improving Data Transfer Performance for REST Messages
When working with REST APIs, one of the critical factors to consider is how to optimize data transfer performance. Efficient data transfer not only improves the user experience but also reduces server load and operational costs. In this article, we'll explore several strategies for improving data transfer performance for REST messages in Java.
1. Use GZIP Compression
One of the most effective ways to improve data transfer performance is by using GZIP compression. GZIP is a popular data compression algorithm that can significantly reduce the size of HTTP responses, leading to faster data transmission.
In Java, you can enable GZIP compression for REST messages by using libraries like java.util.zip
or javax.ws.rs.ext.WriterInterceptor
. By compressing the response data before sending it over the network, you can reduce the amount of data that needs to be transferred, thereby improving performance.
Example of GZIP Compression in Java
@Provider
@Compress
public class GZIPWriterInterceptor implements WriterInterceptor {
@Override
public void aroundWriteTo(WriterInterceptorContext context)
throws IOException, WebApplicationException {
MultivaluedMap<String, Object> headers = context.getHeaders();
headers.add("Content-Encoding", "gzip");
context.setOutputStream(new GZIPOutputStream(context.getOutputStream()));
context.proceed();
}
}
In this example, the GZIPWriterInterceptor
class uses WriterInterceptor
to intercept the outgoing response and apply GZIP compression to the data.
2. Leverage HTTP/2
HTTP/2 is a major revision of the HTTP network protocol and offers significant performance improvements over its predecessor, HTTP/1.1. One of the key features of HTTP/2 is its ability to multiplex multiple requests over a single TCP connection, avoiding the overhead of opening multiple connections for parallel data transfer.
In Java, you can leverage HTTP/2 for REST message transfer by using libraries like Jetty or Netty, which provide support for HTTP/2. By upgrading your server and client to use HTTP/2, you can take advantage of its performance benefits and improve data transfer speeds for REST messages.
3. Implement Caching
Another strategy to improve data transfer performance is to implement caching for REST messages. Caching can help reduce the amount of data that needs to be transferred by storing and reusing previously retrieved data.
In Java, you can implement caching for REST messages using libraries like Ehcache or Caffeine. By caching frequently accessed data at the client or server side, you can reduce the number of requests that need to be made over the network, leading to faster data transfer and improved performance.
4. Use Asynchronous Processing
Asynchronous processing is another effective technique for improving data transfer performance in REST APIs. By using asynchronous programming models like CompletableFuture or reactive programming with libraries like Reactor or RxJava, you can process and transfer data in a non-blocking fashion, allowing for better resource utilization and improved throughput.
In Java, you can implement asynchronous processing for REST messages by using frameworks like Spring WebFlux or asynchronous servlets in Servlet 3.0. By handling incoming requests asynchronously and using non-blocking I/O operations, you can improve the responsiveness and performance of your REST API.
Closing the Chapter
In conclusion, optimizing data transfer performance for REST messages in Java involves the use of various techniques such as GZIP compression, leveraging HTTP/2, implementing caching, and using asynchronous processing. By employing these strategies, you can improve the overall performance of your REST APIs, leading to faster data transfer, better user experience, and reduced server load.
Optimizing data transfer performance is essential for ensuring that your REST APIs are able to handle a high volume of requests efficiently. By incorporating the discussed strategies into your Java-based REST APIs, you can enhance their performance and provide a better experience for your users.
Remember, the goal is not only to write code but to write efficient and performant code. Consider how your design and implementation decisions will impact the data transfer performance and make adjustments as necessary. Happy coding!
The article aimed to provide a comprehensive overview of strategies for improving data transfer performance for REST messages in Java. By implementing the suggested techniques, developers can enhance the efficiency and speed of their REST APIs, ultimately leading to a better user experience. If you're interested in exploring further details about REST API development, check out Oracle's RESTful Web Services guide.