Troubleshooting Alternating Between Spray Servlet and Spray Can
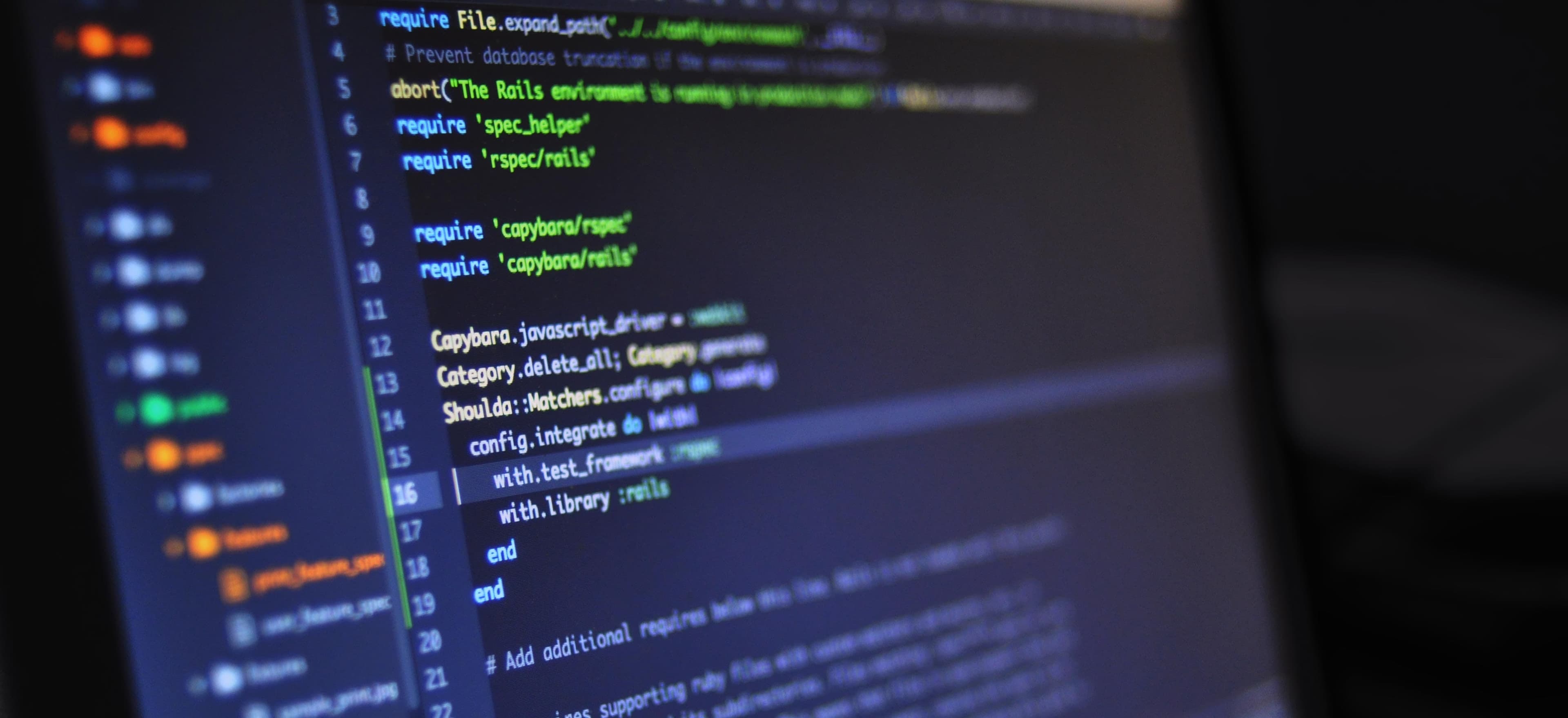
- Published on
Troubleshooting Alternating Between Spray Servlet and Spray Can
In this blog post, we will discuss some common issues and solutions when working with Spray Servlet and Spray Can in a Java application. Spray is a lightweight, high-performance framework for building RESTful web services, and understanding how to troubleshoot common issues can save time and frustration during development.
Issue 1: Handling Request Timeouts
One common issue when working with Spray Servlet and Spray Can is handling request timeouts. When a client sends a request to the server, it expects a timely response. If the server takes too long to process the request, the client may time out and close the connection.
To address this issue, it's important to configure the timeout settings for both the server and client components. In Spray, timeout settings can be adjusted using the spray.can.server.request-timeout
and spray.can.client.idle-timeout
configuration properties.
// Example configuration for server request timeout
spray.can.server.request-timeout = 5s
// Example configuration for client idle timeout
spray.can.client.idle-timeout = 10s
By configuring these timeout settings appropriately, you can ensure that requests are handled within a reasonable timeframe, reducing the likelihood of timeouts occurring.
For more detailed information on configuring timeout settings in Spray, refer to the official Spray documentation.
Issue 2: Resource Exhaustion
Another common issue that can arise when using Spray Servlet and Spray Can is resource exhaustion. This can occur when the server handles a large number of concurrent requests, leading to high CPU and memory usage.
To mitigate resource exhaustion, consider implementing techniques such as connection pool management, load balancing, and optimizing request handling logic. For example, using connection pools can help efficiently manage resources and prevent excessive consumption.
// Example of connection pool configuration
val poolClient = system.actorOf(ConnectionPoolActor.props(httpClient, poolSettings), "pool-client")
Additionally, optimizing request handling logic, such as reducing unnecessary computations and minimizing memory footprint, can contribute to better resource management.
Issue 3: Serialization and Deserialization Errors
Serialization and deserialization errors can also be a source of frustration when working with Spray Servlet and Spray Can. These errors commonly occur when data is not properly serialized or deserialized during communication between the client and server.
To address serialization and deserialization errors, it's crucial to ensure that the data models and protocols are accurately defined and implemented on both the client and server sides. Additionally, using standardized data formats such as JSON or Protocol Buffers can help mitigate compatibility issues and improve data transfer reliability.
// Example of defining a data model using JSON format
case class User(id: Int, name: String)
implicit val userFormat = jsonFormat2(User)
By carefully defining and implementing data models and utilizing standardized data formats, you can minimize the risk of serialization and deserialization errors.
The Closing Argument
In this blog post, we discussed common issues and solutions when working with Spray Servlet and Spray Can in a Java application. By addressing issues related to request timeouts, resource exhaustion, and serialization/deserialization errors, you can enhance the performance and reliability of your RESTful web services built with Spray.
When encountering issues with Spray Servlet and Spray Can, it's essential to approach troubleshooting systematically, considering various aspects such as timeout settings, resource management, and data transfer protocols. Being proactive in addressing these issues can lead to more robust and resilient web services, ultimately improving the overall user experience.
I hope this blog post has been helpful in shedding light on common troubleshooting scenarios when working with Spray Servlet and Spray Can in Java applications. If you have any additional tips or experiences related to troubleshooting with Spray, feel free to share them in the comments below. Thank you for reading!