Troubleshooting Common Issues in Importing Data
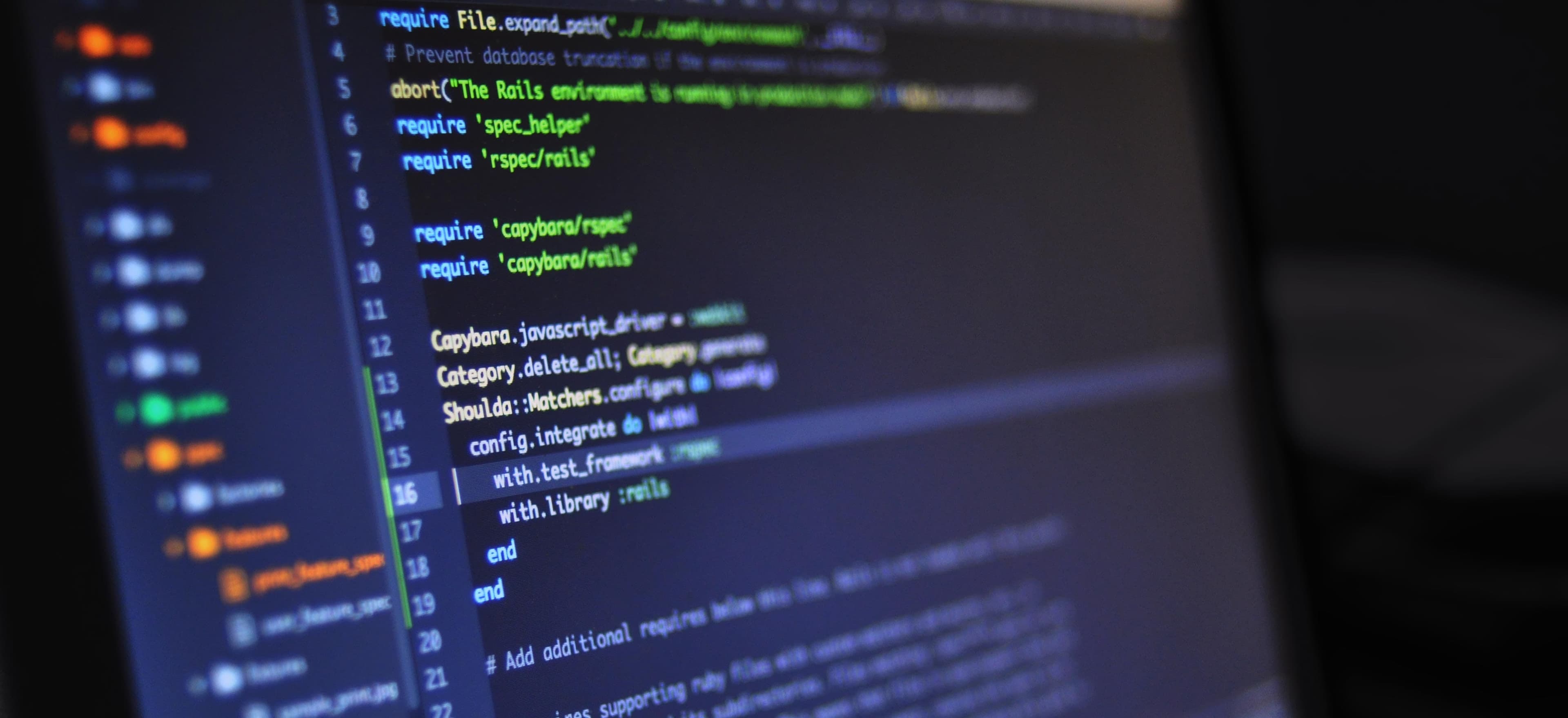
- Published on
Troubleshooting Common Issues in Importing Data
Importing data is a common task in Java development, but it can come with its fair share of challenges. Whether you're reading from a file, a database, or an API, there are several potential stumbling blocks that can hinder the process. In this article, we'll explore some common issues that developers encounter when importing data in Java and discuss how to troubleshoot and resolve them.
Issue 1: File Not Found
One of the most common issues when importing data from a file is encountering a "File Not Found" exception. This typically occurs when the specified file path is incorrect or when the file does not exist at the provided location.
Troubleshooting Steps:
- Ensure that the file path is correct and that the file exists at the specified location.
- Use the
Paths
andFiles
classes from thejava.nio.file
package to handle file operations robustly.
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.io.IOException;
public class FileImportExample {
public static void main(String[] args) {
Path filePath = Paths.get("path/to/your/file.txt");
try {
String content = Files.readString(filePath);
System.out.println(content);
} catch (IOException e) {
System.err.println("Error reading file: " + e.getMessage());
}
}
}
In the code snippet above, we use the Paths.get
method to create a Path
object representing the file, and then use Files.readString
to read the file content. By using these classes, we handle file operations more robustly and can catch potential exceptions that may occur.
Issue 2: Incorrect Data Format
Another common issue is dealing with incorrect data formats when importing data from external sources such as APIs or databases. This can lead to parsing errors, data type mismatches, and other related issues.
Troubleshooting Steps:
- Validate the incoming data against the expected format and structure.
- Utilize libraries like Gson or Jackson for parsing JSON data from APIs, and handle the mapping of JSON to Java objects seamlessly.
import com.google.gson.Gson;
public class JsonImportExample {
public static void main(String[] args) {
String json = "{\"name\":\"John\",\"age\":30,\"city\":\"New York\"}";
Gson gson = new Gson();
Person person = gson.fromJson(json, Person.class);
System.out.println(person.getName());
}
}
class Person {
private String name;
private int age;
private String city;
// Getters and setters
}
In the code above, we use the Gson library to parse JSON data into a Java object. By utilizing libraries like Gson or Jackson, we can efficiently handle JSON parsing and mapping, thereby mitigating potential data format issues.
Issue 3: Connection Errors
When importing data from external sources like databases or APIs, connection errors are a common stumbling block. These errors can occur due to network issues, incorrect credentials, or issues with the external service.
Troubleshooting Steps:
- Double-check the network connectivity and ensure that the required ports are open.
- Verify the credentials being used for authentication with the external source.
- Use proper error handling techniques, such as try-catch blocks, to capture and handle connection-related exceptions gracefully.
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DatabaseConnectionExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/mydatabase";
String username = "username";
String password = "password";
try {
Connection connection = DriverManager.getConnection(url, username, password);
// Proceed with data import
} catch (SQLException e) {
System.err.println("Error connecting to database: " + e.getMessage());
}
}
}
In the above example, we establish a database connection using JDBC. By utilizing proper error handling techniques, such as the try-catch block, we can capture and handle connection-related exceptions gracefully, providing insightful error messages for debugging.
Issue 4: Performance Bottlenecks
Large-scale data imports can sometimes lead to performance bottlenecks, especially when dealing with massive datasets or inefficient data processing algorithms.
Troubleshooting Steps:
- Implement pagination and batch processing techniques when dealing with large datasets to avoid memory issues and enhance performance.
- Utilize appropriate data structures and algorithms to efficiently process and manipulate the imported data.
import java.util.List;
public class DataImportPerformanceExample {
public static void main(String[] args) {
int offset = 0;
int limit = 100;
List<Data> batch = fetchDataBatch(offset, limit);
while (!batch.isEmpty()) {
processBatch(batch);
offset += limit;
batch = fetchDataBatch(offset, limit);
}
}
private static List<Data> fetchDataBatch(int offset, int limit) {
// Database or API call to fetch data batch
// Return fetched batch as a List
return /* Fetched data */;
}
private static void processBatch(List<Data> batch) {
// Process and manipulate the data batch
}
}
In the example above, we demonstrate implementing pagination and batch processing when importing large datasets. By fetching and processing data in manageable batches, we can significantly enhance performance and mitigate potential memory-related issues.
The Bottom Line
Importing data in Java can present various challenges, ranging from file-related issues to performance bottlenecks. By following the troubleshooting steps outlined in this article and leveraging appropriate libraries and techniques, developers can effectively address and resolve common issues encountered during the data import process. Additionally, fostering a robust understanding of Java's I/O operations, data parsing libraries, connection handling, and performance optimization is essential for successful data import operations in Java.
By tackling these common issues head-on and enhancing your expertise in Java data import techniques, you can ensure a smoother and more efficient data import process, laying a strong foundation for robust and reliable Java applications.
Remember, encountering challenges during data import is inevitable, but with the right approach and expertise, you can effectively navigate through them, ensuring seamless data import operations in your Java projects.
Keep exploring and mastering Java data import techniques to elevate your development skills and create impactful software solutions.
Happy coding!