Choosing the Right Request-Response Pattern in Akka
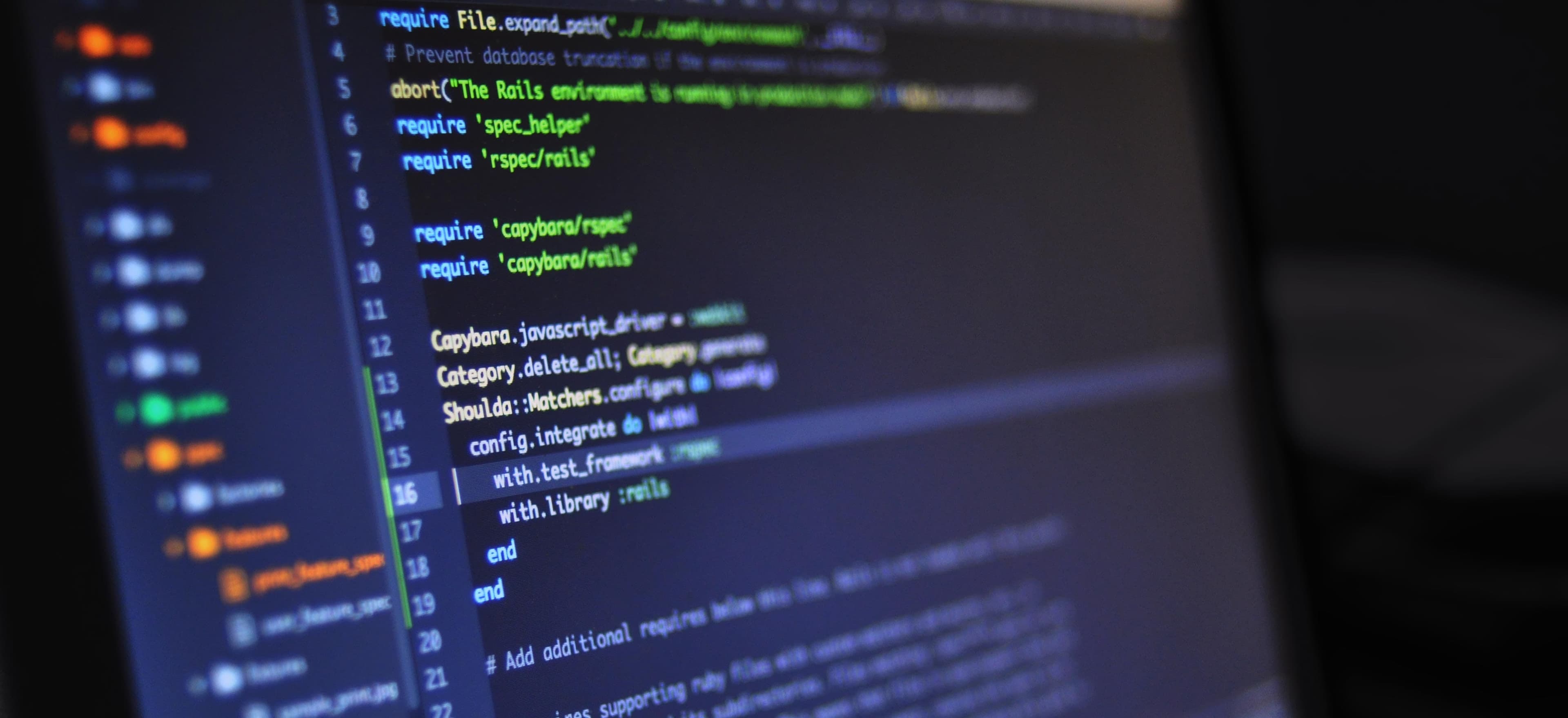
- Published on
Choosing the Right Request-Response Pattern in Akka
When developing applications in Akka, a popular toolkit for building concurrent, distributed, and resilient message-driven systems, it's essential to choose the right request-response pattern to ensure efficient communication between actors. In this article, we'll explore various request-response patterns in Akka and discuss when to use each one.
Understanding Akka Actors
In Akka, actors are the building blocks of concurrent and distributed applications. They communicate with each other by sending and receiving messages. When one actor needs to request information or a service from another actor, it sends a message and expects a response. Choosing the appropriate request-response pattern can significantly impact the performance and design of your Akka application.
The Ask Pattern
The most common way to achieve request-response communication in Akka is through the ask
pattern, which is also known as the request-reply pattern. In this pattern, an actor sends a message to another actor using the ?
operator, expecting a response in the form of a Future
. The sender actor then can map
over the Future
to process the response when it arrives.
import akka.actor.ActorRef;
import akka.pattern.Patterns;
import akka.util.Timeout;
import scala.concurrent.Future;
import java.time.Duration;
import java.util.concurrent.CompletionStage;
public class RequesterActor {
private final ActorRef responderActor;
private final Timeout timeout = Timeout.create(Duration.ofSeconds(5));
public RequesterActor(ActorRef responderActor) {
this.responderActor = responderActor;
}
public CompletionStage<Object> makeRequest() {
return Patterns.ask(responderActor, "Hello", timeout);
}
}
The ask
pattern is suitable when the sender actor needs to wait for a response and perform further processing based on the result. However, it's essential to consider the potential pitfalls of using the ask
pattern, such as potential timeouts and increased overhead due to the creation of Future
wrappers.
The Tell-Only Pattern
In some cases, the sender actor may not require a response from the receiver actor. In such scenarios, it's more efficient to use the tell-only pattern, wherein the sender actor simply sends a message to the receiver actor without expecting a response. This pattern is useful for fire-and-forget scenarios, where the sender actor delegates a task to the receiver actor and continues with its own processing without waiting for a response.
public class SenderActor {
private final ActorRef receiverActor;
public SenderActor(ActorRef receiverActor) {
this.receiverActor = receiverActor;
}
public void sendRequest() {
receiverActor.tell("Execute", ActorRef.noSender());
}
}
The tell-only pattern is suitable for scenarios where the sender actor is not interested in the response and wants to achieve loose coupling between actors. It helps in creating a more asynchronous and decoupled system by allowing actors to independently handle their tasks without waiting for each other.
The Pipe Pattern
Another request-response pattern in Akka is the pipe pattern, which is useful when you want to forward the response from one actor to another while preserving the original sender of the request. This pattern is beneficial when you need to transform or enrich the response before passing it to the final recipient.
import akka.pattern.Patterns;
import scala.concurrent.Future;
public class IntermediateActor {
private final ActorRef finalRecipientActor;
public IntermediateActor(ActorRef finalRecipientActor) {
this.finalRecipientActor = finalRecipientActor;
}
public void forwardRequestResponse(Future<Object> response) {
Patterns.pipe(response, getContext().dispatcher()).to(finalRecipientActor);
}
}
The pipe pattern allows you to maintain the original sender context, which can be crucial for tracking the flow of requests and responses in a distributed system. It's particularly useful when you need to aggregate or modify the response before passing it downstream.
Choosing the Right Pattern
When deciding which request-response pattern to use in Akka, it's essential to consider the specific requirements and constraints of your application. Here are some key considerations to help you make an informed decision:
-
Response Expectation: Determine whether the sender actor needs to receive a response from the receiver actor. If a response is not required, consider using the tell-only pattern for better performance and loose coupling.
-
Asynchronous Processing: If the sender actor can continue its processing without waiting for a response, the tell-only pattern or the pipe pattern may be more suitable, as they allow for asynchronous communication between actors.
-
Error Handling: Consider how each pattern handles errors and timeouts. The
ask
pattern, for example, requires careful consideration of potential timeouts and error handling for the associatedFuture
. -
Message Transformation: If you need to transform or enrich the response before forwarding it to another actor, the pipe pattern provides a convenient way to achieve this while preserving the original sender context.
The Last Word
In conclusion, choosing the right request-response pattern in Akka is crucial for building efficient and resilient message-driven systems. By understanding the trade-offs and characteristics of the ask pattern, tell-only pattern, and pipe pattern, you can make informed decisions when designing the communication flow between actors in your Akka applications. It's essential to consider the specific requirements of your application and the desired behavior of the involved actors to select the most suitable pattern for each interaction.
In the world of Akka, where actors collaborate through message passing, the choice of request-response pattern can make a significant impact on the performance and behavior of the system. By considering the response expectation, asynchronous processing needs, error handling, and message transformation requirements, you can determine the most appropriate pattern for your Akka actors to communicate effectively and efficiently.
For more in-depth insights into Akka and its powerful features, refer to the official Akka documentation. Additionally, exploring real-world use cases and best practices can further enhance your understanding of selecting the right request-response pattern for your Akka applications.